Learn JSDoc - Javascript API document Generator tutorials
- Admin
- Dec 31, 2023
- Javascript
Javascript Documentation
JsDoc is a javascript documentation generator for javascript language. This tool is used to generate code documentation in HTML format of a javascript source code and it is similar to Javadoc in java language. Strong documentation is one of the factors for a successful software application.JSDoc parse javascript source with content from /** */ and outputs HTML documentation.
This blog post covers JSDoc tutorials with examples
Install and Setup JSDoc library using npm library
To Play with this, first, you need to install it. the installation provides command-line tools - jsdoc. This library was available as an npm package manager. First, make sure that Nodejs is installed, npm, and node command works successfully by the following command.
B:\blogger>npm --version
5.6.0
B:\blogger>node --version
v8.10.0
Once installed nodejs, Next step is to install jsDoc npm package
npm install -g jsdoc
It installs the jsdoc package in a global location. I am doing the installation on windows. see Global npm install location on windows which is an npm location folder on the window is %APPDATA%\npm\node_modules\jsdoc. This is a global location for the node_modules module folder To find the jsdoc version. Please issue the command below.
B:\blogger>jsdoc --version
JSDoc 3.5.5 (Thu, 14 Sep 2017 02:51:54 GMT)
JSDoc Basic Example
The simple function declared with two parameters with return type The sample code is in helloworld.js
/**
* Adding two numbers.
* @param {value1} firstParameter First parameter to add.
* @param {value2} secondParameter Second Parameter to add.
* @returns {string}
*/
function add(value1, value2) {
return value1 + value2;
}
Then run the following command to generate HTML documentation.
jsdoc helloworld.js
Generated HTML file as per the below screenshot
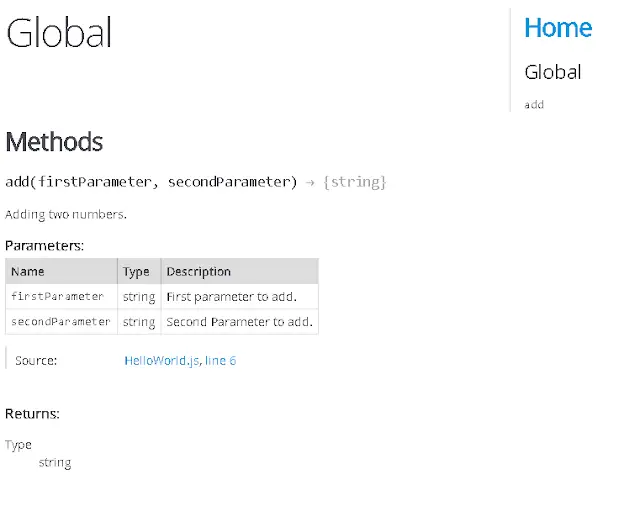
Syntax
/**
* Adding two numbers.
* @param {string} firstParameter First parameter to add.
* @param {string} secondParameter Second Parameter to add.
* @returns {string}
*/
Explanation
- Jsdoc contains multiline comments in addition to the first character is asterisk Each comment includes symbol @ and tag name. @param is one tag here Annotation types, It includes type name in braces
@param {string}
name
JSdoc comments example
Comments are similar to multi-line comments and the difference is the extra asterisk symbol Comments contain HTML tags as well
// Single line comment
/*
* multi-line comment
*/
/**
* Jsdoc comments
*/
JSDoc function example
Added a few metatags @fileOverview,@Author,@Version to the function
/**
* This is a hello world function.
* @fileOverview Employee functions.
