ReactJS input type url form validation Regular expression example
In this tutorial, you will learn how to do input type url form validation in the reactjs application.
You can see the full code on Github🔗
Other versions available:
We will create react application using the create-react-app tool to run the application.
npx create-react-app react-input-form
Now, let’s run the application
cd react-input-form
npm run start
It starts the application and opens localhost:3000
Input type url regular expression validation in reactjs
This example explains valid website URLs on change event handlers.
Here are steps for adding validation
Create a component folder in src folder
Lets create a Form a component named as
InputUrlComponent
Add form element inside a render function
Create
<input>
element withtype=url
insideform
Create a button to handle submit functionality
Attach
onChange
bind event to input elementCreate a two variables
websiteurl
for holding input url, isValid value to check validation succesful or not.Create a state object and initialize default values for
websiteurl
and isValid=false inside a constructor of a form component as seen belowconstructor(props) { super(props); this.state = { websiteUrl: "", isValid: false } }
form input
value
is bounded to the state values as seen below
<input type="text" name="websiteUrl" value={websiteUrl}
- we need to handle the user entered data on the input field, Otherwise, typed data is not shown, So added the
onChange
event handler with the `changeUrl’ function
onChange={this.changeUrl}
OnChange handler in react
being called while user typing the text. This will call changeUrl function defined as follows
changeUrl = (e) => {
const { value } = e.target;
const isValid = !value || this.validateWebsiteUrl(value);
this.setState({
websiteUrl: value,
isValid,
});
};
This saves the entered url value and checks validation logic, stores the validation result as well as entered url.
- Let’s define the validation logic for url
validateWebsiteUrl = (websiteUrl) => {
const urlRegEx =
"[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)?";
return urlRegEx.test(String(websiteUrl).toLowerCase());
};
Defined regular expression pattern for URL
This will check websiteUrl against regular expression using the test
method return true, if url is valid, else false
- Displayed error text in red color if isValid=false
- Disabled if entered URL is invalid
Complete code for handing input type form validation
import React, { Component } from "react";
class InputUrlComponent extends Component {
state = {
websiteUrl: "",
isValid: false,
};
validateWebsiteUrl = (websiteUrl) => {
const urlRegEx =
"[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)?";
return urlRegEx.test(String(websiteUrl).toLowerCase());
};
changeUrl = (e) => {
const { value } = e.target;
const isValid = !value || this.validateWebsiteUrl(value);
this.setState({
websiteUrl: value,
isValid,
});
};
submitForm = () => {
const { websiteUrl } = this.state;
console.log("Website URL", websiteUrl);
};
render() {
const { isValid, websiteUrl } = this.state;
return (
<div className="App">
<form>
<input
type="text"
name="websiteUrl"
value={websiteUrl}
onChange={this.changeUrl}
/>
{!this.state.isValid && (
<div style={{ color: "red" }}>URL is invalid</div>
)}
<br></br>
<button onClick={this.submitForm} disabled={!isValid}>
Submit
</button>
</form>
</div>
);
}
}
export default InputUrlComponent;
InputUrlcomponent is called in App.js
, here is the file.
import "./App.css";
import InputUrl from "./components/Input-url";
function App() {
return (
<div className="App">
<h1>React Input type url pattern validation example</h1>
<InputUrl />
</div>
);
}
export default App;
Here is the output you have seen on the screen.
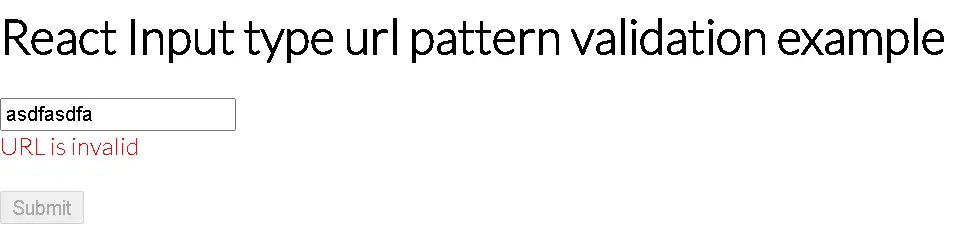