Vuejs Input form Number Validation example
This tutorial explains how to add input form validation in the VueJS application
How to add number validation to a form in VueJS
Input forms are fundamental blocks of the web page. The user enters data, the browser displays errors on validation failures, and the form is submitted to the server. These forms contain different input control types of accepting strings or numbers.
In this post, We learn how to input number validation with accepts only numbers from the page in the Vuejs Application. It also includes displaying an error if validation errors exist.
In Vuejs, This can be achieved in multiple ways - using keypress keycode, regular expression, and npm libraries like vue-numeric
You can see the complete code on Github🔗
Other versions available:
First, Let’s get started creating an application. Write a vuejs component.
Create a new VueJS application using the CLI command
First, please check the console for the Vue version command to see if Vue CLI is installed or not.
B:>vue --version
@vue/cli 4.5.9
if it gives a version number, that means Vue is installed. if vue command has not found an error in the console, please install Vue CLI using the below command.
npm install -g @vue/cli
It installs a vue tool on your machine.
Creating a Vue application is easy by using the vue command.
vue create vue-input-example -n
It creates a Vue application, folders, and dependencies, It installs all libraries
Now Application is ready to start running.
First, go to the root folder, run npm run the serve command.
cd vue-input-example
npm run serve
The application is started and running, can access the application at https://localhost:8080
Limit input numbers only and do not accept alphabet characters
This example accepts only numeric only and does not allow other characters.
Let’s create a Component for this example.
- The component name is InputNumber in the src/component folder
Input-type text is declared and added @keypress event
<input id="number" @keypress="validateNumber" />
@keypress is a javascript event, called whenever the key is pressed. validateNumber is an event handler defined in the Methods section of a component. keycodes for the number on the keyboard are 48 to 57. which means the input box is not allowed other key codes. As keyPressed is directly attached to DOM, any changes refresh the page, event.preventDefault enables not to reload the page.
methods: { validateNumber: (event) => { let keyCode = event.keyCode; if (keyCode
< 48 || keyCode > 57) { event.preventDefault(); } }, },
complete code for InputNumber Component:
<template>
<div>
<h3>Vuejs Input Number Validation example</h3>
<input id="number" @keypress="validateNumber" />
</div>
</template>
<script>
export default {
name: "InputNumber",
data() {
return {};
},
methods: {
validateNumber: (event) => {
let keyCode = event.keyCode;
if (keyCode < 48 || keyCode > 57) {
event.preventDefault();
}
},
},
};
</script>
<style scoped></style>
As keyCode is not the correct way to handle it this will be dependent on the key codes of the hardware.
Let’s try using another approach using regular expression.
Using regular expression
In the vue methods, replace keycode logic with regular expression.
regular expression for number is /[0-9]/
Inside the method, get a key character and validate against a regular expression, if it is not passed, do not accept it.
validateNumber: (event) => {
const charCode = String.fromCharCode(event.keyCode);
if (!/[0-9]/.test(charCode)) {
event.preventDefault();
}
};
Input number error on the validation
This example does input form validation for numbers only and displays errors.
declared a model mynumber in the template, to pass from the template to/from the controller return mynumber and isValid with default values in the data function Added @input and @change events to handle input changes. In this event handlers, Check for typed value against a regular expression Finally, display the errors using the v-if directive
<template>
<div class="hello">
<h3>Vuejs Input type number validation</h3>
<br />
<input type="text" placeholder="Enter Number"
v-model="mynumber" @input='change($event)" @change="change($event)" />
<div class="error" v-if="!isValid">Number is Invalid</div
</div>
</template>
<script>
export default {
name: "InputNumberError",
data() {
return {
mynumber: "",
isValid: true,
regex: /[0-9]/
};
},
methods: {
change:function(e){
const number = e.target.value
this.isNumberValid(number);
},
isNumberValid: function(inputNumber) {
this.isValid= this.regex.test(inputNumber)
}
}
};
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
.error{
color:red;
}
</style>
user can see the output in the browser as follows
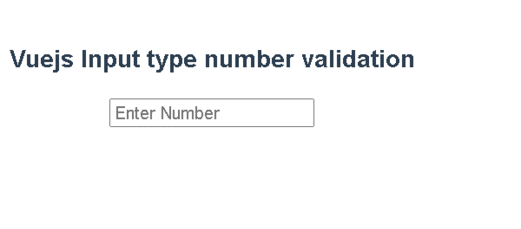
conclusion
It is simple to input form validation for type=url, Even though we can write custom validation for complexform, however, Node provides different frameworks veevalidate
and joi
libraries for simplifying it.