Angular - How to add Read more/less button/link with example
In this tutorial, we are going to explain about reading more or fewer links in Angular with examples.
It is a sample example read more buttons or links with All Angular version.
When you have a long text paragraph displayed on the HTML page, you need to truncate the paragraph with a read more/less link.
Multiple ways of long text can be truncated with height or limiting the text.
For example, if you have long text displayed as shown below
<div>
This is long text replaced with reading more/less link example in the Angular
application paragraph text is shown here
</div>
<anchor></anchor>
implement Read more or less button link with size height
Here are the steps to implement
- Added ngClass directive to long text div,
ngClass
directive allows you to add CSS class dynamically based on angular expressions. - In the controller, declare the isReadMore boolean value in typescript code with a true default value
- Added button class, with click event method (showText) attached, and button text is conditional expression based on isReadMore value- ie Read More/Less
- In showText, assign with an inverse value of isReadMore
- In component CSS, added
limitTextHeight
which limits div height to 20px and overflow:hidden.
The following example clicks on the button, limits long text with height to 20px.
Here is a complete example code
Html template code - app.component.html
<h1>Angular Read More| Read less button link example</h1>
<div [ngClass]="{'limitTextHeight': isReadMore}">
This is long text replaced with reading more/less link example in Angular
application paragraph text shown here continue THis is long text replaced with
reading more/less link example in Angular application paragraph text shown
here continue continue This is long text replaced with reading more/less link
example in Angular application paragraph text shown here continue THis is long
text replaced with reading more/less link example in Angular application
paragraph text shown here continue continue THis is long text replaced with
reading more/less link example in Angular application paragraph text shown
here continue THis is long text replaced with reading more/less link example
in Angular application paragraph text shown here continue continue
</div>
<button type="button" (click)="showText()">
{{ isReadMore ? 'Read More': 'Read Less' }}
</button>
App.component.ts
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
isReadMore = true;
showText() {
this.isReadMore = !this.isReadMore;
}
}
App.component.scss
.limitTextHeight {
height: 20px;
overflow: hidden;
}
here is the output
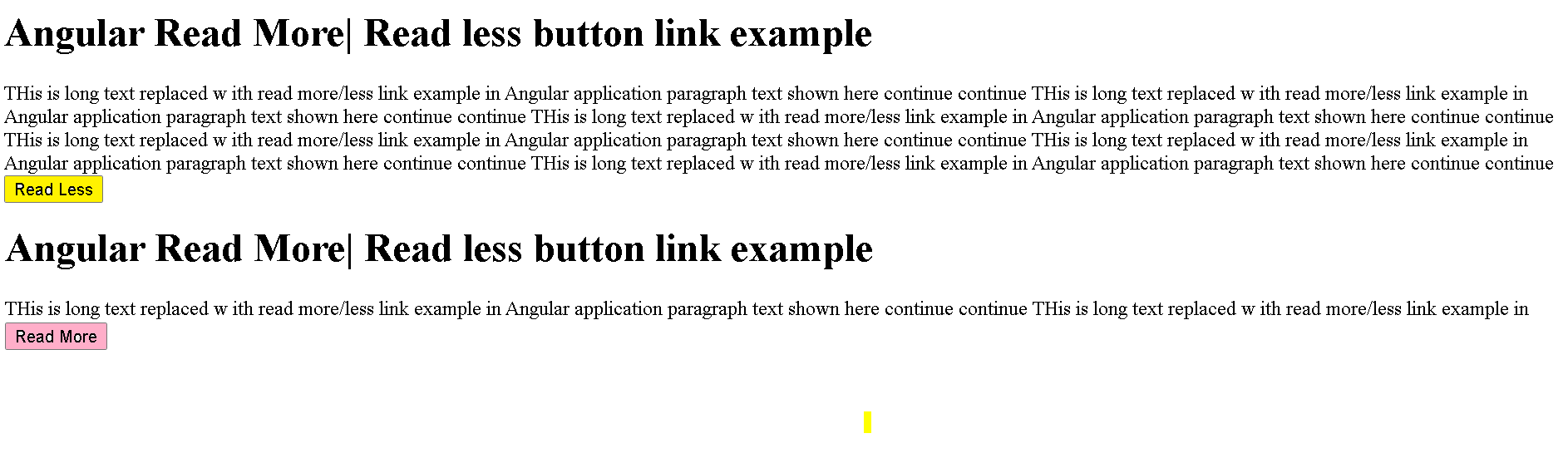
Shortened long text with show more button
This example talks about limiting long text with show more button links on angular pages.
For example, long text displayed as follows
an example of shortened long text with a button link
Adding a link to the button
And output expected is
an example for shortened long text with a button (Read More)
Complete example code HTML template contains the logic for dynamically limiting the long text with short text and the Read More link.
- Div is defined by displayed long text
- long text is limited to 50 characters
- based on boolean value-added Read More link using ngIf directive and click event
<div>
{{(readMore) ? longText : longText | slice:0:50}}
<span *ngIf="!readMore">...</span>
<a href="javascript:;" *ngIf="!readMore" (click)="readMore=true"
>[Read More]</a
>
</div>
Controller code is
- Declared ReadMore boolean in the typescript code with default true value
- And also long text is defined with a variable
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
Readmore = false;
longText = `This is long paragraph text containing several words continued. An example of implementing dynamically limit long text`;
}
here is the output

Please subscribe or comment for any new questions and new posts.