How to sum an array of objects with numbers in react
- Admin
- Dec 31, 2023
- Typescript Reactjs
It is a short tutorial on how to get a sum of numbers in React application. It also includes how to calculate sum or numeric properties of an object array with example.
Let’s consider the array of objects as given below.
[
{
"firstName": "John",
"lastName": "Erwin",
"email": "[email protected]",
"salary": 5000
},
{
"firstName": "Ram",
"lastName": "Kumar",
"email": "[email protected]",
"salary": 5000
}
]
An array contains objects with different employee properties.
Let’s see an example of how to print an array of objects into an HTML table, and also calculate the sum of object numeric values and display it in React component.
- Created a React Component
NumberSum
- Created an array of objects and stored the array in react state
- In Render method construct HTML table with
table
thead
,tbody
andtfoot
. - Iterate an array of objects using the array
map
method and construct a string with alltr
andtd
of rows and columns. - Sum of numbers using array
reduce
method which has accumulator initialized to 0 initially and add currentItem salary into the accumulator and finally return the sum of salary - Finally using javascript expressions to embed those values inside HTML table tags
import React, { Component } from "react";
import { render } from "react-dom";
export default class NumberSum extends Component {
constructor(props) {
super(props);
this.state = {
data: [
{
firstName: "John",
lastName: "Erwin",
email: "[email protected]",
salary: 5000,
},
{
firstName: "Ram",
lastName: "Kumar",
email: "[email protected]",
salary: 5000,
},
],
};
}
render() {
const tableBody = this.state.data.map((item) => (
<tr key={item.firstname}>
<td>{item.firstName}</td>
<td>{item.lastName}</td>
<td>{item.email}</td>
<td>{item.salary}</td>
</tr>
));
const total = this.state.data.reduce(
(total, currentItem) => (total = total + currentItem.salary),
0,
);
return (
<table>
<thead>
<tr>
<th>first</th>
<th>last</th>
<th>Email</th>
<th>Salary</th>
</tr>
</thead>
<tbody>{tableBody}</tbody>
<tfoot>
<tr>
Total:
{total}
</tr>
</tfoot>
</table>
);
}
}
Output
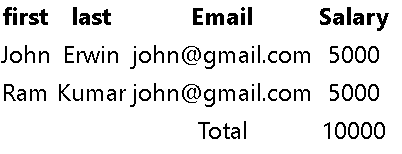
Conclusion
This is a short tutorial with examples of react
- How to display an array of objects into an HTML table
- Get the sum of an array of object number properties