Best javascript Destructuring assignment tutorials and examples
- Admin
- Mar 6, 2024
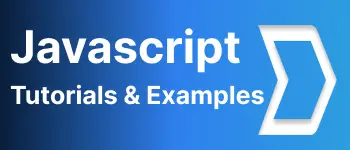
This tutorial covers ES6 destructuring assignment examples. ES6 introduced a new feature called destructuring assignment
.
You can check my previous posts on ES6 in another post:
What is Destructuring Assignment in JavaScript?
Destructuring assignment is a syntax change to extract data from objects, arrays, or nested iterable objects and assign them to variables.
Syntax:
let [variables] = array / object / nestedobject;
It contains two parts:
- The first part is the right side of the equal sign, i.e., iterable objects.
- The second part is the left side with an equal sign, i.e., variables.
Arrays/objects can be broken and destructured, assigning each part to corresponding variables on the left side of the equal sign.
Here, variables are either already existing or can be created using let
, const
, and var
keywords.
Before ES6 and Previous JavaScript Versions
Let’s consider an example where we have declared and initialized an array with two values:
let arrayExample = ["destructuring", "assignment"]
Now, to retrieve the values from this array and assign them to variables, traditionally we would use indexes to access elements:
let firstElement = arrayExample[0];
let secondElement = arrayExample[1];
The same code can be rewritten using destructuring assignment syntax as follows:
let arrayExample = ["destructuring", "assignment"];
let [firstElement, secondElement] = arrayExample;
console.log(firstElement);
console.log(secondElement);
Output from the above code execution:
destructuring
assignment
Array Destructuring Assignment Example
Arrays are groups of elements stored under a single name.
With array destructuring, the right side’s array values are broken down and assigned to variables based on the order of elements.
Here’s a basic example:
var var1, var2;
[var1, var2] = [15, 12];
console.log(var1); // 15
console.log(var2); // 12
Destructuring Assignment with Default Values
If the number of variables declared on the left side is more than the elements on the right side, excess items are not assigned.
Variables with default values are mapped to values from the array. If no matching index element is found in the array, default values are initialized.
let arrayExample = ["destructuring", "assignment"];
const [var1 = 'defaultone', var2, var3 ='defaulthree'] = arrayExample;
console.log(`var1: ${var1}, var2: ${var2}, var3: ${var3}`);
Output is
var1: destructuring, var2: assignment, var3: defaulthree
In this example, var1
and var3
are assigned default values since the array has only two elements. The first element is assigned to var1
, the second to var2
, and var3
is assigned a default value.
How to Skip Variables Initialization in Destructuring Assignment
Sometimes, we want to skip variables by using empty commas. These are called unknown variables.
unknown
variables are indicated by a empty comma in the desturcturing syntax.
let [, , testvar] = ["one", "two", "three", "four"];
console.log(testvar);
In the above example, Two commas are placed, Each comma represents a single unknown variable output is
three;
JavaScript Object Destructuring Assignment Examples
An object is a composition of different attributes stored under a single name.
The right-hand side of an equal operator is an object. We can destructure it by breaking it down and assigning its values to variables.
var employee = {
id: 12,
name: "Frank",
};
var { id } = employee;
var { name } = employee;
console.log(id); // outputs 12
console.log(name); // outputs Frank
Object Default Values Variables declared on the left side can be declared with default values.
var employee = { id: 11, name: "Tom" }; var newEmployee = {}; var { id: newId = 12 } = newEmployee; console.log(newId); // 12
Here,
newEmployee
is empty, and a local variable newId is created with a default value of 12. If the id property is not found in the object, the newId value is assigned the default value, which happens in this case.var { id: newId = 12 } = employee; console.log(newId); // 11
A local variable is created with the id value from the object. Since the id property exists in the object, the id value is assigned to the local variable. The output is 11 for the new id.
New Variable Names Assignment in javascript
Usually, we create variables with the same names as object properties. However, in some cases, we may want to create a new variable with a different name assigned with an object property.
var employee = { id: 11, name: "Tom" }; var { id: newId } = employee; console.log(newId); // 11
It creates a new local variable newId that is assigned with Employee.id newId is initialized with value 11 If you access the id variable, throws an error ‘Uncaught ReferenceError: id is not defined’.
Nested Object Destruction Assignment in JavaScript
An object can have a child object, as shown in the example below.
var employee = { id: 11, name: "Tom", address: { city: 'NewYork', Country:'USA' } }; var { address: { city} } = employee; console.log(city); // outputs Newyork console.log(address); // error: address is undefined
Here, the city local variable is created and assigned the value “New York”. The address variable is not created and serves as a locator for mapping child elements.
Destructuring looping/iteration
The
Object.entries
method is used to destructure the key-value pairs of an object during an iteration of a for loop:var employee = { id: 51, name: "John", }; for (let [key, value] of Object.entries(employee)) { console.log(`${key}:${value}`); // id:51 name:John }
REST Operator with Destructuring Assignment
The rest/spread operators introduced in ES6 can be used to assign variables with destructuring.
let [var1, var2, ...varRest] = ["one", "two", "three", "four","five"];
console.log(var1); // one
console.log(var2); // two
console.log(varRest[0]); // three
console.log(varRest[1]); // four
console.log(varRest[2]); // five
console.log(varRest.length); // 3
Destructuring Parameters from the Object Passed to a Function’s Parameters
Destructuring allows extracting id and name from an object passed as an argument to a function.
var employee = {
id: 11,
name: "Tom",
};
function getEmployee({name, id}) {
console.log('id: '+id + ' name: ' + name);
}
getEmployee(employee);
Conclusion
We have learned various examples of arrays and objects with destructuring assignment.