Async await basic tutorials and examples
- Admin
- Mar 6, 2024
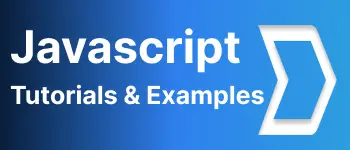
This tutorial explains async
and await
with examples.
Async functions are used for asynchronous programming. They always return a promise. If the function doesn’t return a promise explicitly, it wraps the result in one.
How to Declare Async Functions in Multiple Ways
Async functions are used to perform asynchronous tasks. They pause the current execution and place it in a separate queue called the event queue. In real-time applications, async functions are commonly used for API processing.
async normal functions Async function are declared with the keyword
async
. It is a normal functionasync function getMessage() { return "Welcome"; }
which is equal to
async function getMessage() { return Promise.resolve("Welcome"); }
async function anonymous expressions Async functions can also be declared anonymously without a name. These can be assigned to a variable.
const variable = async function () { // do something };
Calling an async function always returns a Promise. You can explicitly return either a
promise
or avalue
wrapped within a promise.console.log(getMessage())
Async Arrow functions examples
Arrow functions can be declared and assigned to JavaScript variables. They can be declared with or without arguments.
Async Arrow function without arguments
const getMessage = async () => {
console.log("Hello ")
}
Async with single arguments. Parentheses for a single argument are optional.
const getMessage = async (msg) => {
console.log("Hello ",msg)
}
or
const getMessage = async msg => {
console.log("Hello ",msg)
}
Multiple arguments to arrow async functions
const getMessage = async (msg1,msg2) => {
console.log("Hello ",msg)
}
- Async function also declared with static
static myfunc = async () => {
console.log("static function ")
}
How to Call Async Functions
Async functions are asynchronous and always return a Promise.
Calling an async function always returns a Promise.
- Using the Await Keyword
Async
functions are called using the await
keyword.
await
waits for a function to finish its execution. It can only be called within an async
function.
async function getMessage() {
return "Welcome";
}
console.log(getMessage())
async function callMessage() {
const result = await getMessage();
console.log(result); // will print "welcome"
}
callMessage()
If await is called without an async function, it throws an error:
SyntaxError: await is only valid in async functions and the top-level bodies of modules
async function getMessage() {
return "Welcome";
}
console.log(getMessage())
function callMessage() {
const result = await getMessage();
console.log(result); // will print "Hello world!"
}
callMessage()
- How to Call Async Functions in Synchronized Functions
You have to use the then
and catch
blocks in synchronized functions.
then()
is used for successfully resolved promises.
async function getMessage() {
return "Welcome";
}
async function callMessage() {
getMessage()
.then(data=>console.log("success",data))
.catch(error=>console.log("error: ",error))
}
callMessage()// Prints success Welcome
- async functions throws an error
If an async function throws an error, the catch
block is called to handle the error.
async function getMessage() {
throw "failed";
}
async function callMessage() {
getMessage()
.then(data=>console.log(data))
.catch(error=>console.log("error",error))
}
callMessage() // error failed
- Async Functions Rejecting Promises
If an async function rejects a promise, the catch function is called with an error.
async function getMessage() {
Promise.reject("fail");
}
async function callMessage() {
getMessage()
.then((data) => console.log("success", data))
.catch((error) => console.log("error", error));
}
callMessage();
Above code throws
[UnhandledPromiseRejection: This error originated either by throwing inside of an async function without a catch block or by rejecting a promise which was not handled with .catch(). The promise was rejected with the reason "fail".] {
code: 'ERR_UNHANDLED_REJECTION'
success undefined
node:internal/process/promises:279
triggerUncaughtException(err, true /_ fromPromise _);
^
[UnhandledPromiseRejection: This error originated either by throwing inside of an async function without a catch block or by rejecting a promise which was not handled with .catch(). The promise was rejected with the reason "fail".] {
code: 'ERR_UNHANDLED_REJECTION'
}
It calls a catch block with an undefined result.
- async functions returns rejected promise
catch function call back is called with an error
async function getMessage() {
return Promise.reject("fail")
}
async function callMessage() {
getMessage()
.then(data=>console.log("success",data))
.catch(error=>console.log("error",error))
}
callMessage();
Output:
error fail