Learn Primeng Angular ListBox |p-listbox examples
ListBox
is a graphical user component that displays a list of elements from which the user can select multiple elements at once.
The ListBox
can also show optional checkboxes
Create an Angular application from Scratch
First, create an angular application from scratch using the angular cli command.
And integrate primeng libraries into the Angular application
if you are not sure how to do it, You can check the below things.
Once primeng is installed into the application, the application is ready to run. You can import ListboxModule
into the angular application.
ListboxModule
is an angular module for list box components and directives from the primeng framework.
Add ListboxModule
in the application module
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppRoutingModule } from "./app-routing.module";
import { AppComponent } from "./app.component";
import { ButtonModule } from "primeng/button";
import { ListboxModule } from "primeng/listbox";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule, ButtonModule, ListboxModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
Angular ListBox example
First, Create a model object as defined below role.ts
interface Role {
name: string;
value: string;
}
export default Role;
Import model into an angular component
import Role from "./model/Role";
In the typescript component: Here are steps
- declare
roles
model object selectedRole
with the type ofany
type to hold the select values of the list box- Initialize the
roles
object with static data or pull from API
import { Component } from "@angular/core";
import Role from "./model/Role";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
title = "Primeng listbox example";
roles: Role[];
selectedRole: any;
constructor() {
this.roles = [
{ name: "Administrator", value: "ADMIN" },
{ name: "Sales", value: "SALE" },
{ name: "Marketing", value: "MKT" },
{ name: "HR", value: "HR" },
{ name: "Finance", value: "FIN" },
];
}
}
selectedRole
is declared using the two-way binding approach with the ngModel
attribute.
It holds a role object when the user selected an item in the Listbox.
Here is the template component.
<h1>{{title}}</h1>
<p-listbox
[options]="roles"
[(ngModel)]="selectedRole"
optionLabel="name"
></p-listbox>
<p>Selected Role: {{selectedRole ? selectedRole.name : 'none'}}</p>
Output:
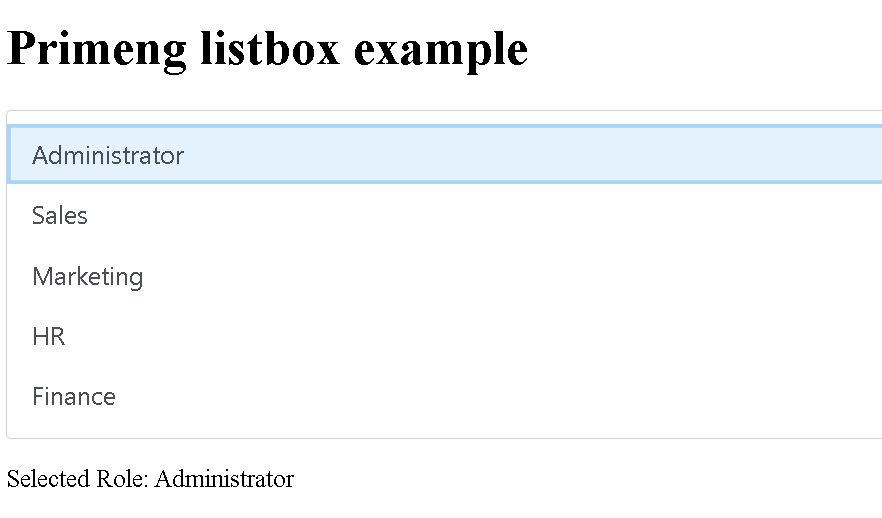
How to select the default list box item on page load?
In the above example, the selected Listbox element is populated selectedRole
object.
In the constructor, please assign the value
constructor() {
this.selectedRole = this.roles[0];
}
This will select the first element of the list box programmatically.
