javascript Clone Understand shallow and deep copy examples
- Admin
- Dec 31, 2023
- Javascript
Shallow and Deep Copy introduction
Sometimes, In applications, we have to write code for use cases like a duplicate copy of an array/JSON/object. This article covers a basic understanding of this and examples.
Javascript offers two types for copying an object 1. Shallow Copy 2. Deep Copy
Shallow Clone in javascript
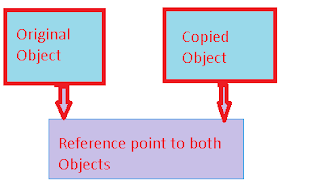
It copies a value of the original object and copies the reference of other child objects if the reference to other child objects. It copies primitives like numbers and strings and if any child object exists, The newly copied object has new values as child object references. If any child object is modified, It reflects in the original and copied object too. In this case, the memory segment is the same and the address of the memory segment is a point to a single. It performs well as only one memory usage is very less for one segment.
Let us see the Shallow copy example. It does achieve in many ways.
Simple assign
First, create an employee object with name=franc and department=Marketing
const originalEmployee = {
name: "Franc",
department: {
type: "Marketing",
},
};
Next is to duplicate this object by assigning it to a new variable.
var copiedEmployee = originalEmployee;
Modify copied object with name=Kiran and department=Development
copiedEmployee.name = "Kiran";
copiedEmployee.department.type = "Development";
console.log("original", originalEmployee); //returns {name:"Kiran", department:{type: "Development"}
console.log("copy", copiedEmployee); //returns {name:"Kiran", department:{type: "Development"}
Now original Employee object’s original values are lost. The original and copied object has pointed to modified values.
using Object.assign() method
values copy and child references are copied, but not child objects themselves. The below code modifies the original object the same as above.
const copiedEmployee = Object.assign({}, originalEmployee);
originalEmployee.name = "Kiran";
originalEmployee.department.type = "Development";
console.log(originalEmployee); //returns {name:"Kiran", department:{type: "Development"}
console.log(copiedEmployee); //returns {name:"Franc", department:{type: "Development"}
Create an original object and copy using the assign method, Now we modified the original object value as well as to object references. The copied object has not modified values still pointing to the original value and modifying object references values are still pointing to the same object by the original and copied objects
Using Es6/Es2015 Spread Operator
It is another way to do shallow copy using the latest javascript, here is an exercise for this.
const copiedEmployee = { ...originalEmployee };
originalEmployee.name = "Kiran";
originalEmployee.department.type = "Development";
console.log(originalEmployee); //returns {name:"Kiran", department:{type: "Development"}
console.log(copiedEmployee); //returns {name:"Franc", department:{type: "Development"}
The behavior is the same as the object.assign() method.
Deep Copy in javascript
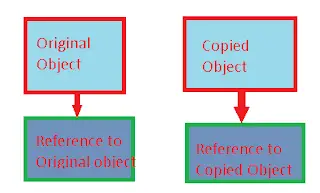
It copies the original object which creates new memory a segment and points a new object to the newly created memory segment. Here two memory segments exist one is for the original object other is for the copied object. It copies all the values and references object values too. So here two objects are different.
Example Usage
It does achieve using creating a new object variable and constructing the object as below I am giving examples for the same original data above.
var duplicateCopy = {
name: originalEmployee.name,
department: { type: originalEmployee.department.type },
};
if we change the Orignal Employee Object, the Copied object will not get modifications. Two objects are independent.
Hope you understand this. Please comment and share this on Facebook/Twitter if you have any questions.