ES6 template String literals syntax and examples
- Admin
- Mar 10, 2024
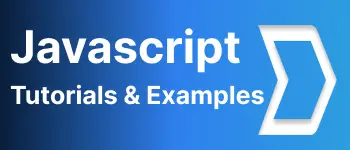
In this tutorial, we will delve into learning the basics of the Symbol primitive data type with examples.
Creating Template Strings in ES5 Example
A string is a sequence of characters or words enclosed in either single or double quotes. When incorporating variables into strings in ES5, it becomes cumbersome as it requires concatenation.
var name = 'Frank';
var message = 'Hello ' + name + ', How are you doing?';
This approach makes string manipulations tedious and prone to errors.
String literals in ES6 are enclosed in backticks
, offering a more straightforward solution for string interpolation.
ES6 Template String Literals Example
The same code can be simplified using backticks.
var name = 'Frank';
var message = `Hello ${name}, How are you doing?`;
console.log(message); // Hello Frank, How are you doing?
Introduction to Template and Tagged Literals Strings
ES6 introduces string literals with domain-specific syntax for interpolation, expressions, and multi-line strings. There are two types of template literals:
Template Literals
: String literals allowing string interpolation and multiline strings.Tagged Template Literals
: Function calls containing parameters passed via template strings.
String Interpolation Expression Example
Template strings support placeholders for dynamic variable values using dollar symbols and curly braces:
var m = 10;
var n = 4;
console.log(`Sum is ${m + n}`);
Multiline String Creation Example
In pre-ES6, multiline strings were created by appending line breaks. ES6 simplifies this process:
before ES6, we used to declare multi-line strings by appending line break
String msg=" Template strings are introduced in es6.\n Developer friendly for declaring string literals".
ES6 simplifies this process.
var msg = `Template strings are introduced in ES6.
Developer-friendly for declaring string literals`;
Just need to define a string literal with a separate lien enclosed in the back-tick (`…`) symbol. The output is the same for both strings examples.
Tagged Template String Example
Tagged templates enable function calls with template strings, where the function receives an array of values and remaining interpolation variables
The function has two arguments The first argument is an array of values the second argument is the remaining interpolation variable The function returned the manipulated string as the output
var name = 'Tom';
var salary = 5000;
function myFunction(stringsArray, nameExpression, salaryExpression) {
var array0 = stringsArray[0]; // Employee
var array1 = stringsArray[1]; // salary is
return `${array0}${nameExpression}${array1}${salaryExpression}`;
}
var result = myFunction`Employee ${name} salary is ${salary}`;
console.log(result);
The output of the above code execution is
Employee Tom salary is 5000
String raw() Method Example
The String.raw()
method returns the raw string from template string literals, ignoring escape character interpretations:
Syntax:
String.raw`templateString`
Example
var str = `Template \n String`;
console.log(str);
var rawString = String.raw`Template \n String`;
console.log(rawString);
var name = 'Frank';
var rawString1 = String.raw`Hi\n${name}, How are you doing?`;
console.log(rawString1);
The output of the above code execution is
Template
String
Template \n String
Hi\nFrank, How are you doing?
Escape characters are ignored, while interpolation expressions are preserved.