Angular 15 Setup Mock API and consume and return data
In this post, You will learn how to create a Simple mock API to return json data in an Angular application without creating a backend API
Sometimes, We want to test data components using API code, if there is no backend code,
This tutorial explains How to create a Backend REST API for mock data which returns json objects in the Angular application.
First Create an angular application
First, check angular CLI or not using ng -version
C:\Users\Kiran>ng --version
_ _ ____ _ ___
/ \ _ __ __ _ _ _| | __ _ _ __ / ___| | |_ _|
/ △ \ | '_ \ / _` | | | | |/ _` | '__| | | | | | |
/ ___ \| | | | (_| | |_| | | (_| | | | |___| |___ | |
/_/ \_\_| |_|\__, |\__,_|_|\__,_|_| \____|_____|___|
|___/
Angular CLI: 12.0.1
Node: 14.17.0
Package Manager: npm 7.11.1
OS: win32 x64
Angular: undefined
...
Package Version
------------------------------------------------------
@angular-devkit/architect 0.1200.1 (cli-only)
@angular-devkit/core 12.0.1 (cli-only)
@angular-devkit/schematics 12.0.1 (cli-only)
@schematics/angular 12.0.1 (cli-only)
typescript 3.6.3
if Angular CLI is installed, use the npm install @angular/cli
command to install it.
Next, Create an angular application using ng new command
B:\githubwork\angular-mock-api-json>ng new
? What name would you like to use for the new workspace and initial project? no
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
CREATE no/angular.json (3015 bytes)
CREATE no/package.json (1064 bytes)
CREATE no/README.md (1048 bytes)
CREATE no/tsconfig.json (783 bytes)
CREATE no/.editorconfig (274 bytes)
CREATE no/.gitignore (604 bytes)
CREATE no/.browserslistrc (703 bytes)
CREATE no/karma.conf.js (1419 bytes)
CREATE no/tsconfig.app.json (287 bytes)
CREATE no/tsconfig.spec.json (333 bytes)
CREATE no/src/favicon.ico (948 bytes)
CREATE no/src/index.html (288 bytes)
CREATE no/src/main.ts (372 bytes)
CREATE no/src/polyfills.ts (2820 bytes)
CREATE no/src/styles.css (80 bytes)
CREATE no/src/test.ts (743 bytes)
CREATE no/src/assets/.gitkeep (0 bytes)
CREATE no/src/environments/environment.prod.ts (51 bytes)
CREATE no/src/environments/environment.ts (658 bytes)
CREATE no/src/app/app-routing.module.ts (245 bytes)
CREATE no/src/app/app.module.ts (393 bytes)
CREATE no/src/app/app.component.html (23809 bytes)
CREATE no/src/app/app.component.spec.ts (1045 bytes)
CREATE no/src/app/app.component.ts (206 bytes)
CREATE no/src/app/app.component.css (0 bytes)
√ Packages were installed successfully.
The directory is already under version control. Skipping initialization of git.
json-server for Backendless REST API using JSON
json-server
is an expression-based web server that has all REST API operations using JSON as a database.
It helps UI developers prototype or mock REST APIs using JSON files as a backend database.
It allows you to set up and run a REST API server in 5 minutes without coding and helps developers to test UI components.
JSON-server features
- Any changes to the object will update the database json file
- It also provides pagination data during the list of items
- Provides authentication to add security
- Customize Routes using the routes option
--routes
- Add middleware code to apply business logic using
--middlewares
- It is easier and integrates in 5 minutes
- Validations like 404 and duplicate are supported
npm install --save json-server
Next, create a folder API
under the project directory.
Create a database.json in API
{
"users": []
}
Either we can insert dummy data or use the faker
npm library
faker npm library generates dummy data
faker.js is an npm library for generating fake real-time data First, install faker to the angular app
npm install faker --save
Next, Insert dummy data using javascript code.
- Create a users database that holds a list of user information
- using for loop insert 100 users with fake data and push to an array of users
- We have users array objects in database string.
- Convert this object to json string using the JSON.stringfy function
- We have to create a database.json using the
writeFile
method of the fs module writeFile
accepts file path, json object, encoding, and callback- callback throws an error if json file is not created.
