How to Create React Alert Notification with Example| React toastify
In these tutorials, You’ll learn how to implement alert notifications with the react-toastify npm library
.
What is toastify in javascript?
toastify
is a javascript notification library, used to display notifications or alerts to users about the status of an application action.
In Operation System, Notifications are displayed with animated notifications to the user about the status of the user action result, The same concept is implemented in UI Pages in Browser. It is easy and quick to integrate into any application and loading is quick as the size is less. It provides the following features
- Display different types of alert notification messages in applications
- Style toast component styles
- Configuration settings for display position
- It can be used in node applications such as react, vue, and angular framework
- RTL integrated
- Control notifications programmatically as well as configuration
the react-toastify library used in reactJS components and applications.
What is react-Toastify used for?
react-Toastify
is a popular npm library for displaying alerts and toast notifications in an application. It is easy to install and configure the application. You can style the messages, and display message types such as success, and error messages. It reduces a lot of code if we want to write toast message notifications without this library. You can configure timeout which is a valid time to display a notification, once the timeout is expired, the notification is automatically closed.
You can also configure customization such as left, top, and right bottom for the display of notification popups. This npm library has many features included.
- Opensource and easy to configure and customize
- Programmatically control notifications
- Customize the styles and display locations like a top, left, and bottom
- progress bar included
- Package size is less.
- Promise integration
- also integrated with progress notifications
- animations support
What are toast messages in React?
react toast alias alert notifications are used to display alert notifications with user-customized message.react-toastify is a popular toast/alert notification for React projects.
Some examples are
- success message such as a record is added.
- error messages such as duplicate record found warning and informational messages.
Other versions available:
What is a toastcontainer?
toast container is a global object and placeholder to show the notification message to be shown on react component. It is added to the main parent component to load into DOM Tree to make toast notification works in a child component.
It contains default configurations such as position,closeButton, etc
<ToastContainer closeButton="{false}" position="bottom-right" />
How do you use Toastify in react?
Following are steps to use toastify in react
- install
react-toastify
npm library - Create a new component in the src folder
- add
react-toastify
CSS in a react component - Configure
ToastContainer
in an application - Add action such as button click to show Toast notifications
react toastify notification example
Let’s integrate notifications in React application using react application.
- Create react application, add react-toastify npm library to component
First, Let’s create a new react application using the create-react-app
command.
npx create-react-app react-toast-tutorial
This creates a react-toast-tutorial project and creates all required files to run a project, and installs dependencies.
Next, Go to the application root folder
cd react-toast-tutorial
and run the npm run start
command.
npm run start
This starts the application in the browser and can access with localhost:3000
Go to the application folder, and run the below command to install the react-toastify npm dependency
npm install --save react-toastify
This updates package.json
and installs the dependency to the node_modules
folder in the application
{
"dependencies": {
"react": "18.2.0",
"react-dom": "18.0.0",
"react-toastify": "^9.0.8"
}
}
Secondly, add the required CSS files to the application.
Adding ReactToastify.css
to an existing application using import react-toastify CSS
into react component
It provides CSS styles that you need to import into component
import "react-toastify/dist/ReactToastify.css";
Another way to import in sass code using the @import
feature
@import '~react-toastify/dist/ReactToastify.min.css';
- Add ToastContainer one time in the global component
ToastContainer
and toast
are two classes imported into your app to display a toast notification.
import { toast, ToastContainer } from "react-toastify";
ToastContainer
is a container for an application in which you need to include a root component. This must be loaded into the DOM tree. However, It is not required to add it to every component,
<ToastContainer closeButton={false} position="bottom-right" />
toast configure method:
toast.configure()
has to call in the Root component and it is a one-time process
toast.configure();
This can configure the configuration object which is an object of configuration
This can be used to configure how to toast popup can be customized
toast.configure({
autoClose: 10000,
draggable: true,
});
Next, the popup can be displayed using the toast object
Toast.success/error/info/warn/dark/default(message,optionalobject)
An example is to configure the success popup displayed in the top right position
toast.success("Success message", {
position: "top-right",
pauseOnHover: true,
draggable: false,
progress: undefined,
});
an optional object contains the below parameters
position
values top-left,top-right,top-center, bottom-left,bottom-right,bottom-centerdraggable
popup is draggable or not, true/falseprogress
is to configure the progress bar
How to Dismiss toast programmatically:
toast.dismiss()
is to close the toast using code.
