Typescript tutorials Hello World demo application examples
- Admin
- Mar 6, 2024
- Typescript
Typescript is an extended version of javascript with more features like static typing, Module-based code, and Object-oriented support.
Typescript is an open-source language from Microsoft. Typescript will not run on any browser directly. The compiler is required to compile typescript to javascript.
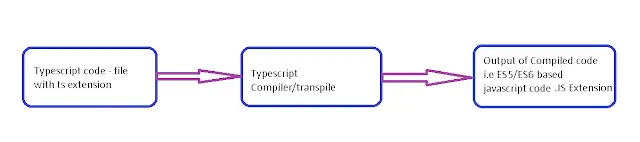
The above diagram depicts the steps for the execution code process.
- The developer writes the code using typescript language in a Normal Editor/ Compatible IDE. These files always contain .ts extension.
- To compile the files, use either a typescript compiler (tsc command) or any other build tools.
- Transpiler or compiler is processing converting code written in a programming language to another programming language.
- The compilation process generates javascript code, which is compatible with all popular browsers.
Typescript Advantages or Pros
- Supports
Static typing
, ortype inference
which means variables can be declared with the type. You can assign another different type to this variable. - Support Object-oriented concepts like interfaces and classes. This will be very easy to learn for developers who worked on Object-oriented languages like Java and C++
- Integrated Support for all popular Editors like Visual Studio Code, Eclipse, and Intelli Editors
- All the functionalities support all popular browsers.
- Code errors will be shown at compile-time and are very easy for developers to fix instead of production.
- Support NPM-based applications as well as normal applications using a typescript compiler
- Supports the Latest Javascript features like ES5 and ES6
- Supports all popular frameworks like Angular, React, and Vue.js
- Modules/components Support
- IDE supports
Typescript disadvantages or Cons
- One more language to learn. A learning curve is required.
- The compilation is required to run code in the browser
Typescript IDE/Text Editors Support
IDE is used for faster development and has a lot of advantages for Writing code, formatting code, Autosuggestion, compilation, and Debugging support. and also tells the errors while typing the code.
Typescript support is available in almost all popular Editors via either inbuilt or plugin extension
Eclipse Plugin for typescript Opensource Eclipse has very good support for typescript language. You can check eclipse javascript IDE and the commercial MyEclipse version has inbuilt support.
Visual Studio Code integrated typescript This is a popular IDE in the JavaScript community for web application development.
It has built-in support for typescript language. And also has a lot of custom plugins for different frameworks.
Atom editor plugin support: This editor has support via plugins. The atom-Typescript plugin has the latest version of typescript.
Intelli Webstorm support: Intell has a different IDE for Java and javascript projects. Both IDEs have support for this language. There are a lot of extension plugins you can add for other features.
You can also use a normal notepad or Edit Plus which you need to write manually code without the autosuggestion feature.
Installation and setup of typescript on Windows
The only prerequisite is to have a setup of the NPM command working. if you don’t have the npm command working, Please install nodejs on your setup before installation of typescript.
C:\>npm --version
5.6.0
Once the npm command is working, please follow for the installation
npm install -g typescript
C:\>tsc --version
Version 2.8.1
-g option installs the typescript global module. if you want to install it in the project remove the -g option. Linux/UNIX Installation is also the same except you have to use the Sudo command for root permission
sudo npm install -g typescript
TSC command basic syntax:
tsc [options] [file ...]
please see below examples of this command
tsc file.ts - compiles typescript file and generate file.ts
tsc --outFile output.js newfile.ts - customize the out file
Typescript compiler options
The below are different options tsc command
Options | Description |
---|---|
--allowUnreachableCode | Compiler will not give an error if any unreachable code |
--init | Create a Typescript prototype project with tsconfig.json file included |
--lib | External Libraries required for compilation of typescript code. without this option, Default libraries are used to compile it. Use this option for the Latest javascript libraries support es6 |
--allowJS | Include javascript files to compile |
--outDir | directory for output of compiled typescript files |
outFile | out file name for compiled typescript code |
Typescript Hello World Basic Example
function HelloWordFunction(information) {
console.log(information);
}
var msg = "Hello World First Typescript example";
HelloWordFunction(msg);
and see the compilation and generation of javascript using transpiler tsc command
tsc HelloWorld.ts
B:\typescriptdemo>dir
Volume in drive B has no label.
Volume Serial Number is 0A9B-F96C
Directory of B:\typescriptdemo
14-07-2018 20:14 153 HelloWorld.js
14-07-2018 20:11 152 HelloWorld.ts
2 File(s) 305 bytes
2 Dir(s) 99,749,167,104 bytes free