ES6 Default Parameters examples | ecmascript5 examples
- Admin
- Dec 31, 2023
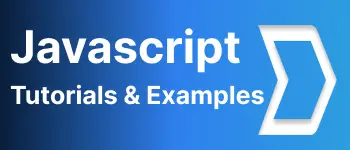
Learn the es6 features -Default arguments to the function tutorials with examples.
Before the Es6 Java version, The following is the way to handle default parameter values.
ES5 Default Parameters and previous javascript versions
Declared a convertEmployObjectToJsonString function to convert the Employee arguments to JSON string, has two arguments - name and salary
The name is a required argument
Salary is optional parameters
salary argument is an optional parameter. When the salary argument is not passed, the default value is assigned with 5000.
function convertEmployObjectToJsonString(name, salary) {
salary = typeof salary !== "undefined" ? salary : 5000;
return JSON.stringify({ name: name, salary: salary });
}
console.log(convertEmployObjectToJsonString("Frank", 6000));
console.log(convertEmployObjectToJsonString("Ram"));
console.log(convertEmployObjectToJsonString());
console.log(convertEmployObjectToJsonString(null, null));
Output is
{"name":"Frank","salary":6000}
{"name":"Ram","salary":5000}
{"salary":5000}
When convertEmployObjectToJsonString is called, the salary argument is not passed means undefined, the default value is 5000. We have added the check for salary arguments for the undefined case and if it is undefined, then the default value is initialized.
ECMAScript 2016 Default parameters
Default parameters are syntax changes to the function parameters Es6 is a new javascript version change Default parameters are declared in functional parameters initialized with a default value when the parameter to the function is undefined or not passed. Syntax:
function [name]([param1[ = defaultValue1 ][, ..., paramN[ = defaultValueN ]]]) {
statements
}
The same above example is rewritten using es6 default parameters.
function convertEmployObjectToJsonString(name, salary = 5000) {
return JSON.stringify({ name: name, salary: salary });
}
console.log(convertEmployObjectToJsonString("Frank", 6000));
console.log(convertEmployObjectToJsonString("Ram", ""));
console.log(convertEmployObjectToJsonString());
console.log(convertEmployObjectToJsonString(null, null));
console.log(convertEmployObjectToJsonString("asdfads", null));
console.log(convertEmployObjectToJsonString("Ram", "undefined"));
output is
{"name":"Frank","salary":6000}
{"name":"Ram","salary":""}
{"salary":5000}
{"name":null,"salary":null}
{"name":"asdfads","salary":null}
{"name":"Ram","salary":"undefined"}
Points to Remember
In javascript, Arguments to functions default to undefined, This allows changing the different values. When valid values are passed as arguments, Default parameters have not initialized any value, empty and null are considered valid values, Undefined, the value is not passed, the argument to the function is not passed, the undefined value is considered, the default value is initialized with default value Default arguments are evaluated at called time.
Please map the above results with these points to have a good understanding.
Function as default parameter example
Here Function next is declared inside the body of the getValues function. getValues has default parameters as a Function. The order of the execution is as follows.
Default parameters always run first. Nested Functions declared in the function body are executed next. When getValues() method is called with a function parameter, the function declaration is not available so the below code throws an error - Uncaught ReferenceError: next is not defined So the takeaway point is, Functions declared inside other function bodies are not passed as default arguments.
function getValues(value = next()) {
function next() {
return "next function method";
}
}
Fix/ Solution for ReferenceError is to move the inside function to global or outside scope.
function next() {
return "next function method";
}
function getValues(value = next()) {}
Default parameters Destructuring Assignment example
Default parameters can also be used with the Destruction assignment. Destruction assignment is a new kind of syntax introduced in ES6 and expression to retrieve values from arrays and objects and assign them to variables.
Destruction assignment example
var value1, value2;
[value1, value2] = [30, 50]; // outputs value1=30 and value2=50
[value1 = 4, value2 = value1] = []; // value1=4;value2=4
[value1 = 5, value2 = value1] = [6]; // value1=6; value2=6
[value1 = 3, value2 = value1] = [3, 5]; // value1=3; value2=5
here is an example of destruction assignment as a default parameter in a function declaration
function func([a, b] = [5, 6], { c: c } = { c: 2 }) {
return a + b + c;
}
console.log(func()); // outputs 13
Destructured Object assignment example
Default parameters declared with Object destructuring assignment. The following is an example of the usage of Object destruction.
function funcObject({ name = "Frank", salary = 5000 } = {}) {
return `${name}, ${salary}`;
}
console.log(
funcObject({
name: "kiran",
}),
);
console.log(funcObject({}));
The output of the above code
kiran, 5000;
Frank, 5000;