Angular Material list tutorial|mat-list examples
This post covers the Angular material list tutorial
and examples.
The Angular material list is a container element for UI elements that have been displayed inside it. Following UI components can also be wrapped with it.
- Cards
- Checkbox
The basic Material List UI component contains the following.
- List container is a required parent container for holding a group of elements or list items
- list item contains list item as a child element
In this blog post, List out the various examples of usage of the material list using angular material.
This post doesn’t cover how to create an angular application, And it covers adding material list code to the angular application.
Angular material list basic example
a material list is a UI element to display the list of objects in a list container.
matList
is a selector used to display the material style of the list on UI pages.
list related components are inbuilt in the angular module in the form of MatListModule
.
Import angular material list module
All you need to do is import the MatListModule
module into your application. You can add import in your component, app.module.ts, or shared common module.
I am adding this module to the app.module.ts file.
import { NgModule } from "@angular/core";
import { MatListModule } from "@angular/material/list";
@NgModule({
imports: [MatListModule],
declarations: [AppComponent],
bootstrap: [AppComponent],
providers: [],
exports: [],
})
export class AppModule {}
Now, the application has access to the following components and directives of MatListModule
available in components and modules.
- mat-list,matList selectors, etc…
- mat-action-list and mat-list-item components
- matList directives
What is the next step? We’ll look at some examples of how a material list can be used.
material list of items example
We are going to create a basic list of elements in angular using the mat-list
component.
Here is a basic component
- mat-list is a parent tag to make it list, configured role as list
- It contains
mat-list-item
as a child, assign a role with listitem
basic-list.component.html
<h3>Basic Material List</h3>
<mat-list role="list">
<mat-list-item role="listitem">INDIA</mat-list-item>
<mat-list-item role="listitem">USA</mat-list-item>
<mat-list-item role="listitem">GERMANY</mat-list-item>
</mat-list>
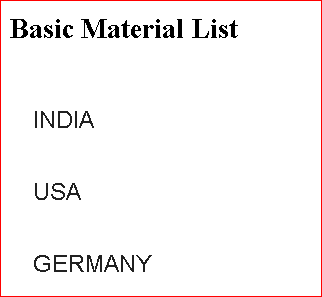
The material list also provides a navigation list, Navigation links are links that are clickable using anchor tags.
Angular template HTML component
<h3>Basic Material Navigation List</h3>
<mat-nav-list>
<a mat-list-item [href]="link.url" *ngFor="let link of links">
{{ link.title }}
</a>
</mat-nav-list>
Angular typescript component
import { Component, OnInit } from "@angular/core";
@Component({
selector: "app-basic-list",
templateUrl: "./basic-list.component.html",
styleUrls: ["./basic-list.component.css"]
})
export class BasicListComponent implements OnInit {
public links: Array<any> = [
{ title: "book1", url: "/basic" },
{ title: "book2", url: "/basic1" },
{ title: "book3", url: "/basic2" },
{ title: "book4", url: "/basic3 " }
];
constructor() {}
ngOnInit() {}
}
Output:
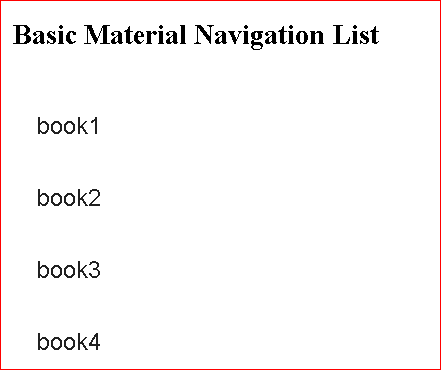
Conclusion
To Sum up, You learned a basic example of an Angular material list with cards and checkboxes.