Learn Service Worker for application offline access
- Admin
- Dec 31, 2023
- Javascript
Why service worker introduced?
In Mobile Applications, features like offline functionality, running background services, and push notifications are implemented as part of native mobile devices. It does only be available on mobile as inbuilt functionality.
Desktop applications and browser-based applications are not available in the builtin.
These functionalities in a web application are available using a service worker. The web page is normally a user-initiated request which is handled by the main thread.
A service worker is a separate thread that runs in the background to track network requests, cache the data, retrieve it, and push the message to the browser.
This worker provides real-time content or cached content based on network availability. It provides offline access to the application to make it a progressive web application.
Service worker browser features
- It acts like a proxy server between web applications, browsers, and network services.
- This can access the Page-level DOM elements.
- This makes use of javascript promises that runs without waiting for a request
- This runs only on the HTTPS protocol
- Service worker is a javascript code that runs in the background without user intervention.
- Works on HTTPS protocol for security reasons
Service worker Lifecycle events
There are various events like register/execution/cache/fetch for providing offline access.
Registration First Services workers need to register. Once the service worker is registered, the Service worker code is copied to the client, and moved to the next lifecycle event for installation browser will start this in a background thread.
<script>
// Register the service worker
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('./service-worker.js').then(function(registration) {
// Registration was successful
console.log('ServiceWorker registration successful with scope: ', registration.scope);
}).catch(function(err) {
// registration failed :(
console.log('ServiceWorker registration failed: ', err);
});
} else{
console.log('Service worker support is not available in a browser')}
</script>
It installs service worker and specific javascript file code. It checks if a service worker is available in the browser, and registers the service worker code in worker.js once the page loads Register() returns the promise, will be registered if the services worker is not registered. else update it. Service worker script code you have to write the code for installation/activate/fetch events.
self.addEventListener("install", function (e) {});
self.addEventListener("activate", function (e) {});
self.addEventListener("fetch", function (e) {});
Install Event:- This has code for storing data to a storage mechanism and allows to save assets for offline access. Activate Event: this will get notified once the service worker installation is completed. write a code for removing the older version of caches Fetch Event: This will be called for any resource of service worker to fetch resources like calling ajax API calls.
Service worker API provides fetch -retrieve the content from the cache and
Cache - storing application-specific data to the storage mechanism
script example for offline access:-
It is a sample code of the angular application for offline access.
var dataCacheName = "mycache-v1";
var cacheName = "mycache-1";
var filesToCache = ["/", "/index.html"];
console.log("ServiceWorker Start");
self.addEventListener("install", function (e) {
console.log("[ServiceWorker] Install");
e.waitUntil(
caches.open(cacheName).then(function (cache) {
console.log("[ServiceWorker] Caching app shell");
return cache.addAll(filesToCache);
}),
);
});
self.addEventListener("activate", function (e) {
console.log("[ServiceWorker] Activate");
e.waitUntil(
caches.keys().then(function (keyList) {
return Promise.all(
keyList.map(function (key) {
if (key !== cacheName && key !== dataCacheName) {
console.log("[ServiceWorker] Removing old cache", key);
return caches.delete(key);
}
}),
);
}),
);
return self.clients.claim();
});
self.addEventListener("fetch", function (e) {
console.log("[Service Worker] Fetch", e.request.url);
e.respondWith(
caches.match(e.request).then(function (response) {
return response || fetch(e.request);
}),
);
});
console.log("ServiceWorker End");
You can check the service worker status in chrome using the URL chrome://serviceworker-internals. You can see debugging Service worker data in chrome as per the below screenshot.
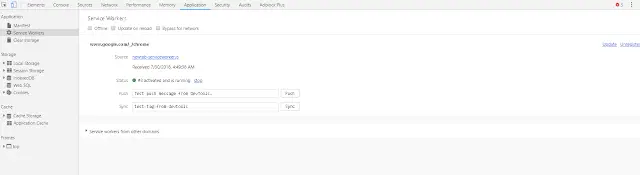
Browser support
Supported by Chrome, Firefox, and Opera. Microsoft Edge has recently introduced supporting this. Apple Safari has plans to support this in the future.
Service workers by default are enabled in browsers. You can also check settings in different browsers as follows.
Firefox Browser Open about:config in URL in Firefox, Check dom.serviceWorkers.enabled value, if it is false, change it to true.
Chrome Browser Open chrome://flags in URL in chrome, Check experimental-web-platform-features value, if it is disabled, Enabled it.
Opera Browser Open Opera://flags in URL in opera, Check Support for ServiceWorker value, if it is false, change it to true. Microsoft Edge Browser Open about:__flags in url in Edge, Check Enable service workers to value, if it is not checked, Checked it.
Once you made changes, please restart the browser.
Browser tools
These tools are helpful for a developer for debugging scripts.
Chrome developer tools have the following things.
chrome://inspect/#service-workers - current service worker and storage device information
chrome://serviceworker-internals - this allows to start/stop/debug the services works
Firefox has the following developer tools.
about: debugging - This shows a list of registered services workers, You can disable/remove it from here
Use cases:-
Offline application access where network bandwidth is limited
Push notifications
GPS support in future
Fix for DOMException: Failed to register a ServiceWorker
During testing the service worker code, you used to get the below errors.
Uncaught (in promise) DOMException: Failed to register a ServiceWorker:
this is an issue with the javascript code located in the js file. To debug further on this.
go to chrome://serviceworker-internals and open developer tools pages, Debug the worked script file code to see where exactly the issue is coming from by inspecting it.
Failed to register a ServiceWorker: A bad HTTP response code (404) was received when fetching the script.
Usually, the main HTML page like index.html has a service worker code to register it. You get this error on this page only. Here you are configuring the javascript file.
Please make sure that you are referring to the exact path of the service worker file. or place both files in the same directory.