How to show/hide div on button click in Vuejs| toggle
Sometimes, you may need to hide a div or an HTML element with a button click event and then show it again by clicking on the button. The toggle
function acts as a switch that dynamically hides or shows elements based on the truthy value of an attribute.
In this guide, you will learn multiple methods to show or hide a div by clicking a button.
How to Hide/Show a Div on Button Click
- Using v-if and v-else Directives
- Using the v-show Directive
- Using the :class Attribute
First, create a component with the default template, script, and style sections.
Next, define the button using a <button>
tag:
<button>click Me</button>
Add a button onclick handler using the @click directive as follows.
<button @click="isShow = !isShow">click Me</button>
Upon clicking the button, the isShow
attribute is assigned the inverse of its existing value.
In the Vue component script, define the boolean attribute isShow
in the data function. The data
function returns an object with the property isShow initially set to false:
export default { name: "ToggleDiv", data: function () { return { isShow: true,
}; }, props: { msg: String, }, };
using v-show directive
v-show directive is a built-in vuejs for conditionally displaying the elements.
Here, the div element is added with the v-show directive, which is shown to the user if isShow=true, else hide the div
<div v-show="isShow">Content testing</div>
Well, How does v-show work?
the div element is always added to the DOM tree irrespective of the truthy value
For v-show=true
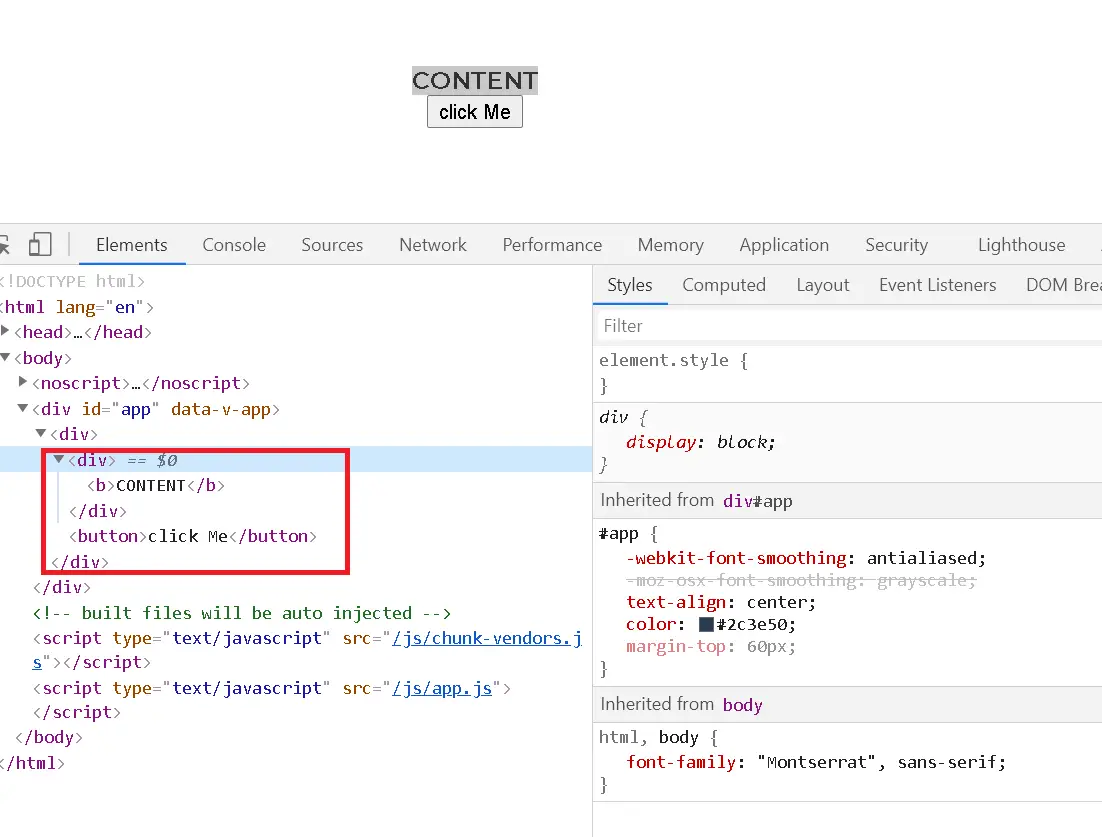
For v-show=false
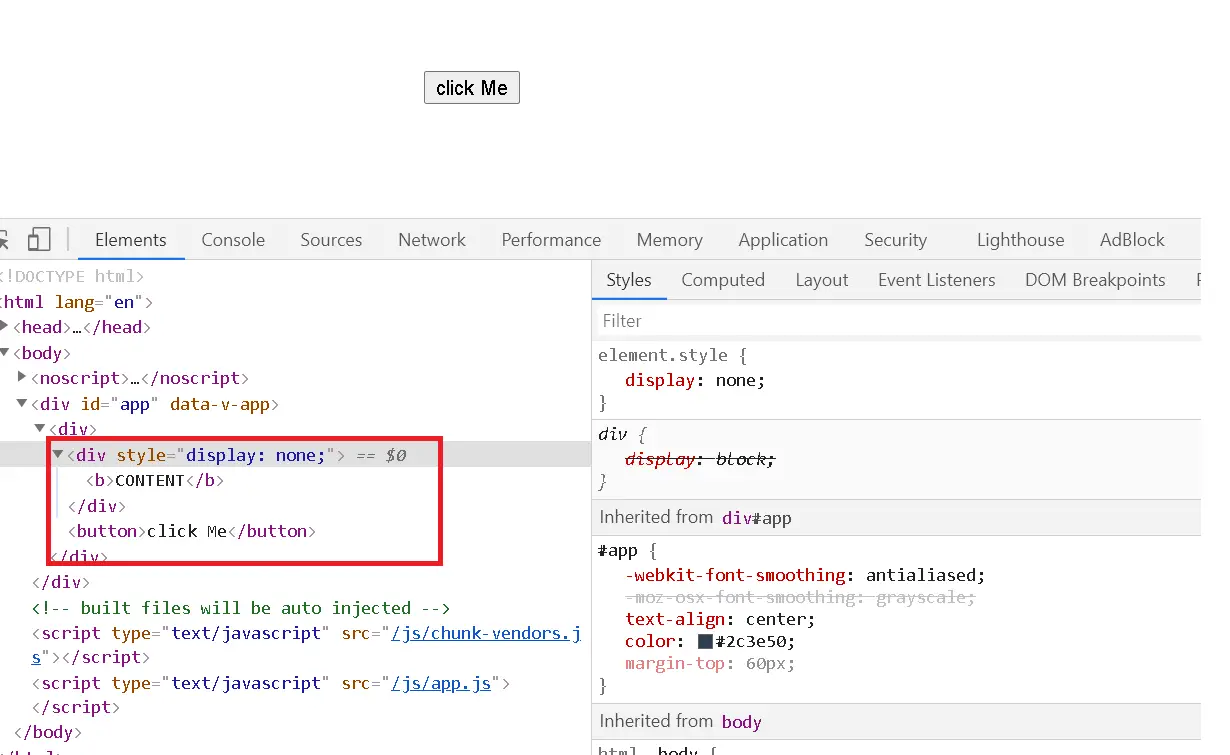
This means that the div element is rendered always in the DOM tree irrespective of the truth value. Hence, because of this, the toggle switch is controlled using the inline display: none property added to it.
using v-if else directive
Another way is to hide the element by clicking a button.
v-if
is a vue directive display content conditionally.
Following is the usage example
<template>
<div>
<div v-if="isShow">Content</div>
<button @click="isShow = !isShow">click Me</button>
</div>
</template>
you can also add the v-else
directive for v-if that works as if-else in any programming language.
The advantage of v-if, the element is not added to the DOM tree when an element is hidden.
The div element is added/deleted dynamically, Hence, the performance of the DOM is improved, which makes the page load faster. You can check the console log as shown below.
