How to Convert BigDecimal to Double or Double to BigDecimal in java with examples
- Admin
- Feb 5, 2024
- Java-examples
BigDecimal
is a class designed for handling arbitrary-precision signed decimal numbers. It comprises a 32-bit integer and an unscaled decimal value. This class is defined in the java.math
package and finds applications in various domains, including product prices and scientific calculations.
On the other hand, Double is used to store floating-point numbers and utilizes 64 bytes.
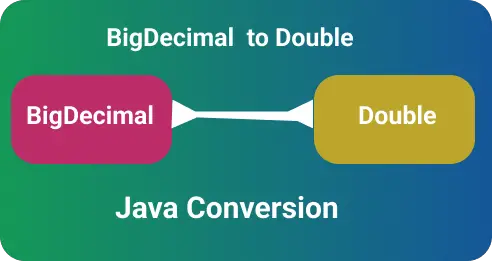
This tutorial will guide you on how to convert BigDecimal to double in Java.
Note: When dealing with currency values and converting them to double, precision loss occurs, leading to inaccurate results, especially in currency processing. Exercise caution in such scenarios.
You can also check my previous posts on the BigInteger
class in java.
- BigInteger Class tutorials
- Convert BigInteger to/from BigDecimal
- Convert BigDecimal to/from String
- BigInteger Divide example
- BigInteger Multiplication example
- Convert BigDecimal to float
- Top 10 BigInteger Examples
- Rounding bigdecimal to 2 decimal places
- Check BigDecimal contains Zero or not
- Convert BigInteger to/from ByteArray
How to Convert BigDecimal to Double in Java
The BigDecimal
class in Java provides a method named doubleValue for converting BigDecimal
to a double
value. Depending on the magnitude of the BigDecimal value, it returns either Double.NEGATIVE_INFINITY
or Double.POSITIVE_INFINITY
.
The syntax for the doubleValue method is as follows:
public double doubleValue()
Here is an example program code
import java.math.BigDecimal;
public class BigDecimalTest {
public static void main(String[] args) {
BigDecimal order = new BigDecimal(123.56789);
System.out.println(order); // 123.567890000000005557012627832591533660888671875
System.out.println(order.getClass()); // class java.math.BigDecimal
Double doubleValue = order.doubleValue();
System.out.println(doubleValue); // 123.56789
System.out.println(doubleValue.getClass()); // class java.lang.Double
}
}
Output:
123.567890000000005557012627832591533660888671875
class java.math.BigDecimal
123.56789
class java.lang.Double
How to Convert Double to BigDecimal in Java
This section explains two ways to convert a Double to BigDecimal in Java.
We can do it in two ways
Using BigDecimal Constructor
The constructor accepts Double values and returns a BigDecimal object.
public BigDecimal(double value)
Using valueOf Method
The static valueOf method takes the double value and returns a BigDecimal object.
public static BigDecimal valueOf(double val)
Here is a complete program code
import java.math.BigDecimal; public class BigDecimalTest { public static void main(String[] args) { Double d = 123.56789; System.out.println(d); //123.56789 System.out.println(d.getClass()); //class java.lang.Double // using constructor BigDecimal order = new BigDecimal(d); System.out.println(order); //123.67890000000005557012627832591533660888671875 System.out.println(order.getClass()); //class java.lang.BigDecimal // using valueOf method BigDecimal order1 = BigDecimal.valueOf(d); System.out.println(order1); //123.56789 System.out.println(order1.getClass()); //class java.lang.BigDecimal } }
Output:
123.56789 class java.lang.Double 123.567890000000005557012627832591533660888671875 class java.math.BigDecimal 123.56789 class java.math.BigDecimal
Summary
In summary, we’ve learned multiple ways to convert BigDecimal to or from double in Java. It’s crucial to be cautious when converting BigDecimal to double to avoid precision loss, especially in currency calculations.