BigInteger tutorial with example in Java
In this blog post, We are going to learn the BigInteger tutorial with an example in Java. You can also check my previous posts on BigInteger class in Java.
You can also check my previous posts on the BigInteger
class in Java.
- Convert BigInteger to/from BigDecimal
- Convert BigDecimal to/from String
- BigInteger Divide example
- BigInteger Multiplication example
- Convert BigDecimal to float
- Convert BigDecimal to double
- Top 10 BigInteger Examples
- Rounding bigdecimal to 2 decimal places
- Check BigDecimal contains Zero or not
- Convert BigInteger to/from ByteArray
BigInteger Class in Java
BigInteger is one of the java objects in java.math package introduced in JDK1.6.
Integer Primitive
type stores the numerical values between the range of (2 power 31 -1) to (-2 power 31).
Long Primitive
stores the numerical values between the range of (2 power 63 -1) to (-2 power 63).
For example, if you want to store arbitrary longer values in Integer or Long, it results in an error.
int value=1231212312312312312312312;
Java compiler throws this error as the value is out of range for integer.
To store these values, Java introduced a BigInteger
class.
There are some other problems solved with the BigInteger
class.
In another case, The Athematic operations on an integer or long, result in an overflow result. The result of these operations is always a larger value, if we use int or long, It results in a value with the range, saving the lower order 32 bits for integer,64 bits for long, and gives the lower range result. But, if we use BigInteger, it gives the correct result.
This can be used to store the result of big numbers which are not able to be stored in normal primitive types.
Another use case of the BigInteger
class is to store the result of numerous bit operations, and other mathematical functions, which can store the numeric values over 2 power 64 values.
How to create a BigInteger object in Java?
BigInteger provides multiple ways we can create.
The first way, create an object using the new
operator, and the constructor
accepts parameters either integer string number or long.
and the second way using the valueOf
static method introduced in the Java1.9 version.
BigInteger bigValue1,bigValue2;
// One way using Constructor
bigValue1=new BigInteger(123);
// Second way using valueOf method
bigValue2=BigInteger.valueOf(121);
or
// Constructor using string number
BigInteger bi= new BigInteger("578");
It is the immutable
class, When doing the arithmetic operation, It can’t change the existing value instead creates a new object with the modified value.
Any operations on BigInteger always return the new object.
Big Integer Example in Java
Here is an example of Java.
import java.math.BigInteger;
public class BigIntegerDemo {
public static void main(String args[]) {
int integerMaximumValue = 2147483647;// This is maximum value for
// integer type i.e 2 power 31
System.out.println("Case=1 = " + integerMaximumValue);
System.out.println("Case=2 = " + (integerMaximumValue + 1));
System.out.println("Case=3 = " + (integerMaximumValue * 2));
System.out.println("Case=4 = " + (integerMaximumValue * 4));
// All the above cases expect Case=1 gives wrong value as overflow
// occured
// All the below cases give expected values as BigInteger Object
// accommodates more values
BigInteger bigIntegerdemo = BigInteger.valueOf(integerMaximumValue);
System.out.println();
System.out.println("Case=5 " + bigIntegerdemo);
System.out.println("Case=6 " + bigIntegerdemo.add(BigInteger.ONE));
System.out.println("Case=7 "
+ bigIntegerdemo.multiply(BigInteger.valueOf(3)));
System.out.println("Case=8 "
+ bigIntegerdemo.multiply(BigInteger.valueOf(4)));
}
}
Output:
Case=1 = 2147483647
Case=2 = -2147483648
Case=3 = -2
Case=4 = -4
Case=5 2147483647
Case=6 2147483648
Case=7 6442450941
Case=8 8589934588
Attached are the methods available in BigInteger using the javap command.
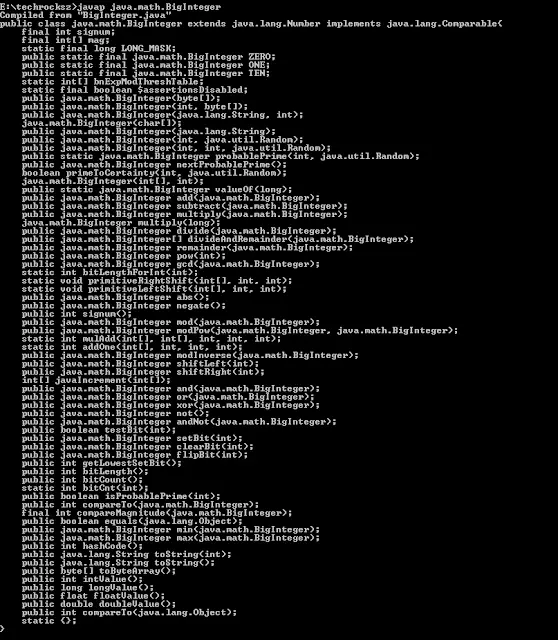
What is the range of BigInteger?
Every primitive has a range of lower and upper-bound values based on the JVM platform.
Whereas BigInteger
has no limit on the range of values stored. It supports larger values that support RAM for storage.
It is always bigger than the Long
and Integer
maximum values.
This topic has been a very basic start to explore on BigInteger example. Hopefully, you have enough information to get started. If you have any questions, please feel free to leave a comment and I will get back to you.