ES6 features- Arrow Functions Examples | ES6 tutorials
- Admin
- Mar 6, 2024
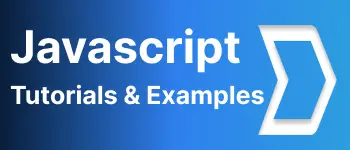
In this blog post, we’re going to delve into the basics of Arrow functions in JavaScript through examples.
Why Arrow Functions?
Let’s start by creating a standard function declaration in JavaScript. Such a function typically consists of a name, parameters, and a body.
function incrementByOne(value) {
return value + 2;
}
incrementByOne(2);
Now, let’s rewrite the above code using the new syntax - an equivalent function with a more concise syntax. This is an arrow function, which omits the function name, curly braces, and return statement. An implicit return is not necessary. The syntax includes the fat arrow =>
.
var incrementByOne = (value) => value + 2;
incrementByOne(2);
Syntax
(parameters) => {
statements / expression;
};
Here, parameters are optional, statements are the code to be executed, and expressions are code snippets enclosed within curly braces.
There are various ways to declare arrow functions, which we’ll explore through different examples.
No parameters syntax:
() => {
statements / expression;
};
One parameter syntax
value => { ... }
Multiple parameters Syntax
(x, y) => { ... }
Arrow functions example
var strs = ["one", "two", "three", "four"];
console.log(strs.map((str) => str.length));
Arrow Function Errors/Undefined Examples
However, there are certain cases where arrow functions do not behave as expected.
Usage with new and constructors: Using arrow functions with constructors will throw a TypeError.
function MyObj1() {} var myobj1 = new MyObj1(); console.log(myobj1); // outputs object var MyObj = () => {}; var myobj = new MyObj(); console.log(myobj); // TypeError: MyObj is not a constructor
Usage with Prototype Methods
Arrow functions with prototype methods return undefined.
var MyObj = () => {}; console.log(MyObj.prototype); // outputs undefined function MyObj1() {} console.log(MyObj1.prototype); // outputs object
The
yield
keyword also does not work in arrow functions.