How to add comments to render jsx in React
Comments are useful pieces of text in the code about lines of code and are ignored by the interpreter of an application. These will not be displayed by the user.
React is a UI framework with reusable components.
Components can be written using JSX or TSX language and CSS
Comments in React JSX
JSX is a special syntax with uses a mix of JavaScript and HTML. The render method is written with JSX syntax. However, HTML syntax and javascript syntax will not work.
Javascript comments can be used in constructor or callback methods(componentDidMount etc.) or any function or method.
if JSX uses javascript comments, It will be displayed on the browser.
/This is multi comments text/ // Single line comments First component
import React, { Component } from "react";
import PropTypes from "prop-types";
class First extends Component {
constructor(props) {
super(props);
//constructor comments
}
componentWillMount() {}
componentDidMount() {
//constructor comments
}
componentWillReceiveProps(nextProps) {}
shouldComponentUpdate(nextProps, nextState) {}
componentWillUpdate(nextProps, nextState) {}
componentDidUpdate(prevProps, prevState) {}
componentWillUnmount() {}
render() {
return (
<div>
/* This is multi comments text */ // Single line comments First
component
</div>
);
}
}
First.propTypes = {};
export default First;
Displayed on browser:
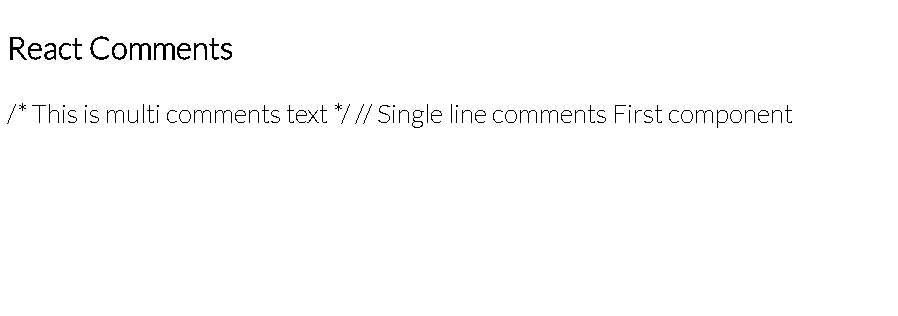
So there is a special syntax for adding comments to jsx
Syntax:
{
// javascript syntx expression
/** this is multi line comments ofsyntax
}
or
{
// this is a single line of syntax
}
That’s how javascript comments wrapper inside {}
.
Important points jsx comments in react application
- JavaScript comments are wrapped inside a parenthesis
{}
. - Single line comments can be included with closing parenthesis
}
in a new line - comments not displayed on browser page as well as source code
- special syntax
{}
applied the code inside a single element of return of render method - In the render method outside element, you can use normal javascript syntax style
You can check the comments usage example below
render() {
/* multi line valid comments */
// single line valid comments
return (
/* multi line valid comments */
// single line valid comments
<div>
{/* This is multi comments special syntax
line2
*/}
{// Single line special syntax comments
}
First component
</div>
);
}
}
Invalid comments in jsx:
Below are invalid comments declarations.
render() {
return (
<div>
// single line invalid comments
/* multi line invalid comments
{// Single line invalid comments}
First component
</div>
);
}
This will throw a react error by the compiler - Unexpected token, expected }
Wrapped up
Comments can also be included in react JSX with special syntax.