Vuejs popup notification tutorial | Sweetalert2 example
- Admin
- Mar 10, 2024
- Sweetalert2 Vuejs
In this blog post, we will learn how to create a popup in a Vue.js application using the Sweet Alert framework.
You can check other posts on Sweetalert tutorials with examples and Alert Notifications with Angular here as follows
How to Add Sweetalert to Vue Component
Alerts are simple popup dialog boxes used to provide useful information to the user.
Vue.js is a popular progressive JavaScript framework for creating single-page applications.
Sweetalert🔗 is a vanilla open-source lightweight JavaScript library used to create popup notifications in JavaScript applications. These applications can be built using Jquery, Plain JavaScript, or NPM-based frameworks like Angular, Vue.js, and React.js.
With Sweetheart, We can achieve the following alert notifications
- Alert notifications to give success, warning, error, and informational message
- Popup to Prompt the user to take input information
- Confirmation alert
- Responsive
There are several Vue.js plugin wrappers available for Sweetalert:
In this blog post, we will integrate vue-sweetalert2 into a Vue.js-based application. The blog post covers two parts:
- Vue.js Application Creation using the Vue CLI tool
- Integrating Sweetalert into the Vue.js Application with examples
Vue.js Hello World Application Generation
Vue framework provides a vue-cli tool to create a Vue application from scratch using the vue create command.
B:\>vue create vuesweetalertdemo
Vue CLI v3.0.0-rc.8
? Please pick a preset: default (babel, eslint)
Vue CLI v3.0.0-rc.8
✨ Creating a project in B:\Workspace\blog\vuesweetalertdemo.
⚙ Installing CLI plugins. This might take a while...
> [email protected] install B:\Workspace\blog\vuesweetalertdemo\node_modules\yorkie
> node bin/install.js
setting up Git hooks
can't find .git directory, skipping Git hooks installation
added 1281 packages in 278.557s
� Invoking generators...
� Installing additional dependencies...
added 3 packages in 18.413s
⚓ Running completion hooks...
� Generating README.md...
� Successfully created project vuesweetalertdemo.
� Get started with the following commands:
$ cd vuesweetalertdemo
$ npm run serve
This will create the vuesweetalertdemo
application with a predefined Vue folder structure and initial configuration. Go to the application folder, run the npm run serve command to start the Vue.js application.
Install and Save Dependencies to the Application
To integrate SweetalertJS into the Vue application, go to the application root folder. The vue-sweetalert2 package can be installed using npm, yarn, or bower.
npm install -save vue-sweetalert2
or
bower install vue-sweetalert2
or
yarn add vue-sweetalert2
This command will do the following:
- Install
vue-sweetalert2
in your application and create a folder node_modules/vue-sweetalert2 in the application root folder. - Add the below entry in package.json:
"dependencies": {
"vue-sweetalert2": "^1.5.5"
},
Import VueSweetalert2 Module in Vue Application
To make use of the third-party npm package functionality, first, you need to import the VueSweetalert2
module in main.js
. Once imported, the application can access Sweetalert
objects and their methods.
main.js
import Vue from "vue";
import App from "./App.vue";
import VueSweetalert2 from "vue-sweetalert2";
Vue.use(VueSweetalert2);
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App),
}).$mount("#app");
Vue.js Popup Simple Alert Example
This example application has one button. On clicking the button, it shows an alert box with a simple “OK” button.
App.vue.
