How to get Current date, time timestamp in Vuejs with example
Date and time values are specific to the machine that is running.
First, the client side is linked to the current machine browser, Another is the server side where the backend machine is running.
In my previous post, You learned the current timestamp in javascript and ReactJS.
It is very simple in the Vuejs Application If you are already aware of getting a current date in JavaScript.
Javascript provides a Date object and holds date, time, and timezone information. It provides various methods to provide the current date information.
Vuejs always runs on client browsers, Getting the date and time in Vue is client-side only.
This post talks about getting the current date and timestamp in vuejs.
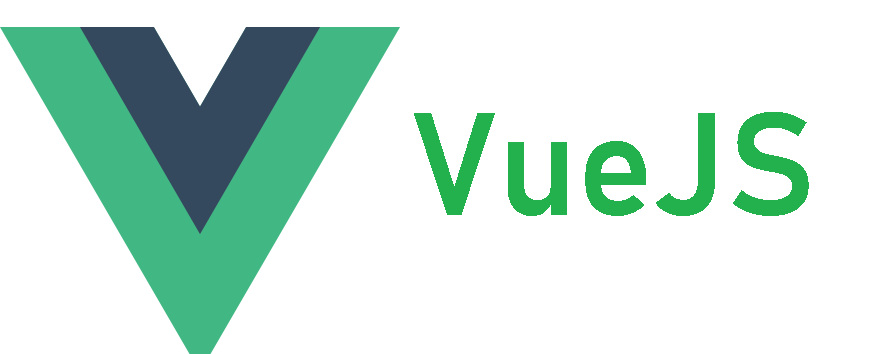
You will learn the following things in vuejs
- Get the Current Date in DD/MM/YYYY format
- Get current time only
- Current UNIX epoch timestamp in UTC
- Retrieve the current Year only
Get Current Date, Year, time/timestamp in vuejs
In the Vuejs Application, Below are steps to get date information.
- Create a Vue component for this example
- Initialize the following global variables in the data function and return this as an object.
data: () => ({
timestamp: "",
date: "",
time: "",
currentYear: "",
}),
Created functions in the methods section.
getDate: function () { return new Date().toLocaleDateString(); },
- Call the methods on vue component load using the mounted callback function and update the variables in the data object.
new Date().toLocaleDateString() return the Date in MM/DD/YYY format
toLocaleTimeString() returns the time in HH:MM:SS AM format
getFullYear() in Date object returns year value only
now() method in date object returns the current Unix timestamp since epoch time
Complete example code
<template>
<div>
<div v-show="timestamp">Timestamp:{{ timestamp }}</div>
<div v-show="date">Date:{{ date }}</div>
<div v-show="time">Time:{{ time }}</div>
<div v-show="currentYear">Only year:{{ currentYear }}</div>
</div>
</template>
<script>
export default {
name: "CurrentDate",
data: () => ({
timestamp: "",
date: "",
time: "",
currentYear: "",
}),
props: {
msg: String,
},
methods: {
getDate: function () {
return new Date().toLocaleDateString();
},
getTime: function () {
return new Date().toLocaleTimeString();
},
getTimestamp: function () {
return Date.now();
},
getCurrentYear: function () {
return new Date().getFullYear();
},
},
mounted: function () {
this.date = this.getDate();
this.time = this.getTime();
this.timestamp = this.getTimestamp();
this.currentYear = this.getCurrentYear();
},
};
</script>
<style>
</style>