Angular 15 Sweetalert tutorial|popup notification in Angular example
In these tutorials, You learn step-by-step tutorials on how to add popup alerts using sweetalert in Angular 4/5/6/7/8/9/10/11/12/13 Versions.
To integrate into Angular applications, you need to learn the following npm libraries into Angular applications.
sweetalert2
is a JavaScript library used to display a nice popup window in NodeJS applications.
@sweetalert2/ngx-sweetalert2
is the npm library that supports Angular 8,9 and 10 Versions.
@sweetalert2/ngx-sweetalert2
is an npm library that is a wrapper on top of sweetalert2 that allows it to easily integrate into angular 4, 5, 6, and 7-based applications. In this article,
You can also check my previous posts on the sweetalert library.
How to integrate sweetalert in Angular 15?
This is a step-by-step guide for setup and the configuration for the latest Angular 15/11/10/9 application.
adding @sweetalert2/ngx-sweetalert2 npm library into application
First step, sweetalert2
and ngx-sweetalert2
are installed using below npm command
npm install --save sweetalert2 @sweetalert2/ngx-sweetalert2
It adds the latest versions in package.json and installs the node_modules folder.
"dependencies": {
"@sweetalert2/ngx-sweetalert2": "^10.0.0",
"sweetalert2": "^11.0.16"
}
Next, Add SweetAlert2Module
module in app.module.ts
, In this file please import SweetAlert2Module.forRoot()
in import section
Here is the complete app.module.ts code
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule } from "@angular/forms";
import { SweetAlert2Module } from "@sweetalert2/ngx-sweetalert2";
import { AppComponent } from "./app.component";
import { HelloComponent } from "./hello.component";
@NgModule({
imports: [BrowserModule, FormsModule, SweetAlert2Module.forRoot()],
declarations: [AppComponent, HelloComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
It provides the following components and directives.
- SwalDirective - This uses for a simple popup
- SwalComponent - It uses to customize with more advanced features.
- SwalPortalDirective
Angular 8 sweetalert example using @toverux/ngx-sweetalert2 library
Let’s see an example for SwalDirective in Angular 11
As you see, the Swal directive is added to the button.
It accepts an Array of parameters- [title, text,(type)]
- title - the title of the popup window
- text - Display text of window text
- type - optional icon displayed type warning, info, success, etc.
Here is a basic swal directive usage example
<h1>Sweet alert Angular example</h1>
<button [swal]="['Notification!', ' This is basic notification/', 'info']">
Click Me
</button>
Let’s see the SwalComponent
usage example.
In this example, this is code for showing a popup for deleting a record swal is a component that has SweetAlertOptions
options passed to this component.
swal component is declared and lazy-loaded, It has a reference #deleteRecord
<swal
#deleteRecord
title="Delete record?"
text="This cannot be undone"
icon="question"
[showCancelButton]="true"
[focusCancel]="true"
(confirm)="deleteFile(file)"
>
</swal>
One component is declared, You have to attach a reference to the button or anchor link.
<button [swal]="deleteRecord">Delete Record</button>
Or you can call the component using the reference fire
method
<button (click)="deleteRecord.fire()">Delete Record</button>
You can also programmatically call the sweet alert popup in the Angular/typescript component using the below component code
class DeleteComponent {
@ViewChild('deleteRecord') private deleteRecord: SwalComponent;
deleteRecord(){
this.deleteRecord.fire();
}
}
Stackblitz code
You can find a complete example - sweetlaert angular example🔗
@toverux/ngx-sweetalert2 integration Angular 6,7,8 versions
These are step by steps for the installation and setup of Angular versions below 8.
