Primeng toast example | Angular Popup component
Toast
is a UI component interactive popup window for showing the progress of an application. Popup will be hidden automatically after a timeout.
Create Anguular Project
E:\work\angular\latest>ng new angular-primeng-toast-examples
? Which stylesheet format would you like to use? SCSS [ https://sass-lang.com/documentation/syntax#scss ]
? Do you want to enable Server-Side Rendering (SSR) and Static Site Generation (SSG/Prerendering)? No
CREATE angular-primeng-toast-examples/angular.json (2886 bytes)
CREATE angular-primeng-toast-examples/package.json (1061 bytes)
CREATE angular-primeng-toast-examples/README.md (1081 bytes)
CREATE angular-primeng-toast-examples/tsconfig.json (903 bytes)
CREATE angular-primeng-toast-examples/.editorconfig (274 bytes)
CREATE angular-primeng-toast-examples/.gitignore (548 bytes)
CREATE angular-primeng-toast-examples/tsconfig.app.json (263 bytes)
CREATE angular-primeng-toast-examples/tsconfig.spec.json (273 bytes)
CREATE angular-primeng-toast-examples/.vscode/extensions.json (130 bytes)
CREATE angular-primeng-toast-examples/.vscode/launch.json (470 bytes)
CREATE angular-primeng-toast-examples/.vscode/tasks.json (938 bytes)
CREATE angular-primeng-toast-examples/src/main.ts (250 bytes)
CREATE angular-primeng-toast-examples/src/favicon.ico (15086 bytes)
CREATE angular-primeng-toast-examples/src/index.html (313 bytes)
CREATE angular-primeng-toast-examples/src/styles.scss (80 bytes)
CREATE angular-primeng-toast-examples/src/app/app.component.html (20884 bytes)
CREATE angular-primeng-toast-examples/src/app/app.component.spec.ts (988 bytes)
CREATE angular-primeng-toast-examples/src/app/app.component.ts (389 bytes)
CREATE angular-primeng-toast-examples/src/app/app.component.scss (0 bytes)
CREATE angular-primeng-toast-examples/src/app/app.config.ts (227 bytes)
CREATE angular-primeng-toast-examples/src/app/app.routes.ts (77 bytes)
CREATE angular-primeng-toast-examples/src/assets/.gitkeep (0 bytes)
√ Packages installed successfully.
Successfully initialized git.
E:\work\angular\latest\angular-primeng-toast-examples>ng serve
? Would you like to share pseudonymous usage data about this project with the Angular Team
at Google under Google's Privacy Policy at https://policies.google.com/privacy. For more
details and how to change this setting, see https://angular.io/analytics. No
Global setting: enabled
Local setting: disabled
Effective status: disabled
Initial Chunk Files | Names | Raw Size
polyfills.js | polyfills | 82.71 kB |
main.js | main | 23.25 kB |
styles.css | styles | 96 bytes |
| Initial Total | 106.05 kB
Application bundle generation complete. [3.981 seconds]
Watch mode enabled. Watching for file changes...
➜ Local: http://localhost:4200/
Primeng Toast UI component
Toast
in an application is a popup window on the main DOM page.
In An application, Toast UI
is shown to the user for the following use case
- Authentication successful
- Give useful messages for Server validation errors - Duplicate records,
- Domain operation results message like the record is deleted from a database.- Confirm the popup window to delete the record
Primeng provides a lot of useful UI components in Angular. Toast
is one of the components that is used to display interactive messages in the overlay.
These tutorials cover Primeng Angular popup example but have not covered how to create an angular application.
install Primeng dependencies
Once your angular application is ready to work on, Please install npm dependencies
Primeng has the following dependencies to integrate into an angular application
Primeng npm module contains actual UI components, themes primeicons npm module provides all the icons for primeng UI library
Installation can be done in one of two always. One way is to install required dependencies npm install primeng command,
This will create required dependencies in the node_modules folder and also add entries in package.json
npm install --save primeng
npm install --save primeicons
And Another way is to provide dependencies in package.json
"primeicons": "^2.0.0",
"primeng": "9.0.6",
Please issue npm install
in the application root directory.
Once dependencies are installed, Next is to add Primeng CSS styles to your application
update styles to angular.json
There is an angular.json file located in the root directory of the angular application.