Basic toast notification example
In the Root component, import and configure ToastContainer in the application root
App.js code:
import React from "react";
import { toast, ToastContainer } from "react-toastify";
import "react-toastify/dist/ReactToastify.css";
import BasicToast from "./components/BasicToast";
export default function App() {
return (
<div>
<h1>Hello StackBlitz!</h1>
<BasicToast />
<ToastContainer closeButton={false} position="bottom-right" />
</div>
);
}
let’s create a toast component for example
import React, { Component } from "react";
import { render } from "react-dom";
import Hello from "./Hello";
import { toast, Zoom } from "react-toastify";
import "react-toastify/dist/ReactToastify.css";
class BasicToast extends Component {
constructor() {
super();
}
showError = () => {
toast.error("ERROR Notification");
};
showSuccess = () => {
toast.success("Success Notification");
};
showInfo = () => {
toast.info("Info Notification");
};
showWarn = () => {
toast.warn("Warn Notification");
};
render() {
return (
<div>
<div>
<span>
<button onClick={this.showError}>Error </button>
</span>
<span>
<button onClick={this.showSuccess}>Success </button>
</span>
<span>
<button onClick={this.showInfo}>Info </button>
</span>
<span>
<button onClick={this.showWarn}>Warn </button>
</span>
</div>
</div>
);
}
}
export default BasicToast;
Now, Run the project and render the output :
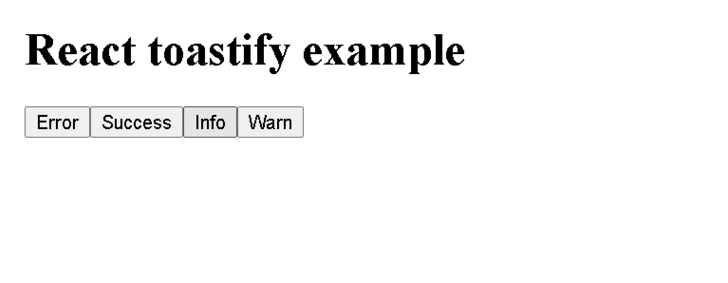
React alert notifications Promise for API result
Assume, call API is an async method that returns the response from API for success result, error response for an API error.
In promise then method,
- toast.dismiss closes the toast if already displayed in the browser
- calling toast.error for error result
- toast.success for success result
callAPI().then((response) => {
if (response.error) {
toast.dismiss();
toast.error("ERROR while calling API");
} else {
toast.dismiss();
toast.success("API SUCCESS Done");
}
});
How to update react-toastify in React Application?
npm command is used to install the latest react-toastify npm library. It is quick and easy to upgrade to the latest version of in react app.
For npm users,
npm install react-toastify@latest
you can specify a version
npm install react-toastify@8.0.0
For yarn users,
yarn add react-toastify
yarn add react-toastify@8.0.0
It adds dependency in the package.json file
"dependencies": {
"react": "18.2.0",
"react-dom": "18.0.0",
"react-toastify": "^9.0.8"
}
React toastify component is not rendered in a component
Sometimes, toast messages are not rendered correctly
Let’s see the solution to this.
First, make sure that configure() method is declared in react component
toast.configure();
Next, add <ToastContainer />
and load this in the Root component to load in the DOM tree.
Check CSS id is imported correctly in a component as given below
import "react-toastify/dist/ReactToastify.min.css";
if you are using scss, import the below line
@import '~react-toastify/dist/ReactToastify.min.css';
Conclusion
This post talks about the basic toast component.