Installation and setup
Use npm to install sweetalert2 and ngx-sweetalert2 libraries.
npm install --save sweetalert2 @toverux/ngx-sweetalert2
It adds the below entries in package.json
"dependencies": {
"@toverux/ngx-sweetalert2": "^4.0.0",
"sweetalert2": "^7.26.9"
}
and also install this dependency and create the following folders in your project node_modules directory sweetalertangulardemo\node_modules\sweetalert2 sweetalertangulardemo\node_modules\@toverux\ngx-sweetalert2
Import SweetAlert2Module
First, you need to declare and import SweetAlert2Module into your main module. So that it will be available across your application. app.module.ts
import { BrowserModule } from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { AppComponent } from "./app.component";
import { SweetAlert2Module } from "@toverux/ngx-sweetalert2";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, SweetAlert2Module.forRoot()],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
Usage in Angular codebase
This library allows us to make use of defined directives as well as component. There are three ways to make use of different directives and components in your angular application.
- SwalDirective
- SwalComponent
- SwalPartialDirective
SwalDirective example usage
It is attributed \[swal\]
to an element where the click happened. SO you have to add this swal attribute to a button. Swal attribute contains the string arrays which accept format same as SweetAlertOptions\[swal\]={title:string,text:string,(type:string)}
swal("Simple Message"); swal("Simplemessage!", "Title inserted hehre")
swal("Sucess message", "Text here", "success");
swal attribute contains a type attribute, and possible values are success, error, warning, info, and input.
SwalComponent example usage
This component is used when there are too many options that need to pass in the swal attribute array. It is useful for advanced use cases.
<swal
#confirmFileDeletionSwal
title="Are you sure want to delete it?"
text="Delete record"
type="warning"
[options]="{ showCancelButton: true, confirmButtonText: 'Yes, deleted!', confirmButtonColor: '#DD6B55' }"
(confirm)="confirmDeleteFile()"
(cancel)="fileNotDeletedSwal.show()"
></swal>
you can call this swalComponent by using id or swal attribute
<button (click)="confirmFileDeletionSwal.show()">Delete the file</button>
or
<button [swal]="confirmFileDeletionSwal">Delete the file</button>
In the typescript component, you can access the component like this.
class AppComponent {
@ViewChild('confirmFileDeletionSwal') private confirmFileDeletionSwal: SwalComponent;
}
SwalPartialDirective directive
This is used angular templates with sweetalert code. Some popup components are replaced with views. You can use an ng-container with a swal component to achieve this. Examples app.component.html
<div class="example">
<button id="button1" [swal]="['Simple Message']">Simple Message popup</button>
<button id="button2" [swal]="['Simplemessage!', 'Title inserted hehre']">
Message popup with title under it
</button>
<button id="button3" [swal]="['Success', 'Text here', 'Success']">
Successful popu message
</button>
<button (click)="confirmFileDeletionSwal.show()">
Delete the imaginary file
</button>
<swal
#confirmFileDeletionSwal
title="Are you sure want to delete it?"
text="Delete record"
type="warning"
[options]="{ showCancelButton: true, confirmButtonText: 'Yes, deleted!', confirmButtonColor: '#DD6B55' }"
(confirm)="confirmFile()"
(cancel)="fileNotDeletedSwal.show()"
></swal>
</div>
typescript component contains the below code
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'sweetalertangulardemo';
public constructor(public readonly swalTargets: SwalPartialTargets) {
}
public confirmFile(): void {
this.deleteFile()
.then(() => this.fileDeletedSwal.show(), () => this.fileDeletionErrorSwal.show())
.catch(swal.noop);
}
public deleteFile(): Promise {
return new Promise((resolve, reject) => {
setTimeout(() => resolve()), 2000);
});
}
}
And the output of the above code is as seen in the below screenshot.
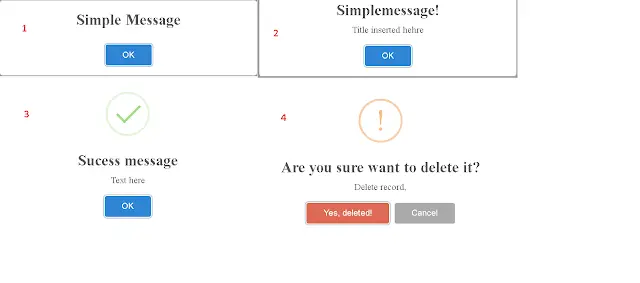
Conclusion
In this post, you learned how to integrate sweetalert in angular applications. This example is compatible with all angular versions.