Primeng Dropdown Component | Angular Dropdown list Example
In this tutorial, learn the basics of Primeng Dropdown with examples.
The ‘dropdown’ is a user interface element that displays an elements list and allows the user to select one of the elements from the list. The element can be plain strings, JSON arrays, or javascript Objects.
Primeng
has a set of rich UI Elements for Agular Framework on typescript from the Primefaces
team.
You can check my previous posts on the primeng framework.
primeng Angular dropdown features
The following is a list of In-built features supported.
- Customize the way look and feel styles
- Custom Content and label using ngTemplate
- Remote Binding with REST API
- Filtering
- Grouping
With the above features already supported, the developer’s job is easy to focus on business functionality. In addition, the completed tested component is ready to use in your application.
This tutorial works with all Angular versions.
Integration primeng dropdown into the existing angular application
This tutorial covers the Integration of the primeng dropdown into the existing angular application.
if you want to start the application from scratch, you check my previous Angular Application from scratch using primeng framework post.
First, install the primeng dependencies primeng
and primeicons
using the npm command.
npm install primeng --save
npm install primeicons --save
npm install @angular/CDK --save
The above code installs the dependencies and makes an entry in package.JSON,
primeng modules provide various components in the form of modules.
For example, DropdownModule
is an angular module that provides a dropdown or select box component.
primeicons npm module
provides icons provided by the primefaces team.
Please check this [add icons to primeng](/prime-icons-list)
post to add icon support to your module. This is an important step and if not configured correctly, icons are not visible correctly.
@angular/cdk
- This npm module is required if scrolling support needs in the dropdown.
Configure CSS styles in styles.css
@import "~primeicons/primeicons.css";
@import "~primeng/resources/themes/nova-dark/theme.css";
@import "~primeng/resources/primeng.min.css";
with the above steps, primeng npm
modules are installed successfully in your application.
Primeng provides each component in the form of angular modules. Therefore, import DropdownModule
into your application as below.
Configured DropdownModule
in the import section of your app.module.ts
.
After that, all primeng dropdown components/directives are used in your application.
import { BrowserModule } from "@angular/platform-browser";
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from "@angular/core";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations";
import { DropdownModule } from "primeng/dropdown"; // include this for dropdown support
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule,
BrowserAnimationsModule,
DropdownModule, // dropdown support
],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
export class AppModule {}
This is a one-time process per your application.
Now, you are ready to go creating the first dropdown example.
Primeng Angular dropdown example
First, Create a Simple angular basic dropdown. component using ng g c nameofcomponent
command
It creates the following files in the existing application.
- basic-dropdown.component.html
- basic-dropdown.component.ts files
- basic-dropdown.component.css
basic-dropdown.component.ts
file
It is a typescript component file component that processes logic for displaying the UI element.
Here is a sequence of steps to add typescript logic.
- First, create a list of Employee objects of type Array
SelectedItem
. - Primeng dropdown supports
SelectedItem
or a Custom array of objects. By default, It supportsSelectedItem
. - Each
SelectedItem
accepts key =label
and value=value
only. - We will cover in the next example if the object is of a custom type other than
name
andvalue
. - In Constructor, an Employee object is created and initialized with fixed values.
selectedEmployee
is an object to hold a selected dropdown value, which is a way of adding a two-way binding reference to this variable.
Here is an example component
import { Component, OnInit } from "@angular/core";
import { SelectItem } from "primeng";
@Component({
selector: "app-basic-dropdown",
templateUrl: "./basic-dropdown.component.html",
styleUrls: ["./basic-dropdown.component.css"],
})
export class BasicDropdownComponent implements OnInit {
employes: SelectItem[];
selectedEmploye: SelectItem;
constructor() {
this.employes = [
{ label: "Select Employee", value: null },
{ label: "Franc", value: 1 },
{ label: "Kiran", value: 2 },
{ label: "John", value: 3 },
];
}
ngOnInit() {}
}
In the basic-dropdown.component.html
file
The HTML template component file contains a code to display the actual component on the browser.
The p-dropdown
directive is used in this HTML file. It has an option
attribute.
Option
attribute accepts array of items, Here SelectItem
object is used, SelectItem
object contains label
and value
the selected item from the dropdown is populated using the selectedEmployee
Object of SelectedItem
.
selectedEmployee
is mapped to the component using ngModel
with two-way binding, which assigns data between the component and the Html element.
<p>Simple Dropdown Example</p>
<p-dropdown [options]="employes" [(ngModel)]="selectedEmploye"></p-dropdown>
<br />
<p>selected Employe: {{selectedEmploye? selectedEmploye.name:'none' }}</p>
And the output of the above code is
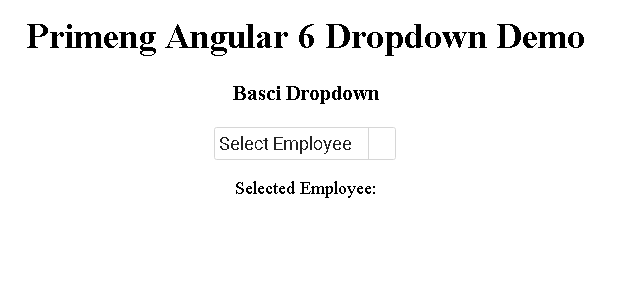
Primeng Dropdown OptionLabel example
In the above example, the Covered default format of the label
and value
of an object.