Angular.json has required configuration information related to styles, scripts, and required module configuration for lint, build and production builds.
Once you installed dependencies, primeng CSS files are located in “./node_modules/primeng/” for primeng and “./node_modules/primeicons” for primeicons.
styles in angular.json
provide global access to primeng styles to all your components in the angular application.
here configured primeicons.css
for primeng fonts, luna-blue is
the primeng theme and primeng.min.css
for required CSS styles for components.
"styles": [
"./node_modules/primeicons/primeicons.css",
"./node_modules/primeng/resources/themes/luna-blue/theme.css",
"./node_modules/primeng/resources/primeng.min.css"
]
import ToastModule into angular NgModule
If you want to access internal or external components, You need to import the module into your application module. Primeng provides toast components in ToastModule
.
And also includes MessageService
in the provider
section of the application module.
MessageService
is in the global scope and accessible across components of an application.
MessageService
is an API Service for displaying toast messages.
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { ToastModule, MessageService, ButtonModule } from "primeng";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppRoutingModule } from "./app.routing.module";
@NgModule({
imports: [
AppRoutingModule,
BrowserModule,
BrowserAnimationsModule,
ToastModule,
ButtonModule,
],
declarations: [AppComponent],
bootstrap: [AppComponent],
providers: [MessageService],
})
export class AppModule {}
p-toast button click examples
Create a basic component
In the template component, place the p-toast component inside it Html template component
<p>Basic toast example</p>
<p-toast></p-toast>
<button
type="button"
pButton
(click)="showMessage()"
label="Simple Toast Example"
class="ui-button-success"
></button>
Typescript code:
import { Component, OnInit } from '@angular/core';
import { MessageService } from 'primeng';
@Component({
selector: 'app-basic-toast',
templateUrl: './basic-toast.component.html',
styleUrls: ['./basic-toast.component.css']
})
export class BasicToastComponent implements OnInit {
constructor(private messageService: MessageService) {}
showMessage() {
this.messageService.add({severity:'info', summary: 'Record is added successully', detail:'record added'});
}
ngOnInit() {
}
}
Adding an object to messageService, Object content three fields
Severity
of toast message type, the Following severity types are supported.
success
: successful message type like record addedinfo
like loading a few more recordswarn
as duplicate records existerror
like a server error- `custom-like custom error
A summary
is the title of the toast message for Popup and the detail
is the subtitle of the toast popup
Output displayed in the browser
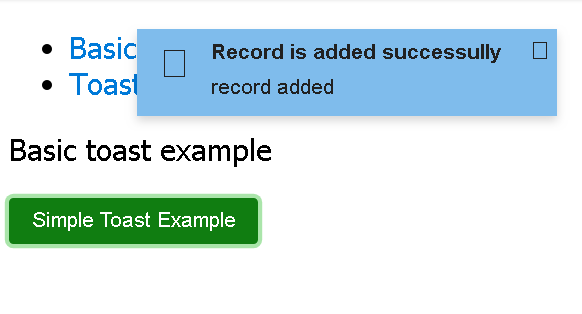
Change toast position in Angular position
By default, the toast popup is shown in the top-right position.
It can be controlled using the position attribute.
position possible values are
- top-left
- top-center
- center
- bottom-center
- bottom-left
- bottom-right
Created ToastPositionComponent
for showing an error popup in the top center ToastPositionComponent.ts:
import { Component, OnInit } from '@angular/core';
import { MessageService } from 'primeng';
@Component({
selector: 'app-toast-position',
templateUrl: './toast-position.component.html',
styleUrls: ['./toast-position.component.css']
})
export class ToastPositionComponent implements OnInit {
constructor(private messageService: MessageService) {}
showError() {
this.messageService.add({severity:'error', summary: 'Server error', detail:'500 error'});
}
ngOnInit() {
}
}
In the Html template, the position attribute is added
<p>toast position example</p>
<p-toast position="top-center"></p-toast>
<button
type="button"
pButton
(click)="showError()"
label=" Toast position Example"
class="ui-button-error"
></button>
manually remove toast clear method
By default popup shows for some time,
We can remove the toast using programmatically using the clear
method.
