Angular Button Click Event examples - Binding Event
In this blog post, You learn How to add a button click event by Clicking submit button, and How to get value from input with an example.
You can also check my post on Angular Anchor Click example
Angular Button Click Event binding
In any web application, the user clicks the button for one of two reasons: to submit the form or to navigate from one page to another. HTML page generates an event for user click.
You should handle some logic for form submission or navigate to another page when the button is clicked.
Every HTML control like button or input type has an event that does fire during the user action. When the button is clicked, DOM Event onClick
event is triggered, You have to handle code to handle this DOM event.
Then, How a button click works in the Angular application? What is Angular event binding
? In the Angular application, displaying button code is placed in the HTML template page i.e view.
The trigger event is placed in the typescript component class. So user event information will be passed from view to component class. This is called Angular Event binding
This post covers the following examples with a detailed explanation
- Angular Button Click event example
- Get input value on button click event
angular button onclick function example
Let’s add changes to the Angular component.
In Html template component- app.component.html:
- component template HTML file Created with an HTML input button
- Added
click
event to a button with event binding syntax i.e bracket() symbol - the event name is the name of the function placed inside the bracket.
- This function is called when the button is clicked.
- Next, displayed the status using
angular two-way binding
that passes the data from/to view to the component. - Data displayed using either
ngModel
or interpolation - [object Object] syntax. - Used displayed interpolation syntax to display data
<div style="text-align:center">
<h1>Angular Button Click Event Example</h1>
<button (click)="clickEvent()">Click Me</button>
<h2>{{msg}}</h2>
</div>
Event handling in Component:
In the component class,
- You have to define the method for handling click events. This method is called always when a user has clicked a button.
- defined msg variable of type string.
- On clicking button event, the msg variable is updated with the status “button is clicked”.
app.component.ts:
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
msg: string;
constructor() {}
clickEvent() {
this.msg = "Button is Clicked";
return this.msg;
}
}
Output:
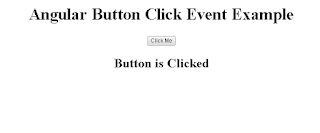
How to get Input text value on button click event example
This example explains about display input value on the clicking button.
In the Html template,
- Added
click
event to the button and provided the name of the function inside it - Input is defined with the
ngModel
attribute which binds the value from view to component or component to view - Displayed the value typed in the text box using
interpolation
syntax
<div style="text-align:center">
<h1>Angular Button Click Event Example</h1>
<button (click)="clickEvent()">Click Me</button>
<input type="text" [(ngModel)]="msg" />
<h2>{{msg}}</h2>
</div>
Typescript Component class
on click event updates the value to a new variable which is displayed in HTML using interpolation syntax {{}}
import { Component } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent {
msg: string;
msg1: string;
constructor() {}
clickEvent() {
this.msg1 = this.msg;
}
}
Conclusion
You learned two things. Firstly, the button with click event example in Angular, Secondly, how button event binding works in Angular applications.
To summarize, It is easy to handle the click events in the Angular button.