How to iterate array of object inside jsx render with examples in React
In this short tutorial, You learn how to iterate an array or object in the JSX render function.
In react components, the Render method returns the single element renders to the page.
Sometimes, we want to display child components with for loop. we can use a loop inside render JS. however, is It is tricky to iterate and display child components
This article explains about an employee’s array data is stored in react state, Display these in the child component using react props
with iteration in the parent component.
Let’s create EmployeeComponent.js
in react
This component reads the object from react props and displays it to a page with ul/li elements. Data received as an object, converted to individual fields using es6 object destruction syntax.
import React, { Component } from "react";
import "../index.css";
export default class EmployeeComponent extends Component {
render() {
const { id, name, salary } = this.props.data;
return (
<div>
<ul>
<li>Id:{id}</li>
<li>Name:{name}</li>
<li>Salary:{salary}</li>
</ul>
</div>
);
}
}
In the Parent component, a List of employee are iterated in React JSX using different syntax
Iterate array loop inside react jsx
First, react state object is initialized with an array of employee data.
In the render method, here are a sequence of steps
- Basic for loop syntax is used to iterate the array, You can check more about for loop
- Constructed an array of employee components child with data
- Please note that each child component is assigned with the unique key attribute, if you don’t pass this, you get an error like Each child in a list should have a unique “key” prop
- finally, the component array is placed inside a div element.
Here is the complete example code:
import React, { Component } from "react";
import EmployeeComponent from "./EmployeeComponent";
import TextControl from "./TextControl";
export default class EmployeeListComponent extends Component {
constructor() {
super();
this.state = {
employees: [
{
id: "1",
name: "Franc",
salary: "5000",
},
{
id: "11",
name: "John",
salary: "6000",
},
],
};
}
render() {
let list = [];
for (var i = 0; i < this.state.employees.length; i++) {
list.push(
<EmployeeComponent
key={i}
data={this.state.employees[i]}
></EmployeeComponent>,
);
}
return <div>{list}</div>;
}
}
Output:
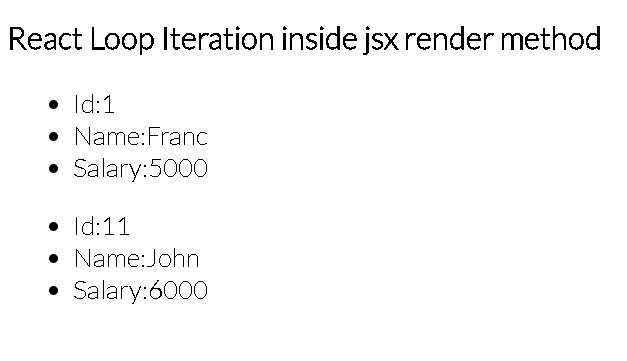
You can also rewrite the above using the map method in react jsx
map
is an inbuilt function in a JavaScript array, and it iterates each element of an array and returns a new array. This method has a call-back called for each element during iteration. It uses es6 arrow function for an call-back
with map, code as below
render() {
let list = [];
this.state.employees.map((employee, index) => {
list.push(<EmployeeComponent key={index} data={employee} ></EmployeeComponent >);
});
return <div>{list}</div>;
}
Another way is to rewrite using for-of syntax to iterate and render in react
for-of loop🔗 is new way to iterate objects.
render() {
var list = [];
for (const [index, employee] of this.state.employees.entries()) {
list.push(<EmployeeComponent key={index} data={employee} ></EmployeeComponent >);
}
return <div>{list}</div>;
}
Apart from the above approaches, you can different ways to iterate in javascript
Conclusion
As react render returns one element, before returning the list, construct the list using different approaches mentioned above and return a final array.