How to change navbar color in Bootstrap with css
This post talks about multiple ways to solve the below items in Bootstrap 4.
- How to change the color of text color in the navbar bootstrap
- Change the background color navigation bar
navbar in bootstrap can be created as follows
<nav class="navbar navbar-default" role="navigation"></nav>
The default background color is white and the color is blue. In bootstrap, Changing the color of navigation is not that easy, as it contains brand and anchor links inside it.
Bootstrap classes are LESS-based styles, so you need to know the parent and child classes used inside the navigation bar.
Navigation bar text color change
By default navigation bar is transparent, which means the text color is synced with background-color
Bootstrap provides two inbuilt selectors for text colors.
- navbar-light This is used to change the text color to dark from light and background colors are light only.
- navbar-dark It changes text color from light to dark and the background color is dark.
Background color change
Bootstrap 4.5 provides the following inbuilt classnames we can use in the navigation bar
- bg-primary - primary color
- bg-secondary - change background color to secondary
- bg-success - change background to success color
- bg-danger - updates background to danger color
- bg-warning - warning color is updated in the background
- bg-info - information color is updated in the background
- bg-light- light color
- bg-dark - dark color
- bg-white - background color is white
So we can use the following approaches to change colors for navigation
- custom css class
- style attribute
- Inbuilt selectors
Here is an example using navbar-light/dark and bg-light/dark
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<div class="collapse navbar-collapse" id="navbarTogglerDemo01">
<a class="navbar-brand" href="#">Website</a>
<ul class="navbar-nav mr-auto mt-2 mt-lg-0">
<li class="nav-item active">
<a class="nav-link" href="#">Home </a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
</ul>
</div>
</nav>
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<div class="collapse navbar-collapse" id="navbarTogglerDemo01">
<a class="navbar-brand" href="#">Website</a>
<ul class="navbar-nav mr-auto mt-2 mt-lg-0">
<li class="nav-item active">
<a class="nav-link" href="#">Home </a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
</ul>
</div>
</nav>
<nav class="navbar navbar-expand-lg navbar-dark bg-success">
<div class="collapse navbar-collapse" id="navbarTogglerDemo01">
<a class="navbar-brand" href="#">Website</a>
<ul class="navbar-nav mr-auto mt-2 mt-lg-0">
<li class="nav-item active">
<a class="nav-link" href="#">Home </a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
</ul>
</div>
</nav>
<nav class="navbar navbar-expand-lg navbar-dark bg-danger">
<div class="collapse navbar-collapse" id="navbarTogglerDemo01">
<a class="navbar-brand" href="#">Website</a>
<ul class="navbar-nav mr-auto mt-2 mt-lg-0">
<li class="nav-item active">
<a class="nav-link" href="#">Home </a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Link</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
</ul>
</div>
</nav>
Output:
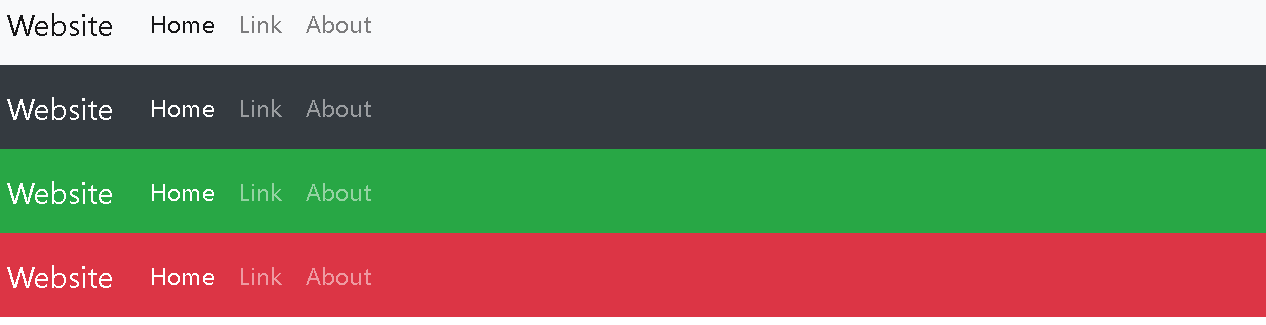
Inbuilt selectors
We can override the inbuilt selectors in CSS for color, However, this will affect other places where these classnames are being used. It is not recommended to use the override classnames
custom css class
This is another way existing CSS selectors are not touched, It is a clean way of changing color. Create a CSS class navbar-customclass or your name
// custom class for background
.navbar-customclass {
background-color: blue;
}
/* changing brand text color*/
.navbar-customclass .navbar-brand,
.navbar-customclass .navbar-text {
color: #ffffff;
}
/* navigation link text color */
.navbar-customclass .navbar-nav .nav-link {
color: rgba(255, 255, 255, 0.6);
}
/* navigation links anchor hover and active styles */
.navbar-customclass .nav-item.active .nav-link,
.navbar-customclass .nav-item:hover .nav-link {
color: #ffffff;
}
Codepen example
This example is the code for creating a custom class for the navigation bar.
style attribute It is not a recommended approach as it only applies to inline elements of the navigation bar, You have applied them in each child element of a navbar.
So we have to change the entire brand, and nav links colors, It is not recommended approach.