Reactjs Fix Failed form propType: You provided a `value` prop to a form field and an `onChange` handler
If you are learning to react and working on applications, we used to get the following errors.
Initially, I got the following errors while learning reacts framework.
This blog post talks about the solution for Failed form propType errors in input controls.
- Failed form propType: You provided a
value
prop to a form field without anonChange
handler - Failed form propType: You provided a
checked
prop to a form field without anonChange
handler
This error comes in the following cases.
the input form declared with initializing value attribute without onchange
Handler
Like HTML form handling, React also supports form input control handling with two approaches.
value
prop to a form field and an onChange
handler
Fix for form propType: You provided a Let’s see how this error comes with react component.
Created a simple react component with input type=text”.
import React, { Component } from "react";
class TestComponent extends Component {
render() {
return (
<div>
<input type="text" value="Test value"></input>
</div>
);
}
}
export default TestComponent;
And you will see the error on the console
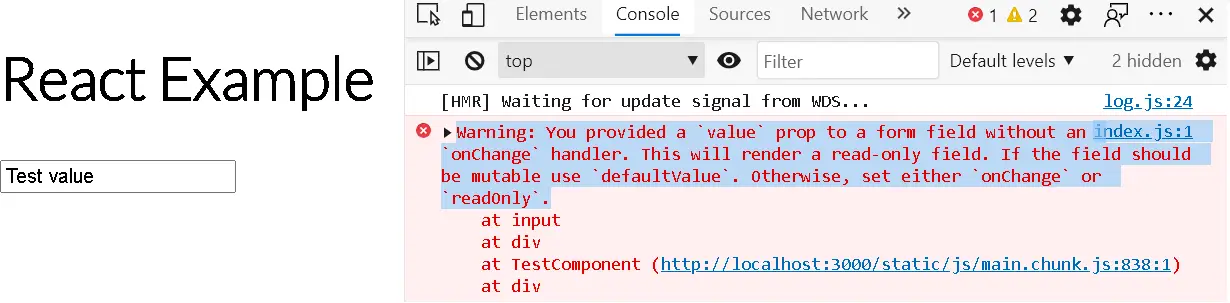
Another way, Created another component with input type=checkbox
, able to reproduce the issue.
<input type="checkbox" checked="true" ></input>
you got the below error in the console
Warning: You provided a checked
prop to a form field without an onChange
handler. It will render a read-only field. If the field should be mutable use defaultChecked
. Otherwise, set either onChange
or readOnly
.
You are setting input values initially using a value attribute, So you can use a placeholder for it.
checked
prop to a form field without an onChange
handler
Solution and Fix for You provided a UI form has its start and react has a separate state object which holds form data.
In React, Form handling is done using the below approaches
Handling state by React
Controlled Component🔗 In this, form input is controlled by React component itself using state object with the useState
method
This error is thrown in the console in the below cases Missing required attributes and directives in Controlled and uncontrolled components
Then, What are the required things for controlled components?
Value
for input elements and checked
for radio and checkbox attribute is updated by React component from state object while user typing or selecting the input control.
ok, How and to whom do you update the state?
State object in react is updated using setState() method
, and onChange
directive is fired while keystroke is entered in input, so you will update state in onChange
event handler
In the below form handling is done by React component, User entered data on the input-form, onChange event fired and handler is executed, get the value and update the state using setState
object
import React, { Component } from "react";
class TestComponent extends Component {
constructor(props) {
super(props);
this.state = {
name: "",
};
}
onChangeEvent = (event) => {
const newName = event.target.value;
this.setState({
name: newName,
});
};
render() {
const { name } = this.state;
return (
<div>
<input
type="text"
placeholder="Enter Name..."
value={name}
onChange={(event) => this.onChangeEvent(event)}
/>
<div>{name}</div>
</div>
);
}
}
export default TestComponent;
In the same way for checkboxes, We will use the onChange event handler to update the state which bound to the checked value.
Form handling with DOM
In this, the input form data is handled by the Inbuilt DOM itself. This is called Uncontrolled Components🔗
So errors, when you don’t specify the following attributes
defaultValue for input text controls defaultChecked for radio and checkbox
These attributes are used for configuring default values while showing the form to the user. Then, How does DOM update the data?
Here DOM handles the data, refs in react
are used to retrieve the values from DOM.
Here is the complete code and explanation
- Defined input bounded to submit handler which is bounded in constructor
- Added ref to input element which initialized
React.createRef()
in constructor - Input form is initialized with defaultValue for input type=text/textarea/select
- For checkbox and radio types, use defaultChecked
- In the submit event handler, input values is retrieved using
this.input.current.value
import React, { Component } from "react";
class TestComponent extends Component {
constructor(props) {
super(props);
this.onSubmitEvent = this.onSubmitEvent.bind(this);
this.input = React.createRef();
}
onSubmitEvent = (event) => {
event.preventDefault();
console.log(this.input.current.value);
};
render() {
return (
<form onSubmit={this.onSubmitEvent}>
<label>
Name:
<input defaultValue="Franc" type="text" ref={this.input} />
</label>
<input type="submit" value="Submit" />
</form>
);
}
}
export default TestComponent;
Conclusion
In this tutorial, we will learn how to fix Fix for form propType error in reacting with controlled and uncontrolled components for example.