Vue Tailwind Example: Integrating the Tailwind CSS Framework
In this example, we’ll explore how to seamlessly integrate the Tailwind CSS framework into a Vue.js application. The tutorial also covers using Tailwind CSS buttons within Vue components.
How to Integrate Tailwind CSS in a Vue.js Application with Example
- Step 1 Create Vue Application
- Step 2 Start Development Server
- Step 3 Add tailwindcss dependencies
- Step 4 Create tailwind configuration
- Step 5 Create a CSS file and import a CSS file
- Step 4 Add tailwind CSS styles
- Step 5 Add button to Vue component.
- Step 6: Test Vue Application
Create a New Vue application
Open your terminal and run the following command to initiate a new Vue application:
A:\work>vue create vue-css-examples
Vue CLI v4.5.15
? Please pick a preset: Default (Vue 3) ([Vue 3] babel, eslint)
Vue CLI v4.5.15
✨ Creating a project in A:\work\vue-css-examples.
🗃 Initializing git repository...
⚙️ Installing CLI plugins. This might take a while...
added 1274 packages in 2m
21 packages are looking for funding
run `npm fund` for details
🚀 Invoking generators...
📦 Installing additional dependencies...
added 73 packages in 19s
22 packages are looking for funding
run `npm fund` for details
⚓ Running completion hooks...
📄 Generating README.md...
🎉 Successfully created project vue-css-examples.
👉 Get started with the following commands:
$ cd vue-css-examples
$ npm run serve
vue create
command creates the necessary files, folders, and configurations for the Vue application, along with installing dependencies and setting up a live auto-reload and Vue web server.
This command takes a couple of minutes and installs all required dependencies. Once It is done. You should see new brand new Vue application with the folder name - vue-css-examples
Start the development server
Navigate to the newly created application folder
cd vue-css-examples
Then, start the Vue development server with the following command:
npm run serve
This command launches the Vue development server and opens a browser window at localhost:8080
.

Add tailwindcss dependencies
To integrate Tailwind CSS, you need to install the necessary dependencies You can either manually install them Tailwindcss
and peerdependencies
such as postcss
and autoprefixer
. Npm packages required
tailwindcss
: CSS framework with predefined CSS stylespostcss
: Tool to transform preprocessor CSS(sass,less) styles into another CSS files using javascriptautoprefixer
: It is a postcss plugin to add vendor prefix selector.
You can either manually install them
- Install dependencies manually
Following install tailwindcss,postcss7-compact, and autoprefixer dependencies using the below npm command
npm install -D tailwindcss@npm:@tailwindcss/postcss7-compat postcss@^7 autoprefixer@^9
The installation will be done after a couple of minutes.
As a result, It installs and adds the dependencies in the package.json file.
{
"devDependencies": {
"autoprefixer": "^10.4.0",
"postcss": "^8.4.5",
"tailwindcss": "^3.0.2"
}
}
- vue cli using vue-cli-plugin-tailwind
Please run the below command to install all tailwind CSS dependencies
vue add tailwind
We learned two ways to add dependencies, One way is manual, the second way is using the vue CLI command. Finally, ,Second way is Recommended as it simplifies the process and automatically creates the Tailwind configuration
If you use this approach, It also creates a tailwind configuration and you can skip the Add tailwind configuration
section
Add tailwind configuration
This section you can skip this if you add dependencies with the vue add tailwind command
.
You can add tailwind.config.js
with the below command
A:\work\vue-css-examples>npx tailwindcss init -p
Created Tailwind CSS config file: tailwind.config.js
Created PostCSS config file: postcss.config.js
The following files are created in the application root folder.
- tailwind.config.js
- postcss.config.js
tailwind.config.js
contains the following entries.
module.exports = {
content: [],
theme: {
extend: {},
},
plugins: [],
};
In tailwind.config.js, Update the below things.
- change from purge:[]
- to purge:
['./src/**/*.{js,jsx,ts,tsx}', './public/index.html']
,
module.exports = {
purge: ["./src/**/*.{js,jsx, ts,tsx}", "./index.html"],
content: [],
theme: {
extend: {},
},
plugins: [],
};
Create a CSS file and import in component
Create a css
folder within the src directory, and inside it, create a new file named tailwind.css
. Include the following content in tailwind.css
:
Following is the directory structure.
src/
├── CSS/
├── tailwind.css
├── app.js
└── index.js
In tailwind.css, please include tailwind CSS styles
@tailwind base;
@tailwind components;
@tailwind utilities;
Next, please import tailwind.css in main.js
import { createApp } from 'vue';
import App from './App.vue';
import './css/tailwind.css';
createApp(App).mount('#app');
Running npm run serve
will generate output.css
in the src/css
folder.
