How to disable the button for invalid form or click in Angular?
In this blog post, you’ll learn the Angular Button disable for form invalid
- Form invalid form validation
- After clicking submit
First, create an application using Angular CLI, This post does not cover the creation of an application created from scratch.
In any application, You have a user form that contains input fields and submit button. Input fields have validation like required
or email
or custom validation
We have a Submit button with disabling initially. Once form validation is valid, the Button should be clickable.
This example does the following things.
- Submit button is enabled for form validation to be passed
- submit button is disabled if the form contains validation errors.
Button disable form invalid validation Example
In Angular, we do form handling validation using the below two approaches.
- template-driven forms
- Reactive form
FormModule
is required for angular template-driven form validation ReactiveFormsModule
is required to import into an application for reactive form validation
Import form FormsModule, ReactiveFormsModule in application modules
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { FormsModule, ReactiveFormsModule } from "@angular/forms";
import { AppComponent } from "./app.component";
import { HelloComponent } from "./hello.component";
@NgModule({
imports: [BrowserModule, FormsModule, ReactiveFormsModule],
declarations: [AppComponent, HelloComponent],
bootstrap: [AppComponent],
})
export class AppModule {}
Button disable template-driven form invalid
FormsModule
is already imported as per the above step, this enables us to use all template driven features in our application Create a component using the Angular CLI command
Define the template form variable for the form element In our example, the myForm variable is declared, and updated the form tag with the syntax below
<form #myForm="ngForm"></form>
W
declared for two-way binding passing from/to component to model and also gives control as invalid or value changed
In the button, myForm.invalid returns true if the input is empty, disable becomes true
you can also !myForm.valid in place of myForm.invalid
<form #myForm="ngForm">
<div class="form-group">
<label>Name</label>
<input
[(ngModel)]="name"
#nameInput="ngModel"
name="name"
type="text"
required
/>
<button [disabled]="myForm.invalid">Submit</button>
</div>
</form>
Button disable reactive driven form invalid
In typescript component reactiveForm variable is declared of type FormGroup
this is initialized with a null value and validator configured of the required type
import { Component, OnInit } from "@angular/core";
import { FormGroup, FormBuilder, Validators } from "@angular/forms";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent implements OnInit {
reactiveForm: FormGroup;
constructor(private builder: FormBuilder) {}
ngOnInit() {
this.reactiveForm = this.builder.group({
age: [null, Validators.required],
});
}
}
In the template HTML component,
the form tag is updated with the formGroup variable name - reactiveForm
and input is added with formControlName with the name of the field.
This will be given two ways to binding and form valid and invalid properties.
<h3>Submit button disable example Reactive Form</h3>
<form [formGroup]="reactiveForm">
<input placeholder="Enter your Age" formControlName="age" />
<button type="submit" [disabled]="reactiveForm.invalid">Submit</button>
</form>
<br />
<div>reactiveForm.valid= {{ reactiveForm.valid }}</div>
<div>reactiveForm.invalid = {{ reactiveForm.invalid }}</div>
<div>reactiveForm.status = {{ reactiveForm.status }}</div>
<div>reactiveForm.disabled = {{ reactiveForm.disabled }}</div>
And output is
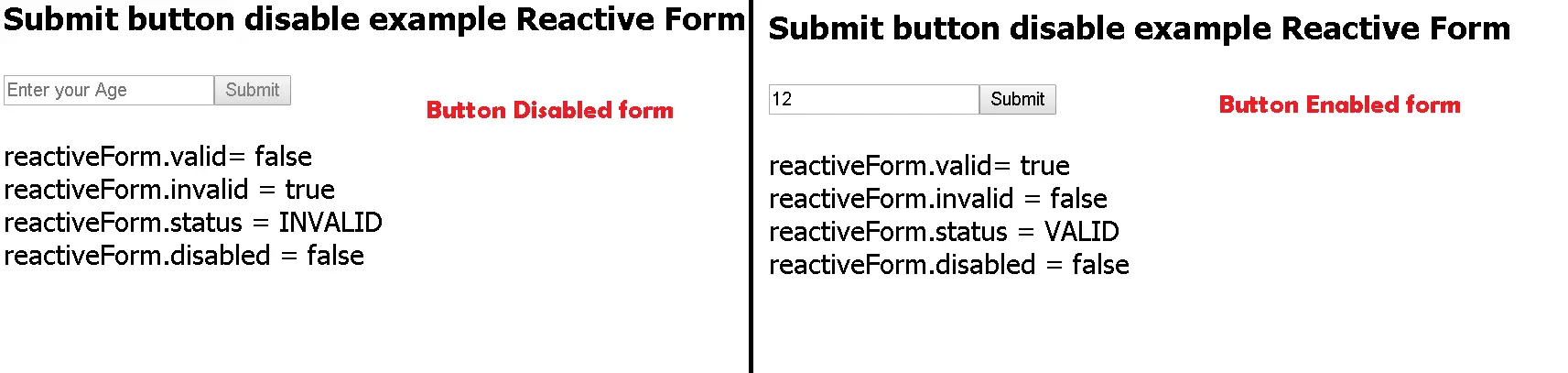
stackblitz example
You can check to complete the latest working code angular material divider stackblitz🔗
Can’t bind to ‘formGroup’ since it isn’t a known property of ‘form’ error
This error is that formGroup is not able to find the corresponding module in your component.
The Fix is to please import FormsModule
, and ReactiveFormsModule
in your app.module.ts