react helmet tutorials with examples
React Helmet is an npm library that provides react components to change and update tags values inside the head tag of an HTML in react pages.
Head is a parent tag for a title, meta, script, link, noscript, and style tag, So you can change or set this tag’s values dynamically.
This tutorial covers a basic react-helmet package, how to change document head values dynamically, and How it is useful in SEO during server-side rendering.
Why it is required and what are the advantages?
In Index.html, you can set basic head child as you see below.
public/index.html
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<meta name="theme-color" content="#000000" />
<meta name="description" content="react descript" />
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<title>React title</title>
</head>
It is the main component for your react pages. Any component you created rendered as a child component of the main component ie App.js.
you can override parent components with child components values dynamically using the helmet component.
It helps to override and update dynamically the child elements inside a header tag. It is very useful to have a clean SEO for crawlers about each page in Single page applications.
Some important features
- Can be changed all tags - title, meta, script, link,noscript, and style tag
- It is easily integrated into server-side and client-side rendering
Installation
First, install the library with npm or yarn command to the existing react application.
yarn add react-helmet
npm install react-helmet
Changing HTML head Children using the react-helmet example
The helmet is a component that can dynamically change document head attributes values dynamically
import React, { Component } from "react";
import { Helmet } from "react-helmet";
class AddingScriptExample extends Component {
render() {
return (
<div className="application">
<Helmet>
<base />
<title>React helmet example</title>
<meta
name="description"
content="React helment is useful for seo for dynamically changing head information"
/>
<link rel="canonical" href="somelink" />
<script type="text/javascript">
alert('react component script');
</script>
</Helmet>
...
</div>
);
}
}
export default AddingScriptExample;
You can inspect these meta tags on view source option of your webpage.
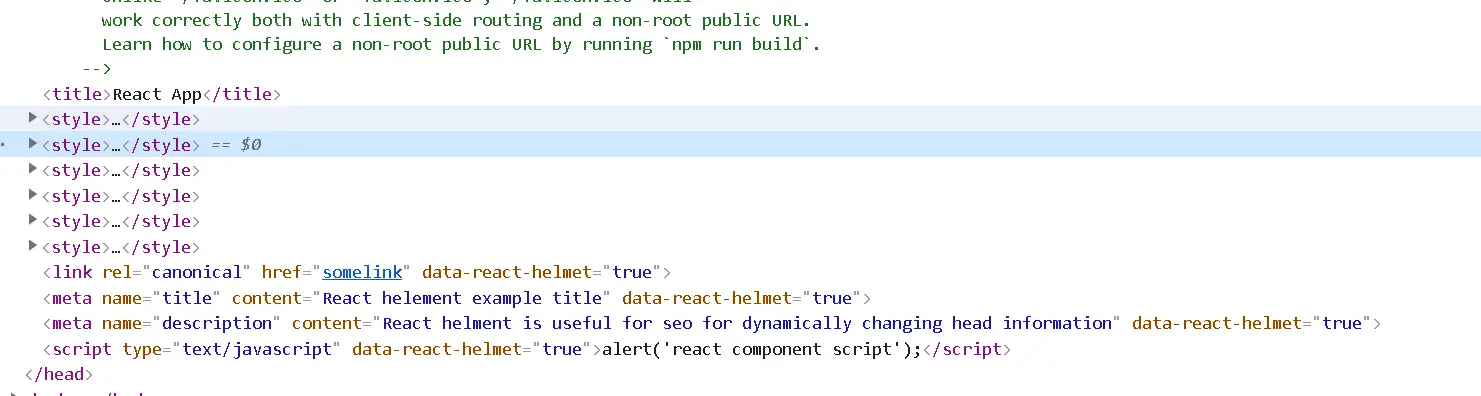
Following are the attributes that can be changed dynamically with the helmet component.
base - the base path of the page meta - metadata information about a webpage, these can have attribute types robots
, description
, keywords
. link - Adding external styles script -This can add the inline script as well as external javascript files.
Note: if there is any issue with overriding tag values dynamically, try to add data-react-helmet=true to tags inside the helmet component.
How to load an inline and external Script tag
if you want to add custom JavaScript to react component, You can add the following line of code.
script tag with type=“text/javascript” to be defined inside helmet component
<helmet>
<script type="text/javascript">alert('react component script');</script>
</helmet>
Adding script custom URLs, we can do with script url syntax as discussed [here]
How do you document the title in react component
We can use PureComponent instead of Component as there are no state or props changes required and do a shallow comparison of scalar values and object references, Thus improving performance on loading components.
import React from "react";
import { Helmet } from "react-helmet";
class DocumentTitle extends React.PureComponent {
render() {
return (
<>
<Helmet>
<title>About us</title>
</Helmet>
</>
);
}
}
export default DocumentTitle;
react-helmet-async example
The helmet component is synchronous and works perfectly on client-side rendering,
It causes issues on the server side while streaming components with ignoring react side effects.
react-helmet-async requires the provider to capture the helmet component state of the DOM tree in react application.
Async usage is the same as like helmet component except by adding a component inside the HelmetProvider
component.
You can also use renderToString
in Server side rendering for better usage.
import Helmet, { HelmetProvider } from "react-helmet-async";
class HelmentAsyncExample extends Component {
render() {
return (
<HelmetProvider>
<div>
<Helmet>
<title>React Helmet Asyc example</title>
<link rel="canonical" href="https://www.url.com/" />
<meta keywords=" seo keywords" />
<meta description=" website description" />
</Helmet>
</div>
</HelmetProvider>
);
}
}
export default HelmentAsyncExample;
server-side rendering example
On the server-side, Call Helmet.renderStatic() method to get document header tags values during prerender process.
Here is the component example code:
import React from "react";
import { renderToString } from "react-dom/server";
import express from "express";
import App from "./src/App";
import { Helmet } from "react-helmet";
const expressServer = express();
expressServer.get("/*", (req, res) => {
const appRender = renderToString(<App />);
const helmet = Helmet.renderStatic();
res.send(stringHtml(appRender, helmet));
});
expressServer.listen(4000);
function stringHtml(appStr, helmet) {
return `
<!doctype html>
<html ${helmet.htmlAttributes.toString()}>
<head>
${helmet.title.toString()}
${helmet.meta.toString()}
${helmet.link.toString()}
</head>
<body ${helmet.bodyAttributes.toString()}>
<div id="container">
// react component code can be added
</div>
</body>
</html>
`;
}
Advantages
- Added the tags dynamically in single-page applications and improves Search engine optimization of each page
- Helps crawlers and search engine bots understand the page
- If there are multiple meta tags with different values in react tree components, the child component overrides the parent