ReactJS - How to add google fonts library example
In this post, How to integrate google fonts library in Reactjs application with examples
. This covers a *multiple approaches to integrating google font lato into reactjs projects.
Other versions available:
React application from scratch
The create-react-app
command creates new applications with all files and builds pipelines. It provides all the latest reactjs and their dependencies.
npx create-react-app react-google-fonts
Success! Created react-google-fonts at B:\blog\jswork\react-google-fonts
Inside that directory, you can run several commands:
yarn start
Starts the development server.
yarn build
Bundles the app into static files for production.
yarn test
Starts the test runner.
yarn eject
Removes this tool and copies build dependencies, configuration files
, and scripts into the app directory. If you do this, you canβt go back!
We suggest that you begin by typing:
cd react-google-fonts
yarn start
Happy hacking!
This will create a react application react-google-fonts
, with all required files and project structure, and install dependencies to start running.
Go to the application root folder, start the server using npm start
cd react-google-fonts
npm start
[email protected] start B:\blog\jswork\react-google-fonts
react-scripts start
Compiled successfully!
You can now view react-google-fonts in the browser.
Local: http://localhost:3002
React application running with localhost:3002
adding Google library CDN in React application
Go to google libraryπ link and select the font you want to integrate into react application. Lato font is selected and given the following url
https://fonts.googleapis.com/css2?family=Lato:wght@300&display=swap
Following are ways to add google font library without hosting in the application.
@import google fonts
@import CSS is used to import external CSS libraries into current styles. It can be used in Global styles(app.css) or specific component stylesheets. Go to App.css
in the src folder. Add the following entries to enable Lado font in React applications
@import url("https://fonts.googleapis.com/css2?family=Lato:wght@300&display=swap");
html,
body {
font-family: "Lato", sans-serif;
}
link google fonts
Another approach is to add a link
tag in the head section of public/index.html
<html>
<head>
<link rel="preconnect" href="https://fonts.gstatic.com" />
<link
href="https://fonts.googleapis.com/css2?family=Lato:wght@300&display=swap"
rel="stylesheet"
/>
</head>
<body></body>
</html>
Complete React component code
import "./App.CSS";
function App() {
return (
<div className="App">
React Google fonts library integration example
<p>This is testing google fonts LATO in React application</p>
</div>
);
}
export default App;
CSS styles contain rules for font-family: Lato, sans-serif;
configured in HTML and body.
html,
body {
font-family: "Lato", sans-serif;
}
App {
font-size: 24px;
}
p {
font-size: 14px;
}
And output you see in the browser is
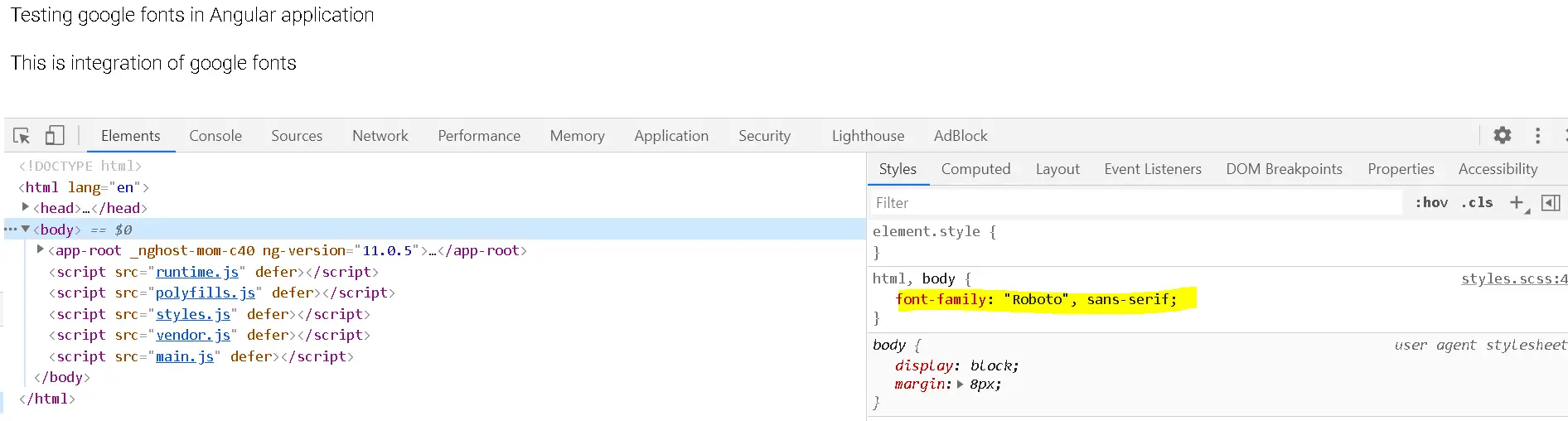
hosting fonts with the font-face declaration
In this approach, CSS font libraries are copied to react application. You will see how to add google fonts to stylesheets in react application.
