Fix for java.lang.UnsupportedClassVersionError in Java
The UnsupportedClassVersionError
is a runtime error that occurs when Java code is compiled and run with incompatible and unsupported Java versions. UnsupportedClassVersionError
class extends ClassFormatError
, a subclass of LinkageError
.
This runtime error is thrown by the JVM when attempting to run a Java class file with major and minor versions that are not supported. The error stack trace contains a message like “Unsupported major.minor version x.x”, with x.x corresponding to the version of the installed Java.
The simple fix is to ensure that you install both JDK
and JRE
with the same version. For instance, if you installed JDK 11, use JRE 11, not JRE 8 or any other versions.
This error arises when running Java programs with an incompatible version of compiled and running programs. It usually occurs when a Java file is compiled with JDK 11 and then run using JDK 8.
To understand what caused this error, consider the following major.minor version table for different Java versions:
JDK Version | Major Number |
---|---|
1.1 | 45 |
1.2 | 46 |
1.3 | 46 |
1.4 | 47 |
1.5 | 48 |
1.6 | 49 |
1.7 | 50 |
1.8 | 51 |
1.9 | 52 |
1.10 | 53 |
1.11 | 54 |
1.12 | 55 |
1.13 | 56 |
1.14 | 57 |
1.15 | 58 |
1.16 | 59 |
1.17 | 60 |
1.18 | 61 |
1.19 | 62 |
1.20 | 63 |
1.21 | 64 |
Solution for java.lang.UnsupportedClassVersionError Error
First, check the Java and javac versions using the command line.
For example:
C:\myapp>javac -version
javac 1.8.0_102
And for the runtime version.
C:\myapp>java -version
java version "10.0.2" 2018-07-17
Java(TM) SE Runtime Environment 18.3 (build 10.0.2+13)
Java HotSpot(TM) 64-Bit Server VM 18.3 (build 10.0.2+13, mixed mode)
In this case, both have different versions.
To resolve, upgrade either JDK or JRE to the same version. Once the same version is set, recompile and run the code. This error may occur due to:
Compilation of code running a different, higher Java version.
The runtime of your code running with a lower Java version.
This error comes in eclipse as well as command line
Fix for UnsupportedClassVersionError Error in Eclipse
When you run java code, First change your project settings point to JDK, Next set the java compiler to a specific java version. Here are steps
- Right-click on your
project
—>Properties
—>Java Build Path
—Libraries
- Select Java libraries as shown below, and choose Edit and select target JRE
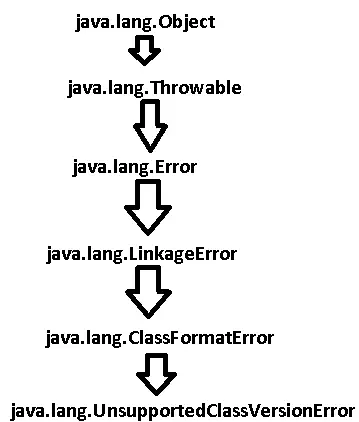
Next, set the java compiler version and target level.
Please check below screenshot to do eclipse configuration
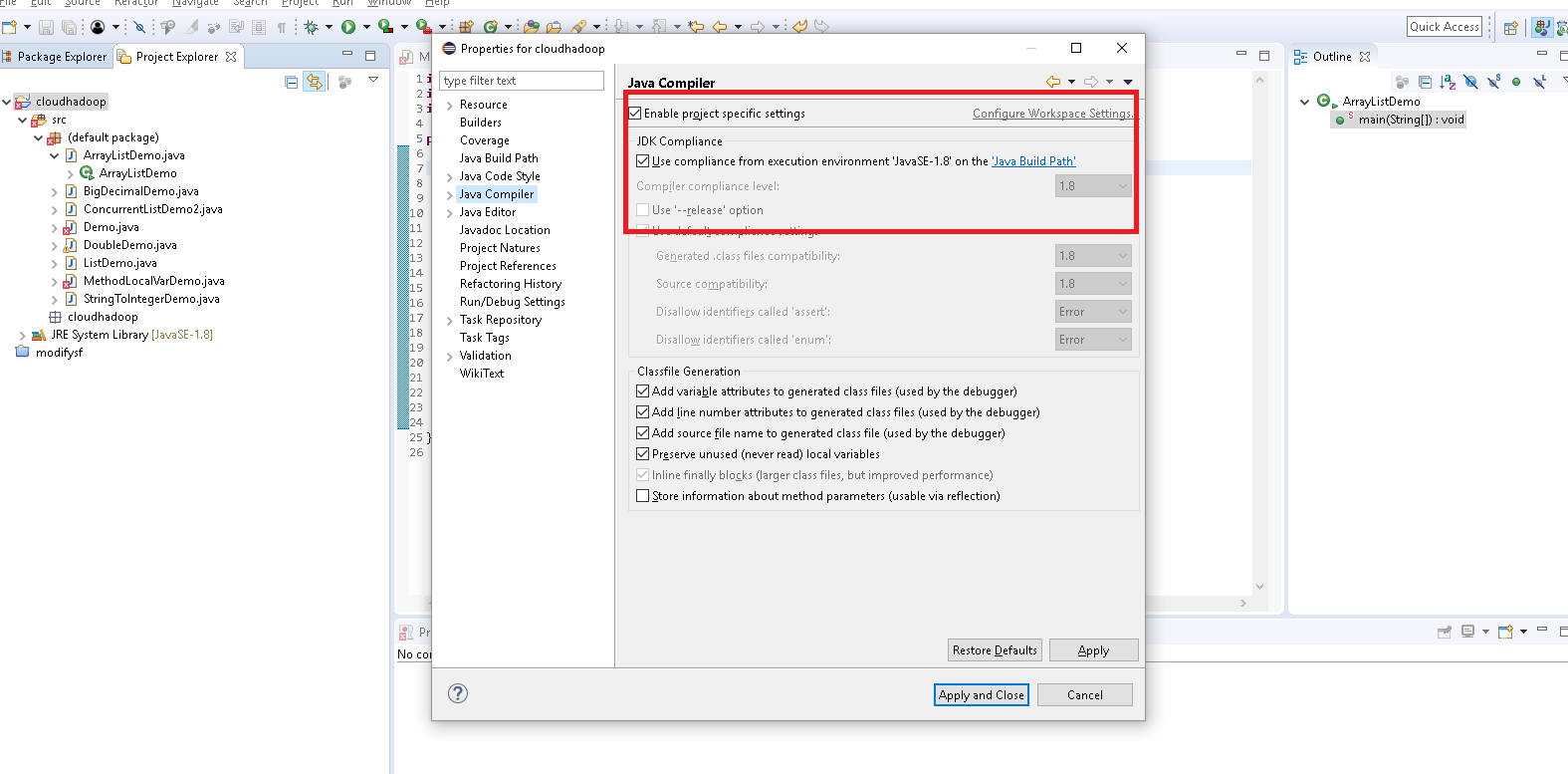
Fix for UnsupportedClassVersionError Error via Command Line
This error can also be fixed using the source
and target
command-line options. Provide source
and target
options with your version:
javac -source 1.8 -target 1.8 javafile.java
This ensures compatibility with backward versions.
Fix for UnsupportedClassVersionError Error for Maven Project
To fix this error in a Maven project, add source and target options for the Maven compiler plugin. This compiles and runs your project with the same version.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<source>1.10</source>
<target>1.10</target>
</configuration>
</plugin>
Conclusion
This post addressed the cause of UnsupportedClassVersionError and provided fixes for command line, Eclipse, and Maven projects, dealing with compile and runtime version mismatches.