Primeng data table Filtering Sorting Paging | Angular p-table examples
In this blog post, We are going to learn the Primeng Angular 12 Data table example with tutorials.
Primeng
is an Angular open-source framework for a collection of Rich UI libraries. Primefaces
is the author of the primeng
framework.
Primeng data table in Angular
In every application, there is a requirement to display a large amount of data from the database in table form in the user interface.
UI elements such as Data tables display such data. What is the use of data table components in a web application?
For example, You have to write an admin interface for an eCommerce UI application like amazon. As an admin of the application, you can perform CRUD operations and view data in the admin panel of your eCommerce application.
So you have to use the table component and use features like search fields, filter, and showing all fields. There are three reasons to choose a table representing data on the browser.
First of All, It helps users to display paginated data when there are many records. Secondly, It provides a good experience to display records once or lazy loading. Users are visible and easily filter records on all records. Thus, the table feature has advantages for users.
We can use either the HTML table or the Custom Data table.
Primeng provides an inbuilt table that provides a lot of features (Pagination, sorting, filtering.) as described below, Hence, It helps developers to write lesser code instead of writing custom code.
Primeng data table features
It provides a rich data table UI with a lot of features.
- Pagination
- Sorting and Filtering based on columns
- Grouping columns
- Export to PDF document
- Row, Column expand, and group
- Scroll and toggle
- Context Menu
- Edit, resize, and Reorder
- Responsive
This post is updated and works in Angular 2,4,6,7,8,9,10,11,12 and 13 versions include latest versions.
Generate Angular Application with CLI
First, Check Angular CLI tool is installed or not using the ng command
If not installed, Please install the angular CLI tool using the
npm install
command below.npm install -g @angular/cli
Next, create an Angular project using the ng command
ng new primeng-datatable
This creates an Angular application with all default configurations and initial components with the project structure and installs all dependencies.
Run the project using
ng serve
Go to the project directory, and issue the commandng serve
The application starts and listens at default port 4200, Application can be accessed with ‘http://localhost:4200🔗’.
Angular CLI Project Structure
Following is the project structure which contains all required initial components and configuration files required to run the angular project.
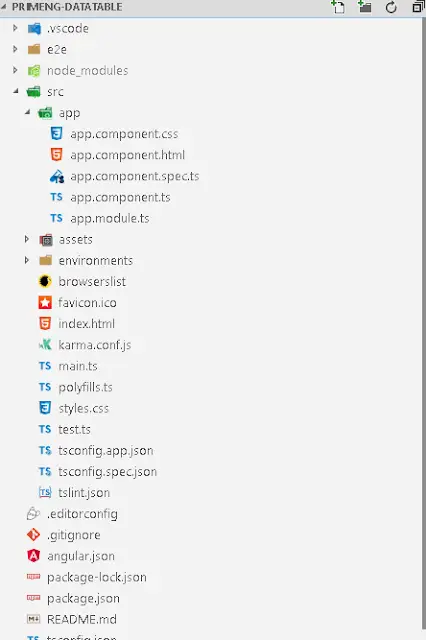
Integrate primeng npm in Angular
primeng
is available as annpm
package.First Install dependencies using the
primeng npm command
Under the project directory, run the following commands. This installs
primeng
andprimeicons
dependencies.npm install primeng --save npm install primeicons --save
Install Animation module which is a required dependency for primeng library
npm install @angular/animations --save
angular.json style configurations
Add primeng
styles
and icons
in styles
section of angular.json
.
primeng icons use prime face icons in the table.
You can check more about prime icons
"styles": [
"../node_modules/primeicons/primeicons.css",
"../node_modules/primeng/resources/primeng.min.css",
"../node_modules/primeng/resources/themes/omega/theme.css",
]
App Module changes - app.module.ts Integrate BrowserAnimationsModule
. A lot of components in primeng are required to have a dependency on the Angular animation module.
Import BrowserAnimationsModule in the main app module.
import {BrowserAnimationsModule} from '@angular/platform-browser/animations'; // added this
The next step is to import TableModule
into the application module which gives access to all primeng table components, directives, and properties
import { TableModule } from "primeng/table";
app.module.ts:
import { BrowserModule } from "@angular/platform-browser";
import { NgModule } from "@angular/core";
import { AppComponent } from "./app.component";
import { TableModule } from "primeng/table";
import { BrowserAnimationsModule } from "@angular/platform-browser/animations"; // added this
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, BrowserAnimationsModule, TableModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
Primeng Data Table Simple Example
In this section, Let’s see a basic static data-binding example
We have the User
class which has attributes id
, name
, and email
.
Let’s define a model class for User
export interface User {
id;
name;
email;
}
This example contains a data table to display a list of users’ data. The data table requires users to object in the form of an array.
Component changes:
In the angular component, get the data and store it under the User object.
import { Component, OnInit } from "@angular/core";
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
})
export class AppComponent implements OnInit {
users: User[];
cols: any[];
ngOnInit() {
this.users = [
{ id: "1", name: "kiran", email: "[email protected]" },
{ id: "2", name: "tom", email: "[email protected]" },
{ id: "3", name: "john", email: "[email protected]" },
{ id: "4", name: "Frank", email: "[email protected]" },
];
this.cols = [
{ field: "id", header: "Id" },
{ field: "name", header: "Name" },
{ field: "email", header: "Email" },
];
}
}
Template html changes
p-table
is an actual component from primeng that provides data table functionality.A list of users is bound to the value property
Html table has
caption
,header
andbody
.Primeng also provides
caption
,header
, andbody
templates.Defined templates for header and body templates, templates are used to define the content of these sections.
Each of these templates is wrapped under the
ng-template
tag.caption
template declared with ng-template withpTemplate="caption"
andcaption
is a title or caption content for a table.pTemplate="header"
- It is a header of the table. Provide a list of columns for a table and it is equivalent to thethead
tag of HTMLtable
pTemplate="body"
It is a body that contains actual data and it is equivalent oftbody
tag of HTMLtable
pTemplate="footer"
- Content for afooter
of the table and it is equivalent oftfoot
tag of HTML table.pTemplate="summary"
Display below the table with summary content.
List of objects bound to the value property of p-table. There are two ways we configure table columns.
One way is using Static Columns
.
In this, Every column is configured in the template of the header section.
<p-table [value]="users">
<ng-template pTemplate="caption"> Users List </ng-template>
<ng-template pTemplate="header">
<tr>
<th>id</th>
<th>name</th>
<th>Email</th>
</tr>
</ng-template>
<ng-template pTemplate="body" let-user>
<tr>
<td>{{user.id}}</td>
<td>{{user.name}}</td>
<td>{{user.email}}</td>
</tr>
</ng-template>
</p-table>
primeng dynamic columns with ngFor loop
Here header template is configured using an array of objects.
In the component, the column array is constructed with field and header keys. The following is an example of Dynamic column binding.
<p-table [columns]="cols" [value]="users">
<ng-template pTemplate="caption"> Users List </ng-template>
<ng-template pTemplate="header" let-columns>
<tr>
<th *ngFor="let col of columns">{{col.header}}</th>
</tr>
</ng-template>
<ng-template pTemplate="body" let-user let-columns="columns">
<tr>
<td *ngFor="let col of columns">{{user[col.field]}}</td>
</tr>
</ng-template>
</p-table>
Both of the above codes produce the same output.
Data are easily shown to users.