Here is InsertIntoDB.js which creates a database.json file
var faker = require("faker");
var fs = require("fs");
var database = { users: [] };
for (var i = 1; i <= 100; i++) {
database.users.push({
id: i,
name: faker.name.findName(),
email: faker.internet.email(),
username: faker.commerce.userName,
password: faker.commerce.password,
});
}
var json = JSON.stringify(database);
fs.writeFile("api/database.json", json, "utf8", (err) => {
if (err) {
console.error(err);
return;
}
console.log("database.json created");
});
let’s configure npm scripts for the below things in package.json
- insert: this generates faker data in json string and creates database.json
"insert": "node ./api/InsertIntoDB.js",
- server: let’s configure the json-server to start the server and the input database file is included
"server": "json-server --watch ./api/database.json"
Here is the package.json file
{
"name": "angular-mock-api-json",
"version": "0.0.0",
"scripts": {
"ng": "ng",
"start": "ng serve",
"build": "ng build",
"watch": "ng build --watch --configuration development",
"test": "ng test",
"insert": "node ./api/InsertIntoDB.js",
"server": "json-server --watch ./api/database.json"
},
"private": true,
"dependencies": {
"@angular/animations": "~12.0.1",
"@angular/common": "~12.0.1",
"@angular/compiler": "~12.0.1",
"@angular/core": "~12.0.1",
"@angular/forms": "~12.0.1",
"@angular/platform-browser": "~12.0.1",
"@angular/platform-browser-dynamic": "~12.0.1",
"@angular/router": "~12.0.1",
"faker": "^5.5.3",
"json-server": "^0.16.3",
"rxjs": "~6.6.0",
"tslib": "^2.1.0",
"zone.js": "~0.11.4"
},
"devDependencies": {
"@angular-devkit/build-angular": "~12.0.1",
"@angular/cli": "~12.0.1",
"@angular/compiler-cli": "~12.0.1",
"@types/jasmine": "~3.6.0",
"@types/node": "^12.11.1",
"jasmine-core": "~3.7.0",
"karma": "~6.3.0",
"karma-chrome-launcher": "~3.1.0",
"karma-coverage": "~2.0.3",
"karma-jasmine": "~4.0.0",
"karma-jasmine-html-reporter": "^1.5.0",
"typescript": "~4.2.3"
},
"_id": "[email protected]"
}
Next, run the above code using the npm run command to create a database
npm run insert
This creates a file in database.json in the API folder.
Start json server using npm run command
npm run server
B:\githubwork\angular-mock-api-json>npm run server
> no@0.0.0 server
> json-server --watch ./api/database.json
\{^_^}/ hi!
Loading ./api/database.json
Done
Resources
http://localhost:3000/users
Home
http://localhost:3000
Type s + enter at any time to create a snapshot of the database
Watching...
This starts the server and is accessible at http://localhost:3000/users
By Default, This provides different APIs available
URL | HTTP Method Type | Description |
---|---|---|
/users | GET | List of Users |
/users/{id} | GET | Retrieve User by Id |
/users | POST | Create an user object |
/users/{id} | PUT | Update an user object by Id |
/users/{id} | PATCH | Update an user object with partial properties by Id |
/users{id} | DELETE | Delete an user object by ID |
if any changes are made to the user object using POST, PUT, PATCH, DELETE request, JSOn-server updates database.json instantly.
Angular Example to consume Mock Data
There are a couple of steps to Consume REST API in angular application. Frontend apps communicate with Backend apps or REST API using HTTP Connection `@angular/common/HTTP provides HttpClient class
HttpClient is a component used to connect and issue HTTP requests to REST API.
Before using HttpClient, You have to import HttpClientModule
Setup HttpClientModule into the module
Import HttpClientModule into angular app.module.ts
.
