Running VueJS Hello World Application using parcelJs
In my previous article Learned how to install parcel and Hello world example.
In this tutorial, we will walk through the Hello World VueJs application using the Parcel module bundler. parcelJS is a web application module bunder with zero configuration and the best in performance. A lot of VueJS applications use either plain NPM commands or webpack as module bunder to run and test it
VueJS is an open-source framework for building UI applications.
Prerequisites:
Nodejs framework is needed to install it. Nodejs comes with node and npm commands.
First, create an empty project using mkdir vuejsdemo. An empty Directory was created.
The next step is to create package.json in an empty directory using the npm command
npm init-i`
package.json file created in a project. package.json file contains initial project configuration details as below
{
"name": "vueJsDemo",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
Install Vuejs Library
npm install --save vue
It installs vue dependency in the local project node_modules folder and will add a dependency in package.json.
Please see the below screenshot for more information.
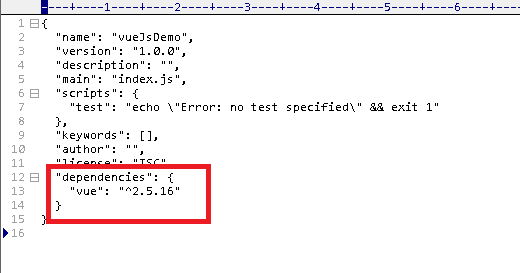
Install parceljs npm dependency
npm install --save-dev parcel-bundler
This installs parcel-bundler dependency in your project and adds it as a dev dependency in package.json. Using yarn package manager if you are using yarn command without npm, use the below commands
yarn add vue
yarn add --save-dev parcel-bundler
The next step is to create the required project files. files are index.html, App.vue, and entry javascript files Index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="Generator" content="EditPlus®" />
<meta name="Author" content="" />
<meta name="Keywords" content="" />
<meta name="Description" content="" />
<title>ParelJS Vue Hello worldApplication</title>
</head>
<body>
<div id="mainApp"></div>
<!-- Parcel will rewrite this path on the build. -->
<script src="./src/main.js"></script>
</body>
</html>
Next, create an App.vue file.
<template>
<div id="mainApp">
<h4>
{{ msg }}</h4>
</div>
</template>
<br />export default {<br /> name: 'mainApp',<br /> data () {<br /> return {<br /> msg: 'Parcel Hello World Application'<br /> }<br /> }<br />}<br />
Create an entry file Main.js which is the starting point of execution in the Vuejs application
import Vue from "vue";
import App from "./App.vue";
new Vue({
el: "#MainApp",
render: (h) => h(App),
});
The next step is to add the below entries in package.json
import Vue from 'vue';
"scripts": {
"dev": "parcel index.html",
"build": "parcel build"
}
Running Vuejs Project using ParelJS
We generated basic application files. Now we are ready to run the app using the npm run dev project with Live reloading support. The server started in localhost:1234. open localhost:1234 URL in browser npm run dev command is a for starting development server. npm run prod command is to build code for a production server. Please see the below screenshot to access the Hello World application.

Parcel EsLint tool support
the parcel has support for this tool. Please install npm install eslint and configure in the project.
Example .eslintrc
Module.exports = {
extends: [
// add more generic rulesets here, such as:
'eslint:recommended,
'plugin:vue/essential'
],
rules: {
// override/add rules settings here, such as:
// 'vue/no-unused-vars': 'error'
}
}
ParcelJS assets transform
the parcel has inbuilt support for different assets like javascript/CSS and HTML
Use babel transpiler for javascript assets, PostCSS for CSS, and postHtml for HTML changes.
To integrate this asset, Create a file .babelrc and .postcssrc and .eslintrc.js for eslint functionality
create .babelrc
, .postcssrc
, and posthtmlrc
files as below
.babelrc example
{
"presets": [
"env"
]
}
.postcssrc example
{
"modules": true,
"plugins": {
"autoprefixer": {
"grid": true
}
}
}
.posthtmlrc example
{
"plugins": {
"posthtml-img-autosize": {
"root": "./images"
}
}
}
Typescript support for vuejs using parcel
Please install typescript using the npm install -g typescript command, next step is to create a typescript file and integrate it.