Vue Data function example - Multiple ways to declare
Vue Data function example
Each component has the data
function methods
as part of the declaration.
data is a function in Vue that returns an object.
Why does data have to be declared in a component?
Each component has some data to show in the browser, so the data function
code returns the data. this will be accessed by Component methods globally.
Does the data function hold API logic to retrieve from the Database? No, data fetching logic should be handled in the method section of the component.
When will the data function be called? data function is called by Vue while creating a component instance and returns an object, These data are included in Vuejs reactive and store the instance data as $data object.
Important points about data function
- It can be arrow functions or data property or normal es5 function
- It should always return an object
- Object properties are available, and can be accessed using component instance
- Methods can be called inside data function
- returned object contains properties, keys as well and function calls
Vue.component("About", {
template: "<div>About Component</div>",
data: function () {
return {
faq: "Vuej component faqs",
};
},
});
The following are different ways to declare data functions in Vue components.
- data using ES6 arrow functions
- data object return
- data es5 style functions
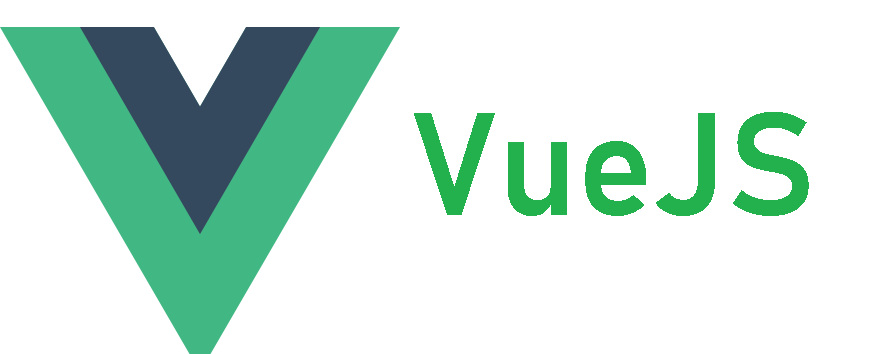
In this blog post, We are going to learn multiple ways to define data function in the vuejs component with examples.
How to declare data arrow functions in vuejs
arrow functions introduced in the ES6 JavaScript language. Vue components can be declared with the data arrow function as seen below.
Arrow functions are a new way of declaring using the()=>
syntax. these functions won’t have this
context inside them. What does mean it? Inside the function, this.HelloWorld props object does not return Component props, **this**
represents the current window
scope, not component scope, This. component throws the below error to the console as these are bound to the parent context.
Uncaught TypeError: Cannot read property ‘InputBlurExample’ of undefined:
export default {
props: ["HelloWorld"],
data: () => ({
name: "Franc",
data: this.HelloWorld, // undefined
}),
};
return keyword returns the object in the data function
The same way can be returned using implicit return syntax
export default {
props: ["HelloWorld"],
data: () => ({
name: "Franc",
data: this.HelloWorld, // undefined
}),
};
How to define data object without function in VueJS
Declared data object rather than a function and initialized with 0 value, property in data are shared by multiple component instances.
what is the issue here? if this component is used in five places, the values are different for each component usage. This is the only drawback.
export default {
props: ["HelloWorld"],
data: {
increment: 0,
},
};
How to define data function object in VueJS
Every component shares a data object, if you declare the data as a function, It is very easy to mutate the state
export default {
props: ['HelloWorld'],
data: function(){
return {increment:0},
}
}
It is the ES5 way of declaring a function in JavaScript.
The above is trimmed with little coding.
export default {
props: ['HelloWorld'],
data: {
return {increment:0},
}
}
export default {
name: "InputBlurExample",
data() {},
methods: {
blurEvent: function (e) {
const email = e.target.value;
console.log(email);
},
},
};
data in vuejs returns the function
data: () => {
return {
stripe: null,
stripePK: stripePK,
...
}
}
How to call methods in the data function in Vuejs
data function returned object contains functions which can instance methods
This will be very useful when you are calling other components event handlers in parent-child components,
export default {
name: "InputComponent",
data() {
var self = this;
return {
onClick: function () {
self.mymethod();
},
};
},
methods: {
mymethod: function () {},
},
};
</script>
Conclusion
In this blog post, You learned multiple approaches to creating a data function and returning an object.
I hope you learned new things from this.