Angular 15 URL validation example |How to check whether URL is invalid or not?
Learn how to do URL validation in Angular and typescript applications with input form.
This tutorial works on all angular 12 and 13 versions.
Other versions available:
Url pattern validation can be implemented with multiple approaches
Angular URL validation with Reactive Forms
First, in the application, an HTML template component creates to display the input form and do the following steps.
- Define the form element with
FormGroup directive
with urlForm binding - Inside the form, define the input element with the
formControlName
name URL - validation errors are bound and available with
urlForm.controls[url]
- Validation check added for input box is not empty(errors.required) and focus is in it(touched)
- if bound errors contains required, display the error message ‘URL is required’
- if errors contain the pattern, display the error message ‘URL format is wrong`.
<div>
<h2>{{ heading | titlecase }}</h2>
<form [formGroup]="urlForm">
<input type="text" formControlName="url" />
<div
*ngIf="!urlForm.controls['url'].valid && urlForm.controls['url'].touched"
>
<div
*ngIf="urlForm.controls['url'].errors.required"
style="background-color: red"
>
URL is required
</div>
<div
*ngIf="urlForm.controls['url'].errors.pattern"
style="background-color: red"
>
URL format is wrong
</div>
</div>
</form>
<button type="submit ">Submit</button>
</div>
Next, In the application controller component, define the logic for regex pattern validation.
- Declared urlForm of type
FormGroup
which already has a binding to form element. - Defined the
URL regular expression pattern
into variable - In the constructor, initialized urlForm with a new FormControl element ie URL
- these URL form control will be bound with input elements in the HTML template
Validators.required
added for not empty validation checkValidators.pattern
added for URL regular expression- These validations are checked with
blur
event `updateOn: blur’ property
import { Component } from '@angular/core';
import { FormControl, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
heading = 'angular url pattern validation with examples;
urlForm: FormGroup;
myusername: string = '';
urlRegEx =
'[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)?';
constructor() {
this.urlForm = new FormGroup({
url: new FormControl('', {
validators: [Validators.required, Validators.pattern(this.urlRegEx)],
updateOn: 'blur',
}),
});
}
submit() {
console.log(this.urlForm.value);
}
}
And output you see in the browser is
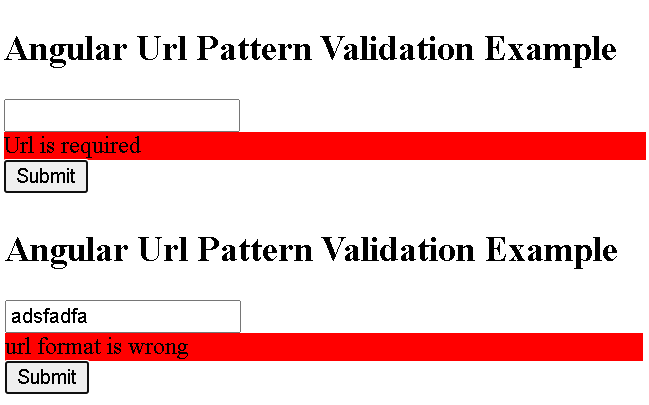
Angular html5 input type url pattern validation
In modern browsers, the Easy approach is to do URL pattern validation with inbuilt html5 language.
Another way to do URL validation is to define the input type=url
in the HTML element. However, this supports html5 only and modern browsers.
Input is defined with the following attributes.
<input type="url" name="websiteurl" id="websiteurl" placeholder="https://example.com" [(ngModel)]="websiteurl" [ngModelOptions]="{ updateOn: 'blur' }" #urlerror="ngModel" pattern="[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)? "
type=url
is an input textbox, that accepts URLs only pattern
attribute value is a regular expression pattern for URL values NgModel
directive is to support two-way binding from view to controller and vice-versa The ngModelOptions
directive enables to change of the behavior of the ngModel value, this will be fired with the updateon:blur
event.
Following is a complete code
<div>
<input
type="url"
name="websiteurl"
id="websiteurl"
placeholder="https://example.com"
[(ngModel)]="websiteurl"
[ngModelOptions]="{ updateOn: 'blur' }"
#urlerror="ngModel"
pattern="[-a-zA-Z0-9@:%_+.~#?&//=]{2,256}(.[a-z]{2,4})?\b(/[-a-zA-Z0-9@:%_+.~#?&//=]*)? "
required
/>
<div *ngIf="urlerror.invalid && (urlerror.dirty || urlerror.touched)">
<div *ngIf="urlerror.errors.required">
<span style="background-color: red">URL is required </span>
</div>
<div *ngIf="urlerror.errors.pattern">
<span style="background-color: red">Invalid URL </span>
</div>
</div>
</div>
In the component, just declare the input elements name here with a default value
import { Component } from "@angular/core";
import { FormControl, FormGroup, Validators } from "@angular/forms";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
heading = "angular html5 URL pattern validation example";
websiteurl: string = "";
}
Typescript url regexp validation
Sometimes, We want to do validation typescript files.
- It uses the same above regular expression pattern for URL validation
- RegExp object is created with regex
pattern.test
checked against supplied URL and return true-valid
public isValidUrl(urlString: string): boolean {
try {
let pattern = new RegExp(this.urlRegEx);
let valid = pattern.test(urlString);
return valid;
} catch (TypeError) {
return false;
}
}
}
}
console.log(this.isValidUrl('https://www.google.com')); // true
console.log(this.isValidUrl('google')); // false
Angular URL validation error not working
Let’s see what are the errors you will get when you are dealing with Angular forms
- error NG8002: Can’t bind to ‘formGroup’ since it isn’t a known property of ‘form’.
Application is unable to locate formGroup
.
formGroup
is a selector defined in the FormGroupDirective
directive. This directive is defined in ReactiveFormsModule
.
Conclusion
In this post, You learned
- Angular URL pattern validation example with reactive forms
- HTML 5 input type URL form validation
- Typescript URL validation