Add button to Vue component
In the Vue component HelloWorld.js
- Please import tailwind.css into this component
- update the component to include tailwind button HTML and tailwind CSS styles.
<template>
<h1 className="text-3xl font-bold underline">
Simple Tailwind CSS application
</h1>
<button class="bg-transparent hover:bg-blue-500 text-blue-700 font-semibold hover:text-white py-2 px-4 border border-blue-500 hover:border-transparent rounded">
Save
</button>
<span>
<button class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 border border-blue-700 rounded">
Submit
</button>
</span>
</template>
Run npm run serve command and You will see the output on the browser page as follows.
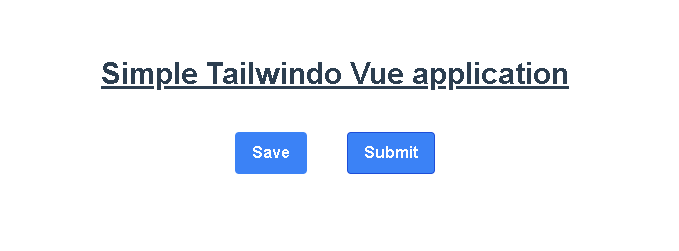
PostCSS plugin tailwindcss requires PostCSS 8 error in the Vue application
I got the below error during the integration of tailwindcss to the existing vuejs application.
./src/css/tailwind.css (./node_modules/css-loader/dist/cjs.js??ref--7-oneOf-3-1!./node_modules/postcss-loader/src??ref--7-oneOf-3-2!./src/css/tailwind.css)
Module build failed (from ./node_modules/postcss-loader/src/index.js):
Error: PostCSS plugin tailwindcss requires PostCSS 8.
Migration guide for end-users:
https://github.com/postcss/postcss/wiki/PostCSS-8-for-end-users
at Processor.normalize (A:\work\vue-css-examples\node_modules\postcss-loader\node_modules\postcss\lib\processor.js:153:15)
at new Processor (A:\work\vue-css-examples\node_modules\postcss-loader\node_modules\postcss\lib\processor.js:56:25)
at postcss (A:\work\vue-css-examples\node_modules\postcss-loader\node_modules\postcss\lib\postcss.js:55:10)
at A:\work\vue-css-examples\node_modules\postcss-loader\src\index.js:140:12
You can check code [Source code](https://github.com/intkiran/vue-css-examples)
This is an issue with tailwind CSS and its dependencies configuration. Solution:
First, Uninstall dependencies using npm uninstall tailwindcss postcss autoprefixer
. command as seen below.
A:\work\vue-css-examples>npm uninstall tailwindcss postcss autoprefixer
removed 50 packages, and audited 1469 packages in 8s
22 packages are looking for funding
run `npm fund` for details
30 vulnerabilities (19 moderate, 11 high)
To address issues that do not require attention, run:
npm audit fix
To address all issues (including breaking changes), run:
npm audit fix --force
Run `npm audit for details.
Next, Add the tailwind CSS dependencies with vue-cli-plugin-tailwind
. The vue-cli-plugin-tailwind
plugin is installed and configured with the vue add tailwind
command.
A:\work\vue-css-examples>vue add tailwind
WARN There are uncommitted changes in the current repository. it's recommended to commit or stash them first.
? Still, proceed? Yes
📦 Installing vue-cli-plugin-tailwind...
added 1 package in 8s
22 packages are looking for funding
run `npm fund` for details
✔ Successfully installed plugin: vue-cli-plugin-tailwind
? Generate tailwind.config.js full? tailwind.config.js already exists! Do you want to replace it? Yes
🚀 Invoking generator for vue-cli-plugin-tailwind...
📦 Installing additional dependencies...
added 103 packages, removed 126 packages, and changed 1 package in 19s
22 packages are looking for funding
run `npm fund` for details
⚓ Running completion hooks...
✔ Successfully invoked generator for a plugin: vue-cli-plugin-tailwind
Conclusion
This step-by-step guide demonstrates creating a Vue application and seamlessly integrating the Tailwind CSS framework.