In real-time applications, The data mapped to the dropdown is in a different format.
Suppose, we have a collection of countries
with each country
containing name
and code
properties.
The OptionLabel
attribute is used to map the custom label with the data of an object.
Here is a Dropdown OptionLabel usage example
<p-dropdown [options]="countries" [(ngModel)]="selectedCountry" placeholder="Select a Country" optionLabel="name" [showClear]="true"></p-dropdown>
<p>Selected Country: {{selectedCountry? selectedCountry.name:'none' }}</p>
</div>
In the typescript component code:
countries:Country[];
selectedCountry: Country;
this.countries = [
{name: 'India', code: 'IND'},
{name: 'United States of America', code: 'USA'},
{name: 'United Kingdom', code: 'UK'},
];
Primeng Modal Driven Form Example
Model-driven form
is one of the form handlings in any angular version. You import module - FormsModule, ReactiveFormsModule
from @angular/forms
in your app module; The formControlName
element has the name of the form element. p-dropdown tag
can be changed to like as follows in HTML.
<p-dropdown
employes=""
formcontrolname="selectedEmploye"
options=""
></p-dropdown>
Primeng ng-template custom label and content
You can also use ng-template
to customize the default behavior. The default behavior is showing the label on the drop-down.
There are three templates -
selectedItem template
: Displayed Labels can be customized to display icons, and styles.item template
: customize the content in UI.group template
: customize the default behavior of the options group.
There are other features filter
- filter by display name, Group
- Group the elements under the category in the display.
Primeng Dropdown style and Icon Change
we can also customize/override the default styles and icons.
There is a predefined style to change it.ui-dropdown-trigger
It overrides the background color to green.
body .ui-dropdown .ui-dropdown-trigger {
background-color: green;
}
For example, To change the icon, you have to use the dropdownIcon
attribute typescript component, declare like as below
export class AppComponent {
customIcon = "fa fa-search";
}
using dropdownIcon
attribute of p-dropdown
tag in HTML like below
<p-dropdown
[dropdownIcon]="customIcon"
placeholder=" Filter by name"
></p-dropdown>
Primeng Important Events and properties
appendTo
: Dropdown attached to the element. here elements are a body or any element. With this, we can avoid scrollingonClick
: Event callback called on clicking the component.onChange
: Fired this event on changing the value in the dropdown.onFocus
: called when dropdown got focusonBlur
: called when the dropdown changed focus to other elements in a page from this.
How to Fix for CUSTOM_ELEMENTS_SCHEMA Angular Error
The below error we used to get during configuring dropdown in the application
Can’t bind to ‘ngModel’ since it isn’t a known property of ‘p-dropdown’.
Uncaught Error: Template parse errors:
Can't bind to 'ngModel' since it isn't a known property of 'p-dropdown'.
1. If 'p-dropdown' is an Angular component and it has 'ngModel' input, then verify that it is part of this module.
2. If 'p-dropdown' is a Web Component then add 'CUSTOM_ELEMENTS_SCHEMA' to the '@NgModule.schemas' of this component to suppress this message.
3. To allow any property add 'NO_ERRORS_SCHEMA' to the '@NgModule.schemas' of this component. ("
<h3 class="first">Basci Dropdown</h3>
<p-dropdown [options]="employes" [ERROR ->][(ngModel)]="selectedEmploye" name="selectedEmploye" placeholder="Select a Employe" optionLabel="name"): ng:///AppModule/AppComponent.html@6:33
at syntaxError (compiler.js:1016)
at TemplateParser.push../node_modules/@angular/compiler/fesm5/compiler.js.TemplateParser.parse (compiler.js:14813)
at JitCompiler.push../node_modules/@angular/compiler/fesm5/compiler.js.JitCompiler._parseTemplate (compiler.js:23992)
at JitCompiler.push../node_modules/@angular/compiler/fesm5/compiler.js.JitCompiler._compileTemplate (compiler.js:23979)
at the compiler.js:23922
at Set.forEach (<anonymous>)
at JitCompiler.push../node_modules/@angular/compiler/fesm5/compiler.js.JitCompiler._compileComponents (compiler.js:23922)
at the compiler.js:23832
at Object.then (compiler.js:1007)
at JitCompiler.push../node_modules/@angular/compiler/fesm5/compiler.js.JitCompiler._compileModuleAndComponents (compiler.js:23831)
Fixing this exception:
The reason p-dropdown
is an HTML tag defined as an angular component which is a custom HTML tag, not an html5 inbuilt tag, so we are using it in our component.
if the name of the component contains a dash(-), then we need to do follow things.
You can add CUSTOM_ELEMENTS_SCHEMA as Schemas decorator to either in component or module.
I am going to add it app.module.ts
file which applies to all components in that module.
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from '@angular/core';
bootstrap: [AppComponent],
schemas:
[CUSTOM_ELEMENTS_SCHEMA]
Conclusion
This sums up learning angular primeng dropdown examples for common issues and their fixes.