How to generate GUID in Angular 15| Typescript UUID example
Learn how to generate Unique ID - GUID, UUID in Angular with examples.
Please see my previous posts on GUID with examples.
How to generate a Unique UUID in the Angular app
Unique Identifier
generation is a requirement in any programming language. It contains 128 bits in size separated by a hyphen with 5 groups.
Angular is an MVC framework based on Typescript.GUID
and UUID
generate 128 bits of the implementation.
This blog post works on all angular versions including the latest versions.
This article is updated and compatible to work with the latest Angular 15 versions.
There are many ways we can generate GUID in the Angular application.
Following are different npm packages available for GUID.
This example also works ionic 5
application.
The example talks about the following things.
- How to generate GUID in typescript.
- How to generate UUID in angular component
Generate UUID using angular2-uuid npm package
First, install the angular2-uuid
npm package using the npm install
command.
npm install angular2-uuid --save
It installs and creates an angular2-uuid
dependency in node_modules and added one entry in the dependency of package.json.
{
"devDependencies":{
"angular2-uuid":"1.1.1";
}
}
Next, Import UUID module in angular component
Once angular2-uuid
is installed to the angular application successfully, The next step has to import UUID
into the angular component. app.component.ts:
import { Component } from "@angular/core";
import { UUID } from "angular2-uuid";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
uuidValue: string;
constructor() {}
generateUUID() {
this.uuidValue = UUID.UUID();
return this.uuidValue;
}
}
HTML Template generation:
Here is a sequence of steps for HTML
- Created button in HTML component
- on clicking the button, it calls a function in the angular component.
UUID.UUID()
generates unique identifier app.component.html You can see my previous about Angular button click event example
<div style="text-align:center">
<h1>Angular Typescript UUID generation</h1>
<button (click)="generateUUID()">Generate UUID</button>
<h2>{{uuidValue}}</h2>
</div>
Output:
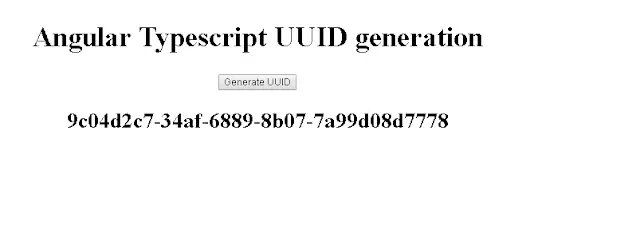
How to Generate uuid in typescript example
To generate UUID in typescript, Please follow the below steps First, Install the uuid
npm library in a project
npm install uuid
- Next, import
uuid
into the typescript - generate unique UUID using
uuid.v4()
Following is a Typescript UUID Class for generating a UUID code
import * as uuid from "uuid";
class UUID {
constructor (public uuid: string) {
this._uuid = uuid;
}
private _uuid: string;
public toString(): string {
return this.guid;
}
// Static
static generateNew(): uuid {
return uuid.v4();
}
}
And also you can pass UUID in methods with parameters in typescript
public toString(uuidvalue: uuid): string {
console.log(uuidvalue);
}
What is GUID typescript?
GUID is a unique code generated for 16 digits using Hexa digital characters. GUID is an npm library to generate 16-digit code, It is used in applications to identify a unique row in tables or documents.
Is GUID same as UUID?
GUID and UUID are both the same and their names are different. You can use and replace
What is GUID in angular?
GUID is a unique identifier for identifying entities in a class or interface field. It is used to represent a unique primary key in the database that retrieved in JSOn format in the Angular application
What is the GUID default value?
GUID is a 16-digit number generated using some algorithm.
The default value is 0000-00000-00000-00000
What is the difference between UUID and GUID?
GUID is a Globally Unique identifier, and UUID is a universally Unique identifier. Even though names are different, but generate the same format of 16 digits. They can be used for exchange in Node applications. It represents primary unique keys in the database. MongoDB contains GUID to represent the unique document.
How do you generate a random UUID in TypeScript?
To generate UUID in typescript, Please follow the below steps First, Install the uuid
npm library in a project
npm install uuid
- Next, import
uuid
into the typescript. - generate unique UUID using
uuid.v4()
Conclusion
In this tutorial, Learned how to generate UUID and GUID in the Angular app.