primeng datatable Sort column ascending
This is an example of a sorting table based on columns. p-table
tag contains sortMode="multiple"
for required column sorting with adding attribute pSortableColumn
and p-sortIcon
tag.
<p-table [columns]="cols" [value]="users" sortMode="multiple">
<ng-template pTemplate="caption"> Users List </ng-template>
<ng-template pTemplate="header" let-columns>
<tr>
<th *ngFor="let col of columns" [pSortableColumn]="col.field">
{{col.header}}
<p-sortIcon [field]="col.field"></p-sortIcon>
</th>
</tr>
</ng-template>
<ng-template pTemplate="body" let-user let-columns="columns">
<tr>
<td *ngFor="let col of columns">{{user[col.field]}}</td>
</tr>
</ng-template>
</p-table>
When a column is selected for sorting, the table level content is changed to reflect the column sorting.

primeng table Pagination example
If there is a large amount of data, it is displayed as ‘Pagination’ by displaying it in a table with 10,000 rows.
The ‘pagination’ option is available in the Primeng table.
You have to configure the below properties paginator
- true/false Enable pagination or not. rows
- Number of rows displayed on each page.
<p-table
[columns]="cols"
[value]="users"
sortMode="multiple"
[paginator]="true"
[rows]="2"
>
</p-table>

<p-table [columns]="cols" [value]="users" sortMode="multiple" #dt>
<ng-template pTemplate="caption"> Users List </ng-template>
<ng-template pTemplate="header" let-columns>
<tr>
<th *ngFor="let col of columns" [pSortableColumn]="col.field">
{{col.header}}
<p-sortIcon [field]="col.field"></p-sortIcon>
</th>
</tr>
<tr>
<th *ngFor="let col of columns" [ngSwitch]="col.field">
<input
pInputText
type="text"
(input)="dt.filter($event.target.value, col.field, col.filterMatchMode)"
/>
</th>
</tr>
</ng-template>
<ng-template pTemplate="body" let-user let-columns="columns">
<tr>
<td *ngFor="let col of columns">{{user[col.field]}}</td>
</tr>
</ng-template>
</p-table>

p-table issues and errors
when I am working for the first time, I got a lot of issues related primeng table.
Module not found: Error: Can’t resolve ‘@angular/cdk/scrolling
primeng table has a dependency on paginator that has a dependency on p-dropdown.
p-dropdown uses the latest version of the angular CDK library
- First, make sure that @angular/CDK version is installed and configured in package.json
- if noCDKplease install with the below command
npm install @angular/cdk --save
.