<template>
<div id="app">
<div><img alt="Vue logo" src="./assets/logo.png" /></div>
<button v-on:click="displaySimplePopup">Click Me</button>
</div>
</template>
<script>
export default {
data() {
return {
msg: "",
};
},
methods: {
displaySimplePopup() {
// Use Sweetalert
this.$swal("Welcome to Vuejs Application using Sweetalert");
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
the output of the above code is
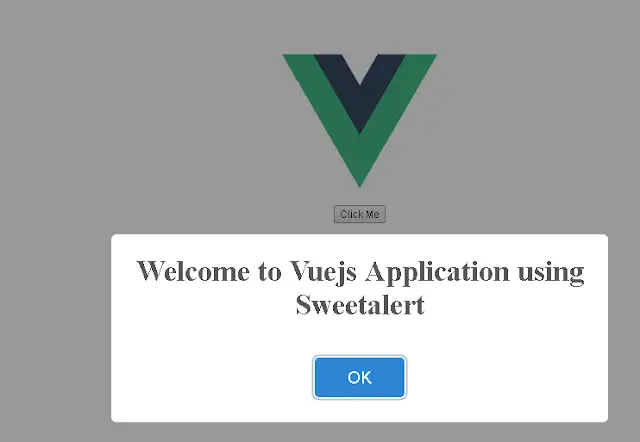
Sweetalert Modal Examples in Vuejs
The demo covers:
- Displaying a successful dialog window
- Showing an informational dialog window
- Displaying the error dialog window
- Modal window prompting the user to take input
- Changing the alert display position
- Delete popup notification
<template>
<div id="app">
<div><img alt="Vue logo" src="./assets/logo.png" /></div>
<button v-on:click="displaySimplePopup">Click Me</button>
<button v-on:click="infoAlert">Info Popup</button>
<button v-on:click="successAlert">Success Popup</button>
<button v-on:click="errorAlert">Error Popup</button>
<button v-on:click="AlertPassingData">Prompt Popup</button>
<button v-on:click="positionPopup">Custom Position Popup</button>
<button v-on:click="deletePopup">Delete Popup</button>
</div>
</template>
<script>
export default {
data() {
return {
msg:''
};
},
methods: {
displaySimplePopup(){
// Use Sweetalert
this.$swal('Welcome to Vuejs Application using Sweetalert');
},
infoAlert() {
this.$swal({
type: 'info',
title: 'Title Info',
text: 'This is an informational message
});
},
successAlert() {
this.$swal({
type: 'success',
title: 'Title Success',
text: 'This is a successful message'
});
},
errorAlert() {
this.$swal({
type: 'error',
title: 'Error Title ...',
text: 'Error Occurred!',
footer: '<a href>Please click this for more about this error</a>'
});
},
positionPopup() {
this.$swal({
position: 'top-end',
type: 'success',
title: 'Data is saved in Database',
showConfirmButton: false,
timer: 1000
});
},
deletePopup() {
this.$swal({
title: "Do you want to delete this record",
text: "This will be recorded from Database",
type: "warning",
showCancelButton: true,
confirmButtonColor: "#4026e3",
confirmButtonText: "Yes, remove it!"
}).then((result) => { // <--
if (result.value) { // <-- if accepted
del('status-delete/' + id);
}
});
},
AlertPassingData() {
this.$swal({
title: 'Please enter String?',
input: 'text',
inputPlaceholder: 'Enter String here',
showCloseButton: true,
});
},
}
}
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
Output is
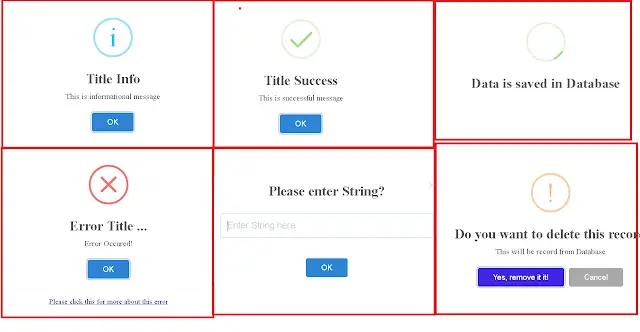
HTML Content in Sweetalert Popup
The alert
dialog can provide both static and dynamic content. Dynamic content can be placed using the HTML attribute of the $swal
function.
It can also contain HTML custom forms to take input from users. Here is an example of HTML content in the dialog window:
<button v-on:click="htmlContentAlert">Html contentPopup</button>
htmlContentAlert { this.$swal({ title: "
<i>This is title</i>
", HTML: "This is HTML text:
<b>bold</b>
<i> italic</i>
", confirmButtonText: "V
<u>Yes</u>
", });; },
Vuejs Sweetalert Ajax demo usage
This is an example of Ajax for the asynchronous processing of a request. It shows a loading icon image on processing the Ajax request.
<button v-on:click="ajaxAlert">Ajax Popup</button>
ajaxAlert() {
this.$swal({
title: "Asynchronous example",
text: "Demo application for Ajax example",
type: "info",
showCancelButton: true,
closeOnConfirm: false,
showLoaderOnConfirm: true
}, function () {
setTimeout(function () {
swal("Completed!");
}, 2000);
});
},
The code for this blog post can be downloaded from here🔗