ReactJS, How to convert unix Timestamp to/from Date example
This post talks about converting timestamp to/from date in reactjs/javascript.
In front-end applications, Date manipulation is a little bit different as client and server dates are different.
Sometimes, data containing date-related information returned from backend API needs to be formatted and converted to date. Reactjs applications offer two approaches
using moments library Intl DateTimeFormat
Timestamp is Unix style long value returning milliseconds elapsed from 1970/1/1 UTC
In javascript, the Following are ways javascript is offering to get the current timestamp.
You can check my previous post about Current timestamp in javascript
Date.now();
new Date().getTime();
new Date().valueOff;
Note, There are two timestamps, First local timestamp which is client-side running on the client timezone, Other is UTC timezone timestamp USA server’s Zone All the above lines of codes return epoch Unix timestamp since 1971-01-01 time.
How to convert Unix timestamp to Date in reactjs
There are multiple ways, can convert epoch timestamp to date format in reactjs.
Intl.DateTimeFormat format method
In javascript, Intl.DateTimeFormat🔗 provides manipulation methods for date and timestamp related things.
DateTimeFormat accepts locale information as the first parameter, and the second parameter is the option It returns an object of Intl.DateTimeFormat, the calling format method will return the required date
Here is an example: Convert timestamp to date and time
let currentTimestamp = Date.now();
console.log(currentTimestamp); // get current timestamp
let date = new Intl.DateTimeFormat("en-US", {
year: "numeric",
month: "2-digit",
day: "2-digit",
hour: "2-digit",
minute: "2-digit",
second: "2-digit",
}).format(currentTimestamp);
Locale is given as en-US
, output desired date format is supplied in the second parameter as an object.
Please note that all the output dates are converted to USA date and time format only
Output:
console.log(date);
1610380287270
01/11/2021, 9:21:27 PM
Here is an example: Convert timestamp to date only
let date = new Intl.DateTimeFormat("en-US", {
year: "numeric",
month: "2-digit",
day: "2-digit",
}).format(currentTimestamp); // 01/11/2021
example : Convert timestamp to time only
let date = new Intl.DateTimeFormat("en-US", {
hour: "2-digit",
minute: "2-digit",
second: "2-digit",
}).format(currentTimestamp); //9:27:16 PM
Let’s see an example in react to convert an array of timestamps into date
Complete example:
import React, { Component } from "react";
const empsApiData = [
{
id: "1",
name: "Franc",
createdAt: 1610378858273,
},
{
id: "21",
name: "John",
createdAt: 1610378858273,
},
{
id: "33",
name: "John",
createdAt: 1610378858273,
},
{
id: "2",
name: "Krish",
},
];
class ListComponent extends Component {
constructor(props) {
console.log("kiran ", empsApiData);
super(props);
const newEmps = empsApiData.map((emp) => ({
id: emp.id,
name: emp.name,
createdAt: new Intl.DateTimeFormat("en-US", {
year: "numeric",
month: "2-digit",
day: "2-digit",
hour: "2-digit",
minute: "2-digit",
second: "2-digit",
}).format(emp.createdAt),
}));
this.state = {
emps: newEmps,
};
}
render() {
return (
<div className="App">
<ul>
{this.state.emps.map((item, index) => (
<li key={item.id}>
<span>{item.name} </span>
<span>{item.createdAt} </span>
</li>
))}
</ul>
</div>
);
}
}
export default ListComponent;
Output seen in the browser is
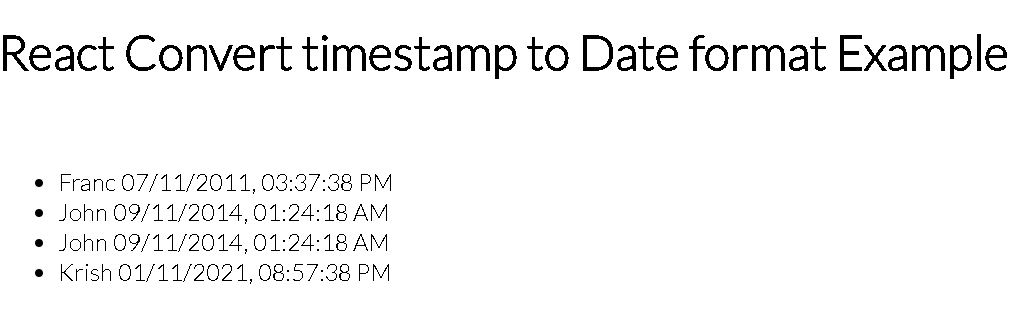
momentjs format ‘YYYY-MMM-DD’
First, install the moments npm library as seen below.
npm install momentjs --save
Next, import the moment
class into react component.
import moment from 'moment'.
In react component, pass the timestamp to the moment object, call the format method with parameter ‘L’, L means localized date formats.
moment(emp.createdAt).format('L') returns 07/11/2011
There is another way you can use customize the format.
moment(emp.createdAt).format("YYYY-MMM-DD");
How to convert Date into a timestamp in reactjs
Here date can be a date object or string date, which is converted to a Unix timestamp in milliseconds.
getTime method
It is simple to convert the timestamp from the given date.
First, You have to convert the string to a Date object using new Date(String)
Next, call getTime() method on date object to return Unix timestamp
console.log(new Date("2021-01-01").getTime()); // 1609459200000
Example: to convert current date to current timestamp
let date = new Date();
var timestamp = date.getTime();
momentjs valueOf method
valueOf method return Unix seconds since epoch time.
Multiple with 1000 to get timestamp in mili seconds
moment("01/01/2021", "YYYY-MM-DD").valueOf() * 1000;
Conclusion
In this post, you will learn multiple ways to convert date to/from Unix timestamp in reactjs/JavaScript.
Hope it helps :-)