Angular Input numeric validation | Typescript
numeric in the form is the basic level of validation in the Angular application. Input form type=text/number
only allows numeric fields and other alphabets and special characters are not allowed. In Html, numbers are entered via input form with input type=text in HTML4 or less, type=number in HTML5 language
In this Angular tutorial, I am going to explain input-type numeric validation in different ways.
Angular offers multiple ways to handle numeric form validation.
- Accept numbers only with Angular forms.
- HTML5 forms
Other versions available:
Url pattern validation can be implemented with multiple approaches.
This tutorial works for all angular versions 2,5,6 8,9,10,11
Let’s get started.
Angular input number validation
Angular offers form handling in two ways. Reactive Forms are used to handle form input controls with a model drive approach to maintain the state between form handling. Template forms work with two-way binding between the model and control to maintain the form state.
Let’s see examples in both ways to handle numeric validations while the focus is lost (onBlur) on the input form.
Reactive form with onBlur event
First, Import ReactiveFormsModule
in the app.module.ts file to enable Reactive form handling. app.module.ts:
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule, ReactiveFormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule, FormsModule, ReactiveFormsModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Next, create an Angular component, Let’s name it an Input-number-reactive component.
It creates three files in an input-number-reactive folder of the application/src folder.
input - number - reactive.component.html;
input - number - reactive.component.ts;
input - number - reactive.component.css;
In Html template component: input-number-reactive.component.html
- Add HTML form with formGroup directive, named this as yearForm
- In the form, Declare the input form by adding formControlName with its value as the year
- validation errors state is maintained in yearForm, so display the validation errors as seen below
- yearForm.controls[‘year’].valid returns true if no validation errors, else false
- yearForm.controls[‘year’].touched returns true if the already user entered the values
- validation logic is added to display or hide the errors
Html Template component code:
<div>
<h2>{{ heading | titlecase }}</h2>
<form [formGroup]="yearForm">
<input type="text" formControlName="year" />
<div
*ngIf="!yearForm.controls['year'].valid && yearForm.controls['year'].touched"
>
<div
*ngIf="yearForm.controls['year'].errors.required"
style="background-color: red"
>
Year is required
</div>
<div
*ngIf="yearForm.controls['year'].errors.pattern"
style="background-color: red"
>
Only Numbers are allowed
</div>
</div>
</form>
<button type="submit ">Submit</button>
</div>
In component typescript code,
- declared variable yearForm of type
FormGroup
, which should have binding to form element in HTML template - Defined the variable for regular expression for numeric only.
- In the constructor, Initialize formGroup with FormControl object
- FormControl object accepts the first parameter as the initial value empty
- Second parameters object with validators - Required and Regular expression pattern
- Validators.required returns true if form control is empty -Regular expression is passed to Validators.pattern method to enable numeric validation
- updateOn tells how the model changes its behavior, in this case, the onBlur event -Validators are executed on input focus lost
Typescript component code:
import { Component, OnInit } from "@angular/core";
import { FormControl, FormGroup, Validators } from "@angular/forms";
@Component({
selector: "app-input-number-reactive",
templateUrl: "./input-number-reactive.component.html",
styleUrls: ["./input-number-reactive.component.css"],
})
export class InputNumberReactiveComponent {
heading = "angular Input Numeric examples";
yearForm: FormGroup;
myusername: string = "";
numberRegEx = /\-?\d*\.?\d{1,2}/;
constructor() {
this.yearForm = new FormGroup({
year: new FormControl("", {
validators: [Validators.required, Validators.pattern(this.numberRegEx)],
updateOn: "blur",
}),
});
}
submit() {
console.log(this.yearForm.value);
}
}
Output:
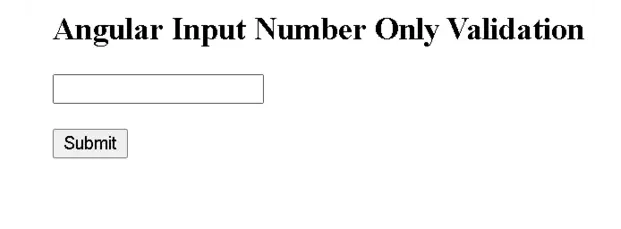
Template-driven forms
It has support for input type=number in the latest modern browsers. HTML5 has support for input type=number combined with regular expression patterns.
In the Component html template code,
- Input is defined with type=number for the text box. Even though it specified the number, You have to add validation to make it work
- For validation to work, the
pattern
attribute is added for numbers only NgModel
directive was added to have binding from the controller to html and vice versa, that tells any value change UI propagates to Model object and also applies to changes in the model to UI- And also local reference is declared #yearerror with value ngModel which holds the state of input controls
ngModelOptions
directive tells to model to change its value on updateOn event configured. which means, validation executed on blur event
<div>
<input
type="text"
name="year"
id="year"
placeholder="enter year"
[(ngModel)]="year"
[ngModelOptions]="{ updateOn: 'blur' }"
#yearerror="ngModel"
pattern="/\-?\d*\.?\d{1,2}/"
required
/>
<div *ngIf="yearerror.invalid && (yearerror.dirty || yearerror.touched)">
<div *ngIf="yearerror.errors.required">
<span style="background-color: red">Year is required </span>
</div>
<div *ngIf="yearerror.errors.pattern">
<span style="background-color: red">Only Numbers allowed </span>
</div>
</div>
</div>
Typescript component code:
In the component, if the two-way binding is defined in HTML code, then the variable is declared with a default value.
import { Component, OnInit } from "@angular/core";
@Component({
selector: "app-input-number-template",
templateUrl: "./input-number-template.component.html",
styleUrls: ["./input-number-template.component.css"],
})
export class InputNumberTemplateComponent {
constructor() {}
year: number;
}
Typescript url regexp validation
Sometimes, We want to do a validation typescript file.
- It uses the same above regular expression pattern for url validation
- RegExp object is created with regex
pattern.test
checked against supplied url and return true-valid
public isValidUrl(urlString: string): boolean {
try {
let pattern = new RegExp(this.urlRegEx);
let valid = pattern.test(urlString);
return valid;
} catch (TypeError) {
return false;
}
}
}
}
console.log(this.isValidUrl('https://www.google.com')); // true
console.log(this.isValidUrl('google')); // false
Angular input type numbers allow numbers only
HTML5 provides input type=number in web pages. This example tells us to accept numbers only by allowing alphabets.
This will be achieved with the keypress event which is called whenever a key is pressed on the keyboard
The call-back handler gets the char code and checks this code against regular numeric expressions. if an entered key is a number, allowed, else not allowed.
In html template,
<input type="number" (keypress)="onKeyPress($event)" />
Typescript code:
onKeyPress(event: any) {
const regexpNumber = /[0-9\+\-\ ]/;
let inputCharacter = String.fromCharCode(event.charCode);
if (event.keyCode != 8 && !regexpNumber.test(inputCharacter)) {
event.preventDefault();
}
}
Conclusion
In this post, You learned
- Angular number validation using regular expression examples with reactive forms
- HTML 5 input type number template form validation
- only numbers are allowed in the input number