All the classes and services available in this module are accessed across all components of app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppRoutingModule } from "./app-routing.module";
import { AppComponent } from "./app.component";
import { HttpClientModule } from "@angular/common/http";
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, AppRoutingModule, HttpClientModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
First Create an Interface model for user data. You can choose interface or class for model
export interface User {
id: number;
name: string;
email: string;
username: string;
}
Let’s first create a component app.component.ts In Angular Component:
Created a User Array object
Initialize the User array with an empty array in the constructor if you skipped this step, you will get an error
Property 'users' has no initializer and is not assigned in the constructor.ts(2564)
You can check more information on how to fix this error
Inject
HttpClient
in the constructor to use HttpClient class methodsMake an HTTP
get
request usingHttpClient.get
get
API returns a response in a JSON object and converts it to a Typescript User object.In the HTML template file, using ngFor loop iterate the
users
object and display it to UI.In the scss file, add CSS styles for alternative table row colors. app.component.ts:
import { HttpClient } from '@angular/common/http';
import { Component } from '@angular/core';
import { Observable } from 'rxjs';
import { User } from './model/User';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss']
})
export class AppComponent {
users: User[];
title = 'angular-crud-mock-api';
constructor(private http: HttpClient) {
this.users = [];
this.getUsers();
}
getUsers() {
console.log('calling')
this.http.get('http://localhost:3000/users')
.subscribe(res => this.users =
res as User[]);
}
}
app.component.html:
<h1>Users List</h1>
<table style="width:100%">
<tr>
<th>id</th>
<th>username</th>
<th>email</th>
</tr>
<tr *ngFor="let user of users!">
<td>{{user.id}}</td>
<td>{{user.name}}</td>
<td>{{user.email}}</td>
</tr>
</table>
app.component.scss:
table {
border-collapse: collapse;
width: 100%;
th,
tr {
border: 1px solid #ccc;
}
th,
td {
text-align: left;
}
tr:nth-child(even) {
background-color: #f0f0f0;
}
}
These examples call mock REST APIs and display them in the table
Output
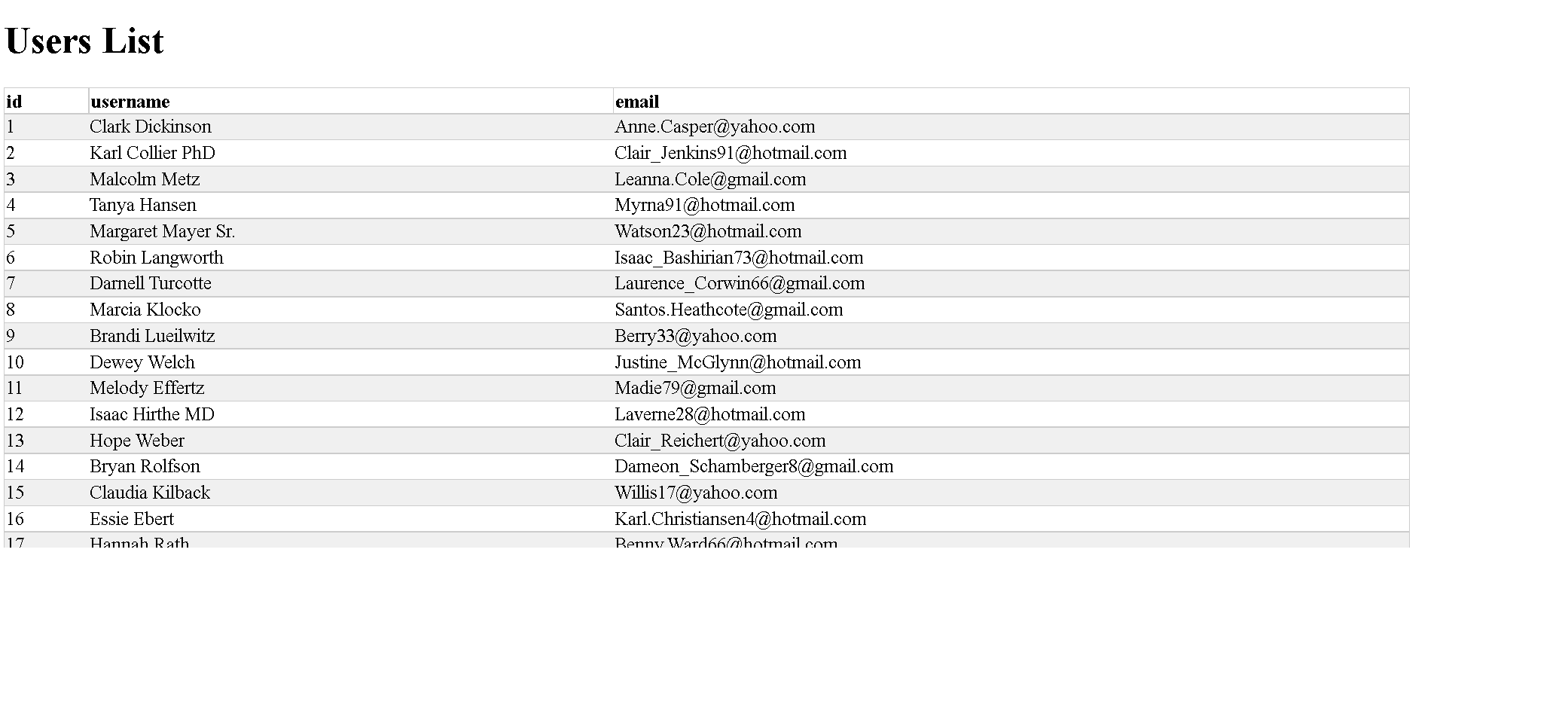
Conclusion
To Summarize, We learned A Step By Step Tutorial for Creating an angular application with CLI Add json-server for a mock API server add a faker for dummy data for CRUD API write a package.json script for inserting data into the database json and start json server add HTTP client module, and consume Mock API in angular app component.