5 ways to use Input blur event in Angular| Angular blur event tutorials
In this tutorial, We will learn how to use input onBlur
event in Angular
First, what is the onBlur event
? where is this being used in Angular?
onBlur
is a javascript event, fired when the input element lost its focus.
You can see my previous about Angular button click event example Other versions available:
It is helpful while doing form validations.
In Plain HTML pages, the onBlur element is added to the input element.
html onblur Syntax
<inputformelement onBlur="eventname"> </inputformelement>
input form elements can be one of these.
- text
- textarea
- checkbox
- radio
- button
- label In Angular, This will be used to do form validation i.e client or server-side. Like in HTML, Angular will have a blur event instead of onBlur.
We are going to explain Different examples using blur event in Angular
How to use blur event in Angular?
blur event
can be captured using event binding in Angular. Event binding is used to attach the event to the DOM element. Syntax is
(event binding)
This is one-way binding
to bind the data from the HTML template to the Typescript controller.
The input element is added with the blur
attribute as seen below
<input type="text" (blur)="blurEventMethod($event)" />
blurEventMethod is called once the focus is lost from this input. $event
is passed to the blur method.
<div>
<h2>{{ heading | titlecase }}</h2>
<input type="text" (blur)="blurEvent($event)" />
<div>Output is {{enteredEmail}}</div>
</div>
The declared method which is being called upon focus lost from HTML. Input value is read using event.target.value
.
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
heading = "angular blur tutorial with examples";
enteredEmail: string = "";
blurEvent(event: any) {
this.enteredEmail = event.target.value;
console.log(this.enteredEmail);
}
}
Two-way binding blur in Angular
In Angular, Two-way binding is achieved with the ngModel
attribute. ngModel
attributes binding from the controller to HTML and HTML to the controller
For two way binding work, please include formsmodule
in the application module.
<input type="text" [(ngModel)]="email" (blur)="blurEvent()" />
Declare binding variable in Typescript Component, Write a function which already configured in HTML, You can directly access the variable
enteredEmail: string = '';
email: string = '';
blurEvent(event: any) {
this.enteredEmail = this.email;
console.log(this);
}
Service call while onBlur event
Input blur template-driven form validation
First, Template-driven form
is one of angular form handling, allowing you to do form validation These usengModel
and ngModelOptions
, local references with hash.
This works from the angular 5 version onwards.
The template input form component has no form method defined.
- input element has a
ngModel
directive to have two-way binding ngModelOptions
directive was added to change the behavior ofngModel
- here email model is bound to
blur
event ofupdateOn
- emailerror reference created to catch errors, true - email is empty, false - email already entered
- This will enable email required validation displayed to the user
<div>
<h2>{{ heading | titlecase }}</h2>
<input
type="text"
[(ngModel)]="email"
[ngModelOptions]="{ updateOn: 'blur' }"
#emailerror="ngModel"
required
/>
<div *ngIf="!emailerror.valid">
<span style="background-color: red">Email is required </span>
</div>
<button type="submit ">Submit</button>
</div>
template form component controller has to declare the ngModel
attribute value email.
import { Component } from "@angular/core";
import { FormControl, FormGroup, Validators } from "@angular/forms";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
heading = "angular blur validation with examples";
email: string = "";
constructor() {}
}
input blur event reactive form validation with updateOn
Reactive forms is another form approach to handling input data, It uses FormGroup
and formControlName
.
form component declared emailForm of type
FormGroup
emailForm instance is created with FormGroup, contains validators and the
updateOn
ofblur
event.emailForm is bounded to the template form defined.
import { Component } from "@angular/core";
import { FormControl, FormGroup, Validators } from "@angular/forms";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.scss"],
})
export class AppComponent {
heading = "angular blur validation with examples";
emailForm: FormGroup;
myusername: string = "";
constructor() {
this.emailForm = new FormGroup({
email: new FormControl("", {
validators: Validators.required,
updateOn: "blur",
}),
});
}
}
App component HTML for displaying input form as well as a validation error
Declared form with
formGroup
directive to bind the emailForm to FormGroupAdded
formControlName
to input elementThere is a blur event configured in-app component HTML
display errors if form control is not valid and touch event passed
<div>
<h2>{{ heading | titlecase }}</h2>
<form [formGroup]="emailForm">
<input type="text" formControlName="email" />
<div
*ngIf="
!emailForm.controls['email'].valid &&
emailForm.controls['email'].touched
"
>
<span style="background-color: red">Email is required </span>
</div>
</form>
<button type="submit ">Submit</button>
</div>
And output displayed in the browser is
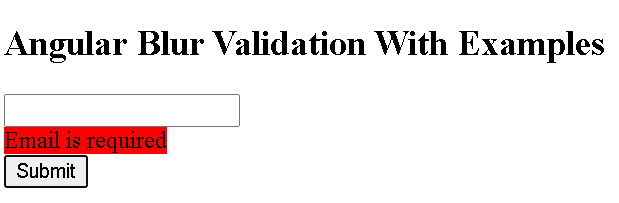
Conclusion
In this tutorial, you learned the following things
- blur event usage in angular.
- blur event with ngModel
- blur validation in template form inputs,
ngModelOptions
- reactive form validation using
blur
.