Typescript Interfaces - Learn in 5 mins with examples
- Admin
- Mar 6, 2024
- Typescript
In this Blog Post, learn the Interfaces tutorials in typescript. You can also check Typescript final keyword
It is one of the concepts of Object-Oriented Programming. Other concepts are
TypeScript Interface Basic Tutorial
Interfaces
in TypeScript are similar to those in other programming languages like Java. They do not provide any implementation but only abstract methods.
An interface serves as a contract or rule for classes that implement it. When the interface is compiled to JavaScript, no code is generated, meaning there is no interpretation of runtime code. Instead, TypeScript provides compile-time type safety, as no code is generated in JavaScript due to absence of type checking.
Interfaces allow developers to extend and write their own implementations, containing properties, member variables, and function or method definitions.
How to Declare an Interface in TypeScript
Interfaces
can be created using the interface keyword, containing member variables and method headers surrounded by braces.
Here’s the syntax for interface declaration:
interface InterfaceName {
// member variables
// methods without implementation
}
Example of an interface:
interface IEmployee {
userId: number;
username: string;
roleName: string;
}
TypeScript Type Safety Example
Type checking is one of the advantages TypeScript offers over JavaScript.
Here’s a simple example demonstrating type safety.
Created interface which has member variable only, the name is a string type. Created a class
that implements this interface
by providing a constructor with the public of the interface
variable.
Let’s see an example of how type safety works in typescript.
interface IEmployee {
name: string;
}
class Employee implements IEmployee {
constructor(public name: string) {}
}
let validEmp: Employee = new Employee("frank"); // valid Object
let invalidEmp: Employee = new Employee("kiran", "abc"); // invalid Object
let invalidEmp1: Employee = new Employee(1); // invalid Object
validEmp
is a valid class object that implements the interface and is initialized with a string parameter.
invalidEmp
throws a compile-time error- Expected 1 argument, but got 2. constructor
accepts only one string, but we are passing two strings.
invalidEmp1
- throws a compile-time error - Argument of type ‘1’ is not assignable to a parameter of type ‘string’. The reason is creating an object with numeric whereas it accepts values of type string only.
The advantage for developers with type safety is avoiding issues with types at runtime. Please see the below screenshot of how code generates in typescript.
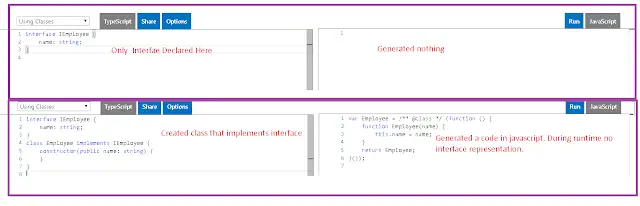
How to declare different member variable types in typescript?
In the below section, we will see various use cases for declaring different variable types.
Interface Optional Types example
Sometimes, when the interface
is passing to function
, the caller of the function will not send all the required parameters. Optional member variables are declared with a question mark(?) at end of the variable.
IShape
interface has two optional parameters and another function
has an interface parameter.
Calling the function with interface contains works either with zero parameters, one parameter, or two parameters.
interface IShape {
length?: number;
width?: number;
}
function createShape(shape: IShape): any {}
let shape = createShape({ length: 10 }); //valid
let shape1 = createShape({}); //valid
let shape2 = createShape({ length: 10, width: 20 }); //valid
Interface Readonly Types example
This is one more way of declaring an interface definition to give more flexibility to developers.
The Readonly
keyword is used with a member variable and declared before the variable.
the member variable is initialized during object creation.
Once values are assigned, variables will not be modified at any cost.
Once values are assigned, if the value is assigned to the legs property, It compiles, whereas assigning to ears will not compile as this is a readonly
property
interface Animal {
readonly ears: number;
legs: number;
}
let animal: Animal = { ears: 2, legs: 4 };
animal.ears = 1; // this will not compiles
animal.legs = 4; // this compiles fine
Function Interface Example
Function types can be declared with interfaces.
This is like Function header + arguments+ type.
The calling Function should have the same number of arguments with the name that might be different, the type should be the same and the return type should be the same Declaration and calling the function.
interface Vehicle {
(name: string, wheels: number): string;
}
let car: Vehicle;
car = function (n: string, w: number) {
return n;
};
You can omit function types string, number as typescript can type infer based on the values passed.
Index types signature in interface Example
We can also declare interface parameters with index types
.
When do we use index types ?. Simple, if the interface contains a lot of member variables of the same type, we will use this.
This is a kind of array declaration in interfaces.
Number array has an index signature, with a numeric index that starts from zero.
Index signature only applicable to string and number datatype
interface numberArray {
[index: number]: number;
}
let arr: numberArray;
arr = [11, 5, 8];
console.log(arr[0]); // returns 11
Interfaces inheritance examples
Inheritance
is one of the important concepts in object-oriented programming.
It allows for extension the of common things and enables a reuse mechanism.
Inheritance can be achieved using implements and extends keywords.
Using extended example
- inheritance example This is single inheritance as a super class only extended by one class
interface Animal {
legs: number;
}
interface Lion extends Animal {
eat: string;
}
var lion = {};
lion.legs = 4;
lion.eat = "nonveg";
console.log("Number of legs=" + lion.legs + " Eat type= " + lion.eat);
JavaScript generated code
var lion = {};
lion.legs = 4;
lion.eat = "nonveg";
console.log("Number of legs=" + lion.legs + " Eat type= " + lion.eat);
the output of the above code is
Number of legs=4 Eat type= nonveg
Multiple inheritances are directly not possible, But you can achieve them using implements or indirect inheritance as below
superclass1-->superclass2-->childobject
Using implements interface example
With the implements
keyword, you can implement multiple interfaces.
Please see the below example. This example created two interfaces. Created class by implementing two interfaces. You can achieve multiple inheritances like this
interface ISuper1 {
method1(): void;
}
interface ISuper2 {
method2(): void;
}
class Child implements ISuper1, ISuper2 {
method1() {
console.log("Method 1");
}
method2() {
console.log("Method 2");
}
}
var child = new Child();
child.method1();
child.method2();
interface in typescript advantages
There are many advantages to interfaces
- Provides type safety with type checking
- Supports multiple inheritances
- Promotes loose coupling between objects
- Allows for abstraction
Relation Between Interfaces and Classes
Interfaces define a contract that classes must adhere to by providing implementations for methods.
For example, Define an interface for DownloadData.
interface DownloadData {
download(): void;
}
Provides a class for downloading XML and json
class DownloadXml implements DownloadData {
download() {
console.log("download xml data");
}
}
class DownloadJson implements DownloadData {
download() {
console.log("download JSOn data");
}
}
The class implements an interface and has to provide an implementation for a method.
Conclusion
In conclusion, this tutorial covers interfaces in TypeScript extensively with various examples.