- Go to google font Latoπ
- Click on the
Download family
button, It will download the Lato font family zip file locally - Extract the zip file and copy it into the
src/fonts
folder.
You can see the project folder structure as well as fonts folder files
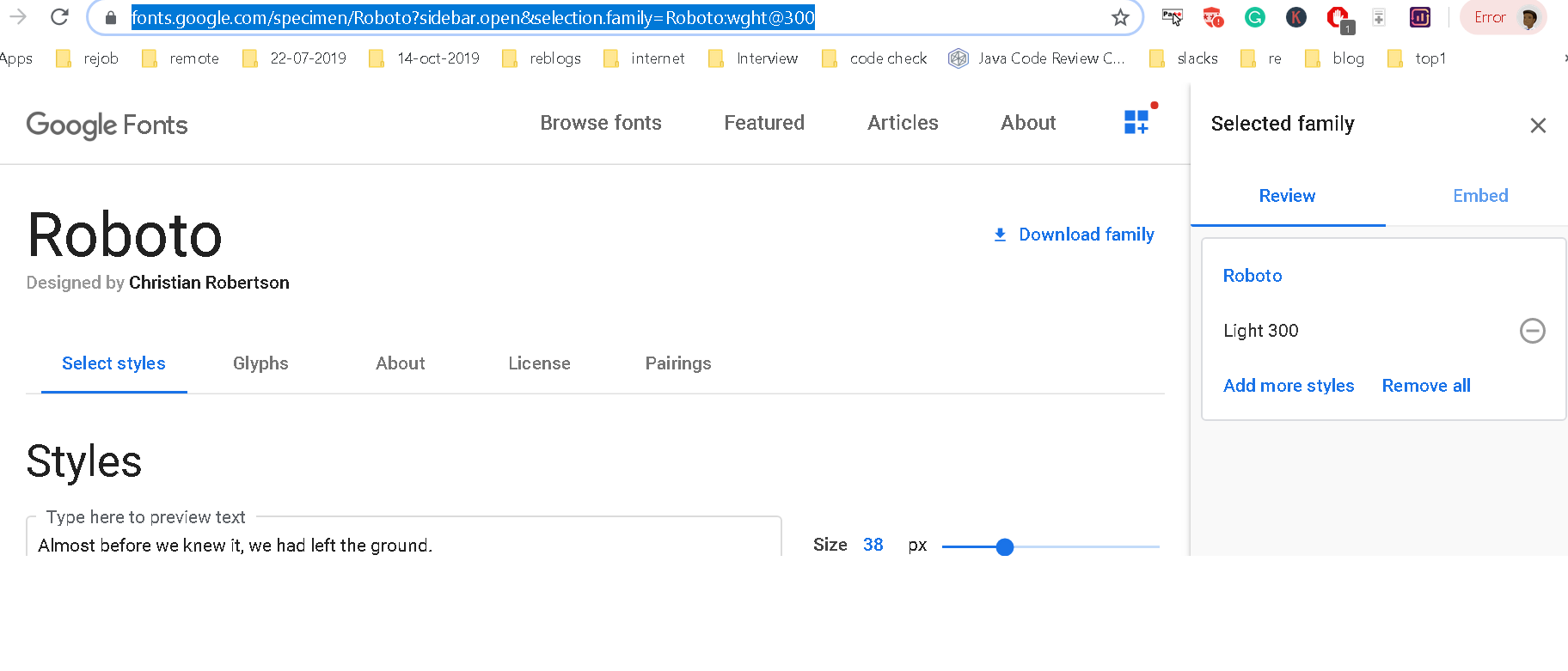
In the index.css file of react project, add Lato font-face declaration to enable google fonts in React application.
@font-face {
font-family: "Lato";
src:
local("Lato"),
url("./fonts/roboto.ttf") format("truetype");
}
CSS properties are configured as follows
heading {
font-family: "Lato", sans-serif;
}
Conclusion
In this tutorial, you learned different approaches to integrating the google font library in the Reactjs app
- @import CSS feature
- link attribute
- hosting CSS files using @font-face declaration
Importantly, the First and second approaches are simple ways of adding the google font library in react application, However, performance is not good as it is pointed to external dependencies.
Finally, host CSS files are good in terms of performance, less dependent, and maintainability.