Three.js Basics introduction - 3d Javascript library
- Admin
- Dec 31, 2023
- Javascript
Three.js Basic tutorial
Learn the three.js
library basics with an example.
Three.js
javascript opensource library to generate and render 3d content into browsers. This API provides rendering 3d and 2d images on the HTML page using canvas, SVG, and WebGL capabilities. It renders the content using webGL🔗() into the browser if supports it. If the browser is not supported, render content using html5 canvas or SVG features.
It is complex to fully feature 3d content for Generating and rendering it as we have to write a code using WebGL or write a code. This library allows developers to simplify the rendering 3d content.
Three.js Advantages for developers
- Complete independent Javascript API and no dependencies, need WebGL support in the browser as most of the latest browsers on web and mobile support.
- All Major latest browsers on desktop and mobile.
- It creates 3d models with javascript API.
- Supports ALl 3d models - Geometry, Lights, Materials, cameras and also provides - export/import of objects.
- Community support.
- No Plugin is required, and no dependency on the client-side
Installation and setup
There are multiple ways we can integrate the three.js library into applications Using script tag or CDN file:
Get the library by downloading from here🔗 into the local machine. Include it in the script tag of your head HTML tag. It is a simple way to use this library.
Another way is to use CDN library URL. In both cases, please use the minified version.
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r83/three.min.js"></script>
<script src="lib/three.min.js"></script>
Using nodejs npm package It is another process of using three.js in a node environment. First, you need to install this library and import it as a module.
npm install --save three
Nodejs Example usage
We already installed it via the npm package manager. Next, is to import the three.js module, using require() function, all the objects are available in the code. You can also import the module using es6 syntax as below.
var THREE = require("three");
or;
import * as THREE from "three";
import { Scene } from "three";
var mainScene = new THREE.Scene();
Once imported, you can access all the objects in this library. Hello World Example In this tutorial, We walk through the simple hello world example, the usage of various objects explained. Here is a sample code using this library
<!doctype html>
<html>
<head>
<title>Basic Three.js App</title>
<style>
body {
margin: 0;
}
canvas {
width: 100%;
height: 100%;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r83/three.min.js"></script>
<script>
// Javascript will go here.
</script>
</body>
<script>
var scene = new THREE.Scene();
var camera = new THREE.PerspectiveCamera(
25,
window.innerWidth / window.innerHeight,
0.1,
1000,
);
camera.position.z = 4;
var renderer = new THREE.WebGLRenderer({ antialias: true });
renderer.setClearColor("#0ffff0");
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
var geometry = new THREE.BoxGeometry(1, 1, 1);
var material = new THREE.MeshBasicMaterial({ color: "#433F81" });
var cube = new THREE.Mesh(geometry, material);
// Add cube to Scene
scene.add(cube);
// Render Loop
var render = function () {
requestAnimationFrame(render);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// Render the scene
renderer.render(scene, camera);
};
render();
</script>
</html>
Here is the output of the above code
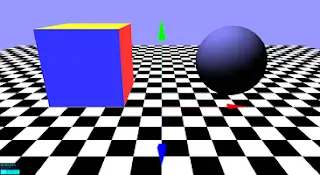
There are various global variables - rendered
, scene
, camera
. First, you need to create these objects during form/window load. Next, you need to create a Scene
object, Which holds all the objects.
var scene = new THREE.Scene();
Next is to create a WebGLRenderer
- does render the screen object to the browser.
var renderer = new THREE.WebGLRenderer({ antialias: true });
After the rendering object creates, create a camera
object which is a visible component seen on the browser.
var camera = new THREE.PerspectiveCamera(
25,
window.innerWidth / window.innerHeight,
0.1,
1000,
);
Once we have all global objects created, the Next is to add different elements, here create geometry
, and add mesh
. We created a BOX
cube that is to render which has 3d properties - width
, height
, and depth
.
var geometry = new THREE.BoxGeometry(1, 1, 1);
var material = new THREE.MeshBasicMaterial({ color: "#433F81" });
var cube = new THREE.Mesh(geometry, material);
Rendered.render()
method provides render the scene to the browser.
How to check Browser support WebGL or not? To render 3d scenes, first, we need to check whether WebGL
support is available or not in a browser. We need to if WebGL
is not supported, then default to HTML5
canvas
or SVG
capabilities. Detector code can be found from here🔗
if (Detector.webgl) {
// WebGL is supported
} else {
// webglo is not supported in the browser.
}
This library supports various types of 3d models- GLTransimission
Format, FBX
, Collada
, or OBJ
.
Browser Support
WebGL is the default rendered mechanism to display 3d models in browsers. It supports all latest below browsers that have WebGL support on Internet Explorer, Firefox, Chrome, Safar, and all browsers on android also. You have to use SVGRenderer
for older versions of the browser. CanvasRenderer
class for html5
SVG
, and Canvas
support.