Angular ngFor index - How to get current last even and odd index?
- Admin
- Dec 31, 2023
- Typescript Angular Angular12
This tutorial explains how to get the current last even and odd index with ngfor in angular.
Let’s create an employee model.
export class Employee {
id: Number;
name: string;
salary: Number;
}
Angular ngFor Index syntax and example
ngFor
is a shorthand form for ngForOf
directive This can be used to iterate an array of objects or objects.
Here is a ngFor
syntax
<div *ngFor="let object of objectarray; let i=index;">
{{i}}/{{users.length}}. {{user}} <span*ngIf="isFirst">default</span>
</div>
This can be applied to any HTML element objectarray - an array of object object - typescript object or model class there are different local variables we can use
index
: get the index of a current item in an arraycount
- length of an array that is equal to the array.lengthfirst
: returns true if the current object is the first object, else false.last
: returns true if the current object is the last, else false.even
: returns true if a current object index is an even numberodd
: returns true if a current object index is an odd number
How to get the first last event odd index of an Object Array
Let’s create an Angular component
- Initialize an object array with employee data
import { Component } from "@angular/core";
import { Employee } from "./employee";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
employees: Employee[] = [
{ id: 1, name: "Ram", salary: 5000 },
{ id: 2, name: "John", salary: 1000 },
{ id: 3, name: "Franc", salary: 3000 },
{ id: 4, name: "Andrew ", salary: 8000 },
];
constructor() {}
ngOnInit() {}
}
ngFor
is used to iterate an enumerated object-like array of objects.
<table style="width:100%">
<tr>
<th>name</th>
<th>index</th>
<th>Count</th>
<th>First</th>
<th>Last</th>
<th>Odd</th>
<th>Even</th>
</tr>
<tr
*ngFor="let employee of employees; let i=index;let count=count;let first=first;let last=last;let even=even;let odd=odd;"
>
<td>{{employee.name}}</td>
<td>{{i}}</td>
<td>{{count}}</td>
<td>{{first}}</td>
<td>{{last}}</td>
<td>{{even}}</td>
<td>{{odd}}</td>
</tr>
</table>
outputs:
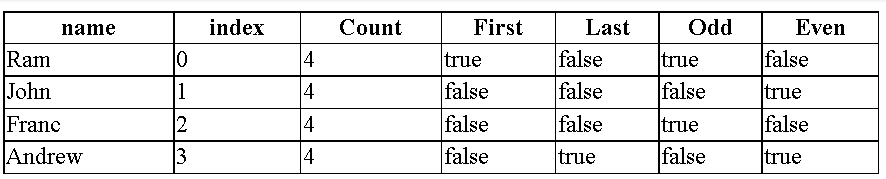
Conclusion
ngFor directive used to iterate an object array, can get current index as well check for a current index is first
, last
, odd
, and event
.