In HTML template components
<button type="button" pButton (click)="remove()" label="Remove"></button>
in typescript code, call the clean method of MessageService
this.messageService.clear();
customize styles
toast CSS can be customized by using the default CSS selector as well as a custom selector default, CSS selector,
ui-toast
: It is a popup window stylesui-toast-message
: This overrides the message content stylesui-toast-detail
: This overrides message detail styles
The below CSS style is to change the font family and color of the toast message content.
.newfont-styles .ui-toast-message {
font-family: "proxima nova";
color: "red";
}
To change the width of the toast popup
:host ::ng-deep .newfont-styles .ui-toast {
display: flex;
width: 70%;
}
Add CSS selector using class attribute to p-toast
component.
<p-toast class="newfont-styles"></p-toast>
HTML content
The below example illustrates about following things.
- Created ToastHtmlComponent
- In the component, toast content contains html content, not text htmlContent
- using ng-template inside p-toast component
- add innerHtml to div tag inside ng-templates
import { Component, OnInit } from "@angular/core";
import { MessageService } from "primeng";
@Component({
selector: "app-toast-html",
templateUrl: "./toast-html.component.html",
styleUrls: ["./toast-html.component.css"]
})
export class ToastHtmlComponent implements OnInit {
htmlContent: string = "";
showHtmlContent(description) {
this.htmlContent = "<h1>Heading 1<h1>\n<h2>Heading 2<h2>";
this.messageService.add({
severity: "info",
summary: "Summary content",
detail: ""
});
}
constructor(private messageService: MessageService) {}
ngOnInit() {}
}
And html component:
<p>HTML content toast example</p>
<p-toast position="top-center">
<ng-template let-message pTemplate="message">
<div innerHtml="{{htmlContent}}"></div>
</ng-template>
</p-toast>
<button
type="button"
pButton
(click)="showHtmlContent()"
label="Toast html content Example"
class="ui-button-success"
></button>
And the output is
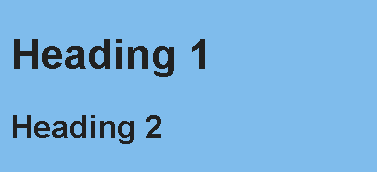
delete confirm toast popup window in Angular
Sometimes, we have a data table in an application, the user needs to notify the message during successful CRUD operations,
The below example talks about how to show confirm windows to ask for the user yes or no based on user input.
For example, In the table of records, you will ask to confirm a window to delete a record.
Confirm window will be shown to the user, if the user click yes, the popup disappears and calls API for deleting records from a Database, if the user clicks no, the popup closes do nothing.
onYes() {
this.messageService.clear('r');
// call delete Service API to record from the database
}
onNo() {
this.messageService.clear('r');
}
<p-toast
position="center"
key="r"
(onClose)="onNo()"
[modal]="true"
[baseZIndex]="1000"
>
<ng-template let-content pTemplate="content">
<div style="text-align: center">
<h3>{{content.summary}}</h3>
<p>{{content.detail}}</p>
</div>
<div class="ui-g ui-fluid">
<div class="ui-g-6">
<button
type="button"
pButton
(click)="onYes()"
label="Yes"
class="ui-button"
></button>
</div>
<div class="ui-g-6">
<button
type="button"
pButton
(click)="onNo()"
label="No"
class="ui-button"
></button>
</div>
</div>
</ng-template>
</p-toast>
Angular primeng toast errors
The below section talks about various errors that used to occur during primeng toast usage
p-toast is not a known element
It is a common error that happens for every developer during angular coding.
The p-toast
component element is used in the HTML template, but the element is not able to recognize.
The fix for the issue is
Please make sure that primeng is installed correctly, Check package.json has required primeng dependencies and the node_modules folder contains primeng dependencies
import ToastModule into your angular module so that all components in the modules are available in your component
s
import { ToastModule, MessageService, ButtonModule } from "primeng";
@NgModule({
imports: [ToastModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
providers: [MessageService],
})
export class AppModule {}
toast stackblitz example
Complete working examples for this article found in angular toast primeng example stacblitz🔗
Conclusion
In these tutorials, You learned how to integrate toast into angular applications. And also examples for deleting confirm toast popup window, customizing styles, and deleting the toast message using clear. It also includes errors and fixes.