How to display list box with checkbox
This example adds the following features
- Select multiple elements
- Adding a checkbox to the list box
- Filter an items
typescript component:
import { Component, OnInit } from "@angular/core";
import Role from "../model/Role";
@Component({
selector: "app-listbox-checkbox",
templateUrl: "./listbox-checkbox.component.html",
styleUrls: ["./listbox-checkbox.component.scss"],
})
export class ListboxCheckboxComponent implements OnInit {
title = "Primeng listbox checkbox example";
roles: Role[];
selectedRoles: Role[];
constructor() {
this.roles = [
{ name: "Administrator", value: "ADMIN" },
{ name: "Sales", value: "SALE" },
{ name: "Marketing", value: "MKT" },
{ name: "HR", value: "HR" },
{ name: "Finance", value: "FIN" },
];
}
ngOnInit(): void {}
}
template component:
<h1>{{title}}</h1>
<p-listbox
[options]="roles"
[(ngModel)]="selectedRoles"
[metaKeySelection]="false"
[checkbox]="true"
[multiple]="true"
[filter]="true"
optionLabel="name"
[listStyle]="{'max-height':'250px'}"
[style]="{'width':'15rem'}"
>
<ng-template let-country pTemplate="item">
<div>{{country.name}}</div>
</ng-template>
</p-listbox>
{{selectedRoles|json}}
Output:
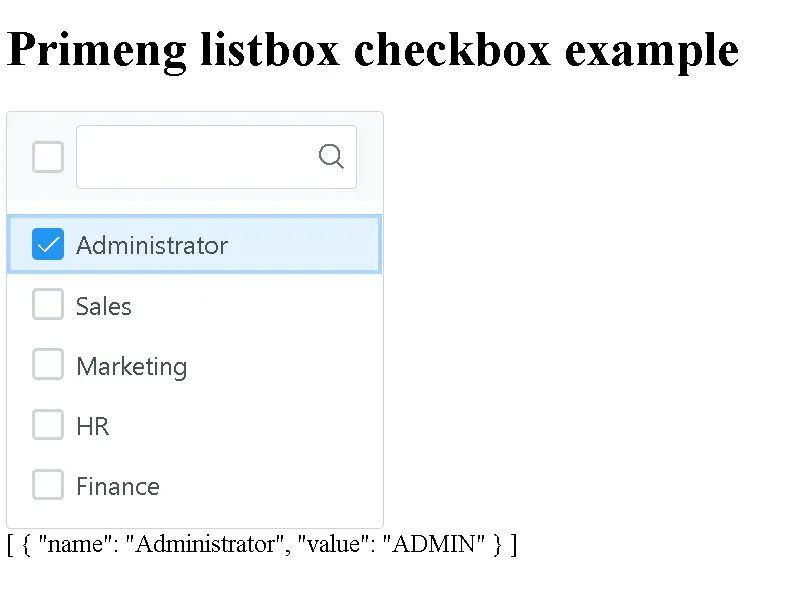
p-listbox is not working because of an error
The following are frequent errors when integrated into primeng listmodule
Can’t bind to ‘ngModel’ since it isn’t a known property of ‘p-listbox’
Error: src/app/app.component.html:3:30 - error NG8002: Can't bind to 'ngModel' since it isn't a known property of 'p-listbox'.
1. If 'p-listbox' is an Angular component and it has 'ngModel' input, then verify that it is part of this module.
2. If 'p-listbox' is a Web Component then add 'CUSTOM_ELEMENTS_SCHEMA' to the '@NgModule.schemas' of this component to suppress this message.
3. To allow any property add 'NO_ERRORS_SCHEMA' to the '@NgModule.schemas' of this component.
3 <p-listbox [options]="roles" [(ngModel)]="selectedRole" optionLabel="name"></p-listbox>
Solution is
add formsModule into app.module.ts
import { FormsModule } from "@angular/forms";
Here is a complete code:
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppRoutingModule } from "./app-routing.module";
import { AppComponent } from "./app.component";
import { ButtonModule } from "primeng/button";
import { ListboxModule } from "primeng/listbox";
import { FormsModule, ReactiveFormsModule } from "@angular/forms";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule, ListboxModule, FormsModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}