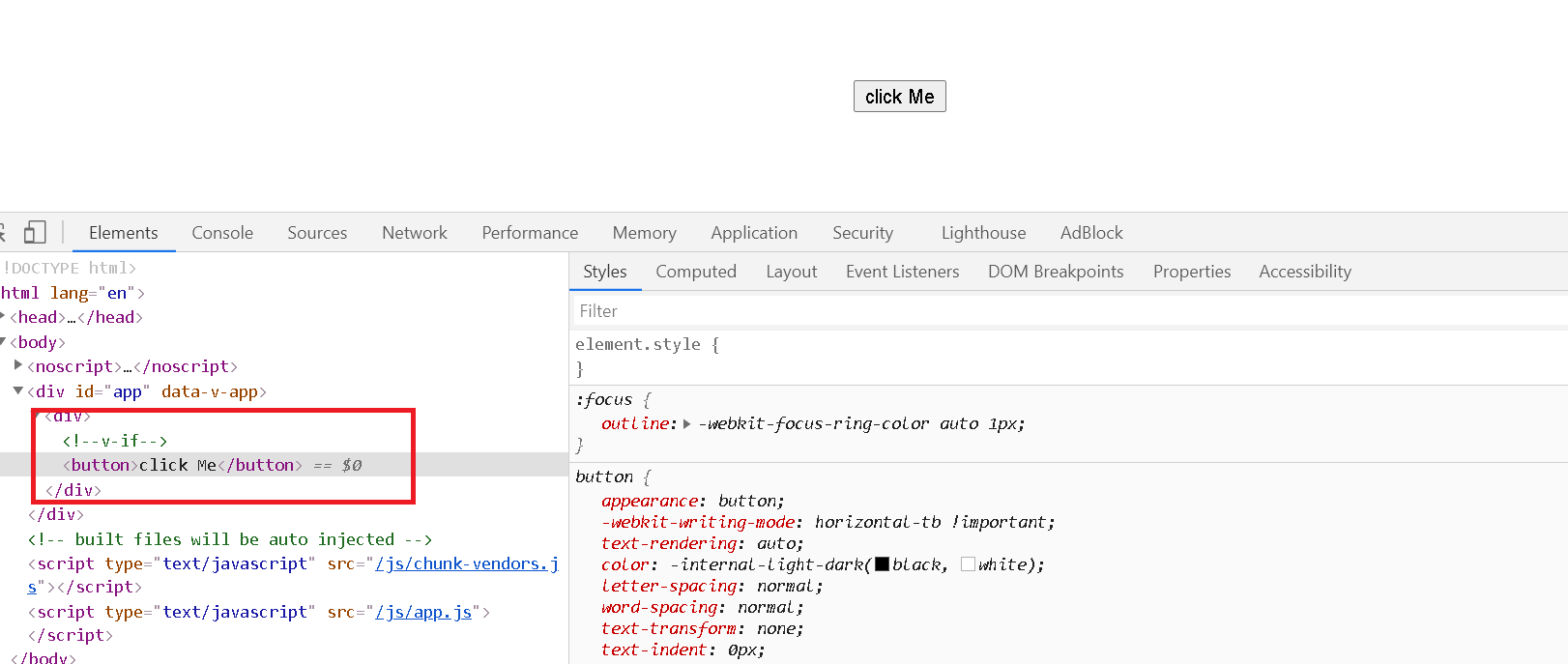
Using HTML :class Binding
Finally, you can control the visibility using HTML and CSS with the :class
binding. In this approach, an object is added to the :class
attribute of the <div>
element.
<div :class="{ hide: !isShow }" id="notShown">Content</div>
Here, the :class
attribute is bound to an object based on the isShow value. If isShow
is true, the hide class will be added to the HTML dynamically.
Here’s the complete code:
<template>
<div>
<div :class="{ hide: !isShow }" id="notShown">Content</div>
<button @click="isShow = !isShow">click Me</button>
</div>
</template>
<script>
export default {
name: "ToggleDiv",
data: function () {
return {
isShow: true,
};
},
props: {
msg: String,
},
};
</script>
<style scoped>
.notShown {
visibility: hidden !important;
}
</style>