Generates Javascript Documentation - ESDoc Tool
- Admin
- Mar 10, 2024
- Javascript
In my previous post, we covered the JavaScript documentation tool using JSDoc.
In this blog post, we are going to learn about the ESDoc tool-generated documentation for JavaScript and the latest ECMAScript standards.
Why is JavaScript Documentation Required?
ESDoc
is a documented API generator for JavaScript applications written in ES6 language. It supports writing documentation for ECMAScript code.
Documentation for any application is critical for its success. When you develop an API for clients, the first thing they need is good documentation about the API. It helps developers to use the API easily.
ESDoc is based on a plugin-based architecture, where everything is configured based on plugins. It parses tags from comments in JavaScript code and generates HTML documentation.
Difference between ESDoc and JSDoc Tools
JSDoc is a popular Javascript documentation API generator. ESDoc follows the same approach.
ESDoc | JSDoc |
---|---|
- It supports ES5, ES6, ES7, or later | - This has supported ES5 and ES3 javascript versions |
- Documentation code coverage | This follows Object-oriented and Prototype-based OOP concepts Many tags have support |
- Integration with test code | - Very difficult to extend the functionality |
- Plugin-based support for extending to different modules | |
- Lesser tags that support ES-based style syntax | Data passed to component |
ESDoc Features
- Supports JavaScript documentation for the latest ECMAScript
- Documentation code coverage
- Test coverage included in the documentation
- Can be hosted independently
- Supports ECMAScript proposal specs
Installation and Setup of ESDoc
ESDoc
provides npm packages. First, you need to install ESDoc and the ESDoc standard plugin to use the ESDoc tool.
npm install --save-dev esdoc esdoc-standard-plugin
This installation provides the esdoc
command-line tool. To check whether the installation was successful, issue the following command:
B:\esdocexample>esdoc -v
1.1.0
ESDoc Command Line Options
B:\esdocexample>esdoc -h
Usage: esdoc [-c esdoc.json]
Options:
-c specify config file
-h output usage information
-v output the version number
ESDoc finds configuration by the order:
1. `-c your-esdoc.json`
2. `.esdoc.json` in the current directory
3. `.esdoc.js` in the current directory
4. `esdoc` property in package.json
ESDoc has an option -c
to configure configuration files. Without -c
, it considers .esdoc.json
or .esdoc.js
files in the current directory, which is the ESDoc configuration file.
Configuration files are considered based on the order of the files without the -c
option provided. .esdoc.json
file or .esdoc.js
can also be configured using the esdoc property in package.json
or esconfig.json.
{
"source": "./src",
"destination": "./docs",
"plugins": [
{
"name": "esdoc-publish-html-plugin"
}
]
}
- source: JavaScript source code directory path
- destination: JavaScript output destination directory
- plugins: You can configure plugins to extend functionality.
How to Generate Documentation using the ESDoc Command Line
Here is a JavaScript code:
/**
* This is Calculator class
* @example
* let class = new Calculator();
*/
export default class Calculator {
/**
* this is the Calculator constructor description.
*/
constructor() {}
/**
* addition of two numbers
* @param {number} param1 number to add.
* @param {number} param2 number to add.
* @return {number} returns the sum of two parameters.
*/
add(param1, param2) {
return param1 + param2;
}
/**
* this is a method description.
* @returns {string} this is a return description.
*/
method() {
return "message";
}
}
We will use the esdoc command-line tool to generate HTML documentation. Please use the following command to generate it.
esdoc
// -c option for esdoc custom configuration
esdoc -c esdoc.json
// esdoc installed locally, using local esdoc tool
./node_modules/.bin/esdoc -c esdoc.json
Here is a command output
B:\esdocexample>esdoc
parse: B:\esdocexample\src\Calculator.js
resolve: extends chain
resolve: necessary
resolve: ignore
resolve: link
resolve: markdown in description
resolve: test relation
output: B:\esdocexample\docs\identifiers.html
output: B:\esdocexample\docs\index.html
output: B:\esdocexample\docs\class\src\Calculator.js~Calculator.html
output: B:\esdocexample\docs\file\src\Calculator.js.html
output: B:\esdocexample\docs\css
output: B:\esdocexample\docs\script
output: B:\esdocexample\docs\image
output: B:\esdocexample\docs\script\search_index.js
output: B:\esdocexample\docs\source.html
Here is the output of HTML generation file:
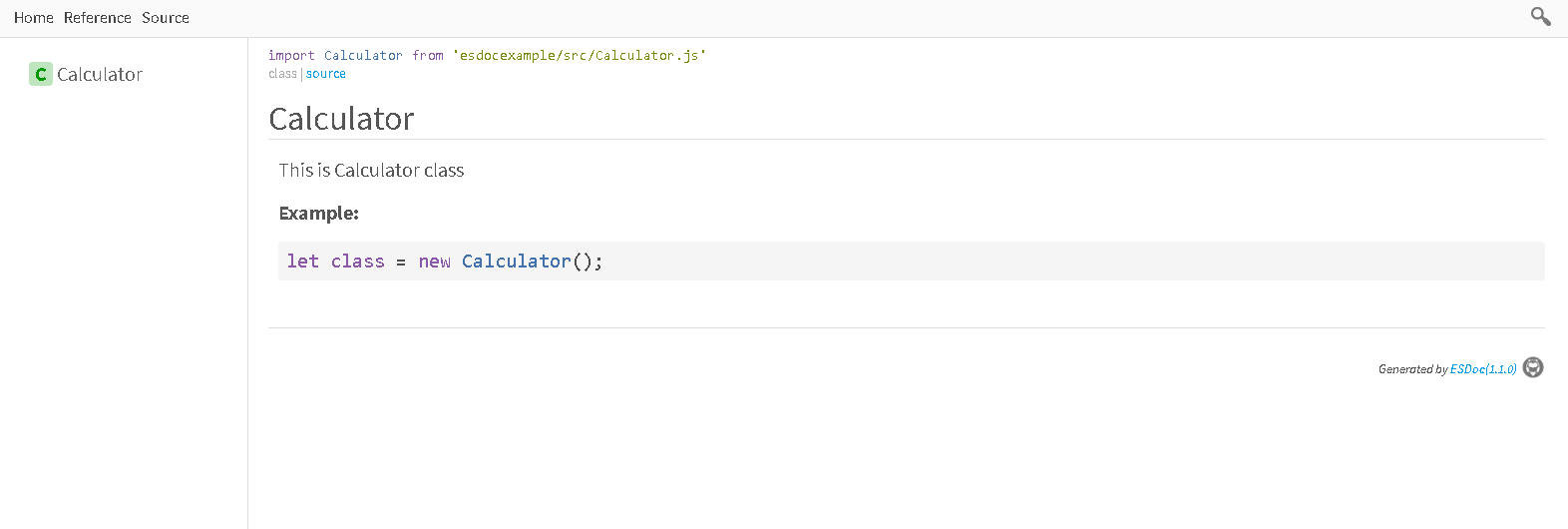