Angular material Snackbar tutorials | mat-snackbar examples
You can see my previous about Angular button click event example
What is snackbar UI component?
Snackbar
is an important UI element in designing front-end applications.
It is a small popup window, that displays reactive information messages to accept user input from a user.
It does dismiss with user action either swapping or a user click.
Important points about Material Snackbar Design component
- It shows a notification message popup at bottom of the screen by default
- It does not interrupt the user experience on the current page
- The recommendation is only one snack bar shown at a time on a page
- Not required to have user action
- Automatically disappear after some time
- Has support for Android, IOS, and Web applications
In Android, the user notified a snack bar message for the below use cases
- the internet is offline or line
- some plugins are not installed
- Any important message that the user notified- Record delete, Revert button shown In this tutorial, How do we implement a snack bar in angular applications using the material library?
Angular Snackbar Configuration
The following are configuration parameters that are being supplied to the snack bar component.
Parameter | Description |
---|---|
data | Data passed to component |
direction | Data passed to component |
duration | time in milliseconds shown before disappearing from the page |
panelClass | used to Customize the snackbar CSS styles |
horizontalPosition | Horizontal position - ‘start’, ‘center’,‘end’,‘left’, ‘right’ |
verticalPosition | Vertical position - ‘top’,‘bottom’ |
Import MatSnackBarModule into the angular application
The MatSnackBarModule
module provides snack bar-related functional components.
To use these components, Import them into your application module as follows.
Now imported in app.module.ts, all components of the module are available across all your components.
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { AppRoutingModule } from "./app.routing.module";
import { FormsModule, ReactiveFormsModule } from "@angular/forms";
import { BasicSnackbarComponent } from "./basic-snackbar/basic-snackbar.component";
import { MatButton, MatButtonModule } from "@angular/material/button";
import { MatSnackBarModule } from "@angular/material/snack-bar";
@NgModule({
imports: [
AppRoutingModule,
BrowserModule,
BrowserAnimationsModule,
FormsModule,
ReactiveFormsModule,
MatButtonModule,
MatSnackBarModule,
],
declarations: [AppComponent, BasicSnackbarComponent],
bootstrap: [AppComponent],
providers: [],
exports: [BasicSnackbarComponent],
})
export class AppModule {}
The angular module for this component is as above.
Simple Basic component
This section covers basic components with message, action, and duration
Notification message
This is an example of showing a simple notification offline message on clicking the button
In the Html template component,
- Added material Button in HTML component
- provided click event for showing a simple snack bar notification popup
- passing a content message to the click event method which displays in a popup
<button
mat-raised-button
color="primary"
(click)="showSnackbar('Net is offline, Trying to reconnect ....')"
>
Simple Snackbar
</button>
In the Typescript component,
- Injected
MatSnackBar
in the constructor and the object of this is available during the component scope - Created method showSnackbar with a content parameter, which does opening snackbar logical
- called
Open()
method of snackbar object
import { Component } from "@angular/core";
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
constructor(private snackBar: MatSnackBar) {}
showSnackbar(content) {
this.snackBar.open(content);
}
}
On the clicking button, the following is an output seen in the browser.
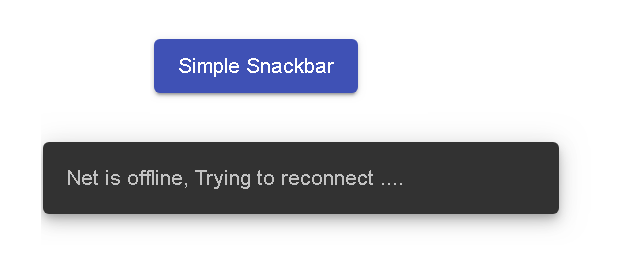
Action button with a message
This is an example of showing a button in the snack bar including a message.
On the clicking button in the snackbar, it disappears from the page.
In the HTML template component,
- Created button added click handler method two parameters
- the first parameter is the message, Second parameter action button label
<button
mat-raised-button
color="primary"
(click)="showSnackbarAction('Net is offline, Trying to reconnect ....','Done')"
>
Snackbar action
</button>
In the component ts file, open() method the second parameter is a button displayed in the snack bar.
showSnackbarAction(content,action) {
this.snackBar.open(content,action);
}
By default, the button disappears from the page, Snackbar has inbuilt events on the displayed object to have full control programmatically
afterDismissed
- This returns an observable when the snackbar disappears from its page afterOpened
- Returns an observable after being displayed on the page dismiss
- Closing it programmatically onAction
- This is an observable handling button that clicks events
An example of snackbar subscribing events.
let snack = this.snackBar.open(content, action);
snack.afterDismissed().subscribe(() => {
console.log("This will be shown after snackbar disappeared");
});
snack.onAction().subscribe(() => {
console.log("This will be called when snackbar button clicked");
});
And the output is shown in the browser
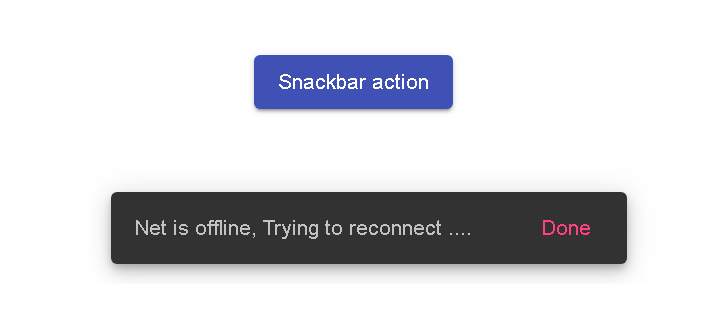
Duration
Duration is how much time the snack bar should be shown to the user before close.