* @author [Kiran Babu](mailto:support@cloudhadoop.com)
* @version 1.0.0
* @param {string} value - A string param
* @return {string} Return a string
*
* @example
*
- displayEmp('Welcome')
*/
function displayEmp(msg) {
return msg;
}
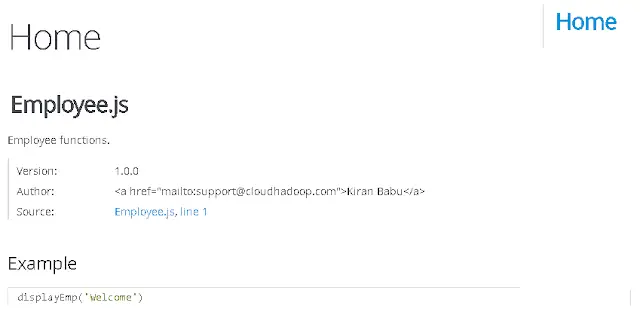
How to return the method as void?
You have to use the @return tag to document the method function with type. Type can be used in curly braces In javascript, a void exists as a type, you can also use undefined.
@returns {void} returns nothing
@returns {undefined} returns undefined
What are valid param tag types in jsdoc? @param tag is used to tag parameter types to a function or method. You can also use @arg or @argument instead of @param @tag contains the name, type, and description of a parameter to a function
/**
* @param {string} argument name of the employee.
*/
Here, the name of is argument type is a string enclosed in curly braces description is the name of the employee You can use javascript inbuilt data types as a value for type parameters Boolean, Null, Number, String, Undefined, Symbol, Object, Optional and default parameter declaration Parameters declared using @param tag, To make it this param option. Let us declare an optional parameter say the message of type string
@param {string} [message] // or
@param {string=} message
@param {string} [message='hello'] // default value initialization
How to declare the mixed type of parameters?
Use | symbol separator to add multiple types for a single parameter in a method or function declaration.
/**
* Utility method
* @param {(string|number)} argument The input parameter to function
* @returns {(string|number)} The modified return value from a function.
*/
function doSomething(argument) {
return argument;
}
how to represent a multidimensional array of objects param type? Multidimensional is an array of objects. which can be represented in jsdoc comments using type - Object.<Array\[\]>
How to write a comment for a callback of a function?
Jsdoc provides a @callback tag. It has information like parameters and returns values about the callback function
/**
* Callback for multiplying two numbers.
*
* @callback multiCallback
* @param {int} multiply - An integer.
*/
/**
* Multiply two numbers, and output the results to a callback function.
*
* @param {int} x - An integer.
* @param {int} y - An integer.
* @param {multiCallback} callback - A callback to execute.
*/
function Multiply(a, b, multiCallback) {
multiCallback(a * b);
}
How to Document javascript classes?
@class or @constrcutor are used to mark it as a class or construction which can be created using the new operator
/**
* Creates a new Employee.
* @class Employee class
*/
function Employee() {
/** @lends Employee */
{
/**
* Create an `Employee` instance.
* @param {string} name - The Employee's name.
*/
initialize: function(name) {
this.name = name;
},
}
var e = new Employee();
@lend tag used to document all members of a class.
JSDoc Frequently Used annotations
Jsdoc supports the following tags and syntax is mentioned. All this syntax should be used included in jsdoc syntax (Adding asterisk to multiline comments)
Tag
Description
Usage/Syntax
- @Author
Adds Author of code, supports to add email
@author
Kiran
- @class
Marks function as a class instance that can be created using the new keyword
@class
Employee class
- @constructor
Marks function as a constructor and same as @class
@constructor
Employee class
- @deprecated
Specifies a comment to tell that this method is going to be deprecated
@deprecated
from version 2.5
- @throws
To indicate the exception or error that a method might throw it.
@throws {Exception} @throws
Throws an error if the parameter is null
- @param
Adding documentation for parameters to method or function
@param {Number}
numericValue numeric value to parameter.
- @private
mark this as private
@private
- @return or @returns
return value documentation of a method or function.
@returns {string}
- @version
the version number of a code.
@version 1.0
- @interface
This makes interfaces
@interface Animal
- @static
Marker for a Static variable, This can be accessed without an instance
@static
- @typedef
Marker for custom data types. These can be used in other tags like normal type params
@typedef {(number|string)} customType
- @todo
Document the things that need to complete in the future.
@todo
Write Edge cases and documentation for this method.
- @lends
Document all the members of a class or object
@lends
Employee