Vuejs Input type url form Validation example
This short tutorial covers step by step tutorials to validate input form type=url in vuejs. URL validation can be done using a regular expression pattern.
How to add number validation to a form in VueJS
You can see the full code on Github🔗
Other versions available:
VueJS new application creation
Here is a sequence of steps to create an application
First, Create a Vue application using the
vuecli
toolMake sure that the Vue CLI npm command is installed already by issuing the following command
B:\blog\jswork>vue --version @vue/cli 4.5.9
It gives the version number of the installed tool if it is not installed, run the below command in the terminal.
npm install -g @vue/cli
It will install Vue CLI into your machine.
Next, create a new application
vue create command
as followsvue create vue-input-url -n
It creates a vuejs application - with files, dependencies, and build pipelines configured and ready to start the application
Go to the application root directory, Run an application using the
npm run serve
commandcd vue-input-url npm run serve
It will start the application and open localhost:3000 in a browser.
Input type URL pattern validation
Input forms are basic forms in the UI pages to take input from the user.
Following are step-by-step guides to add validation to the input form using a regular expression pattern
.
Create a
vuejs component
InputUrlComponent under thesrc/components
folderVueJS component contains HTML, javascript, and styles under the following tags.
<template> // html template code </template> <script> // javascript code </script> <style> // css styles </style>
HTML template contains UI elements logic ie view layer to display to the user. It looks at how the user sees the page. javascript code contains controller logic on how data is passed to/from the view layer. CSS styles - contains styles UI elements
Created input elements to view the layer
<input type="url" placeholder="Website url" v-model="email" @input="change($event)" @change="change($event)" />
An input text box is displayed with type=url only
v-model
is a vuejs directive to have a two-way data binding@input
and@change
are two change handlers while the user types the text.in scripts of the Vue component, Add the following things
Created three instance members url - to hold the form input value isValid- store the validation errors of an input type data regex: is a regular expression to test url validation
Regular expression pattern for URL form The same regular expression that can be used for
javascript
applications alsoregex=[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)?
In the
methods
section, Create two methods, change - to handle change event bounded to@change event
in the template.isUrlValid
function- checks regular expressionchange:function(e){ const url = e.target.value this.isURLValid(url); }, isURLValid: function(inputUrl) { this.isValid= this.reg.test(inputUrl) }
Finally, Display the error using the
v-if
directive attribute<div class="error" v-if="!isValid">URL is Invalid</div
Here is the complete source code for InputUrlComponent
<template>
<div class="hello">
<h3>Vuejs Input type url validation</h3>
<br />
<input type="url" placeholder="Website url" v-model="email" @input="change($event)"
@change="change($event)"/>
<div class="error" v-if="!isValid">URL is Invalid</div
</div>
</template>
<script>
export default {
name: "InputUrl",
data() {
return {
url: "",
isValid: false,
regex: [-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)?
};
},
methods: {
change:function(e){
const url = e.target.value
this.isURLValid(url);
},
isURLValid: function(inputUrl) {
this.isValid= this.regex.test(inputUrl)
}
}
};
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h3 {
margin: 40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
.error{
color:red;
}
</style>
user can see the output in the browser as follows
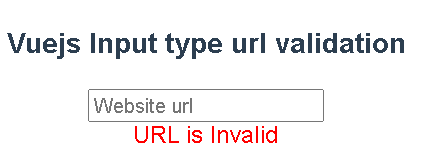
conclusion
This is simple input form validation for type=url, Even though we can write custom validation for complex forms, however, Node provides different frameworks veevalidate
and joi
libraries for simplifying it.