By default, the snack bar is without duration, which means the snack bar is not closed until the page is refreshed. The open method has a third parameter - duration which configured time in milliseconds
<button
mat-raised-button
color=" primary"
(click)="showSnackbarDuration('Net is offline, Trying to reconnect in 5 seconds....','Done','5000')"
>
Snackbar Duration
</button>
provided duration for an open method of a snackbar object in component code
showSnackbarDuration(content, action,duration) {
this.snackBar.open(content, action,duration);
}
Snackbar disappears from the page after 5000 milliseconds or 5 seconds
Snackbar Position
All the above examples are displayed at the bottom of the page. This example talks about how to position the snack bar horizontally and vertically.
We can customize the Position using verticalPosition
and horizontalPosition
configuration to the open()
method
showSnackbarTopPosition(content, action,duration) {
this.snackBar.open(content, action, {
duration: 2000,
verticalPosition: 'top', // Allowed values are 'top' | 'bottom'
horizontalPosition: 'center', // Allowed values are 'start' | 'center' | 'end' | 'left' | 'right'
});
}
<button mat-raised-button color="primary" (click)="showSnackbarTopPosition('Net is offline, Trying to reconnect in 2 seconds....','Done','1000')">
Snackbar Top Position</button>
</div>
panel class custom CSS styles - color, font
We can still customize the UI of a snack bar panelClass
configuration parameter
The below example displayed a snack bar with customized CSS styles on clicking the button.
The button is created with a click event with all the required content
<button
mat-raised-button
color="primary"
(click)="showSnackbarCssStyles('Net is offline, Trying to reconnect in 2 seconds....','Close','4000')"
>
Snackbar CSS Styles
</button>
In component typescript,
Provided panelClass configuration in the third parameter of open()
method panelClass contains CSS class selector
showSnackbarCssStyles(content, action, duration) {
let sb = this.snackBar.open(content, action, {
duration: duration,
panelClass: ["custom-style"]
});
sb.onAction().subscribe(() => {
sb.dismiss();
});
}
In the component, CSS file, Declared the class selector by changing the background color and font color.
DOM for Snackbar is not a child element of the component being called, so because of this reason, `ng-deep is used if the class selector is being used.
component.css
::ng-deep .custom-style {
background-color: brown;
color: white;
}
Another option is to configure the selector in a global style CSS file as the snack bar got access to the main DOM element.
style.css:
.custom-style {
background-color: brown;
color: white;
}
And the output is shown in the browser
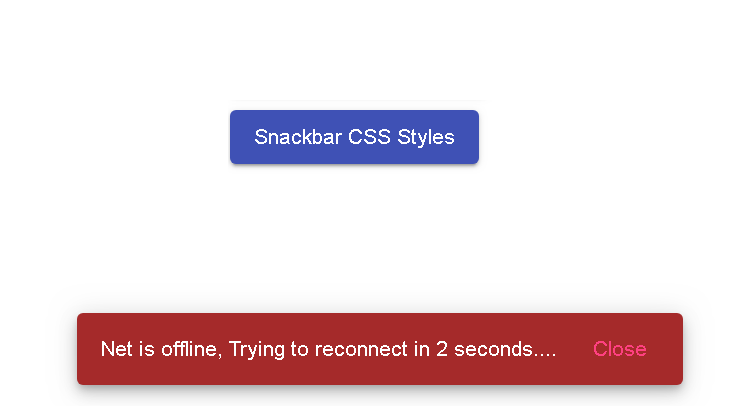
Passing data with the openFromComponent method
There is a data configuration to the open method.
we can pass the data from called component to the displayed snack bar component
This will be very useful when REST API data is consumed using reactive javascript libraries RXJS and displayed the data in it Created basic-snackbar.component
Here is the basic-snackbar.component.html template code for displaying the snackbar data passed from the caller component. Provided dismiss button on clicking on it, the dismiss()
method is called to close snackbar.
<div>
<div>{{data}}</div>
<div class="dismiss">
<button mat-icon-button (click)="sbRef.dismiss()">
<mat-icon>Dismiss</mat-icon>
</button>
</div>
</div>
Here is the basic-snackbar.component.ts typescript code
import { Component, OnInit, Inject } from "@angular/core";
import {
MatSnackBarRef,
MAT_SNACK_BAR_DATA
} from "@angular/material/snack-bar";
@Component({
selector: "app-basic-snackbar",
templateUrl: "./basic-snackbar.component.html",
styleUrls: ["./basic-snackbar.component.css"]
})
export class BasicSnackbarComponent implements OnInit {
constructor(
public sbRef: MatSnackBarRef<BasicSnackbarComponent>,
@Inject(MAT_SNACK_BAR_DATA) public data: any
) {}
ngOnInit() {}
}
Following is a caller component code app.component.html
snack bar.openFromComponent() accepts a component of another component, data is one of the parameters being passed to it.
data = "This is an example for passing data";
showBasicComponent(message: string, panelClass: string) {
this.snackBar.openFromComponent(BasicSnackbarComponent, {
data: this.data,
duration: 10000
});
}
created a button on which method is called on clicking it.
<button mat-raised-button color="primary" (click)="showBasicComponent()">
Snackbar passing data
</button>
You can check more Material snackbar🔗
Stackblitz complete example
You can check the complete working code of this tutorials