Junit: java unit testing framework with examples
java unit testing or JUnit
Junit is a popular Java unit testing framework. Junit is developed to unit test the piece of code.
What do you mean by unit testing? Unit testing is a test code written for checking the runtime errors for each function/line of a java code. Junit can be used to regress each small change so that large applications should not give errors even adding small changes.
Testing is a piece of code written for checking the validity of actual business logic code like a null check and comparing actual and expected values.
Unit testing is a developer activity as part of the software quality assurance process.
JUnit can be coded in to test the correctness of executions paths of java unit code JUnit tests are coded by developers as part of the software development life cycle. Java unit testing can also be called white box testing. Whereas black box testing means functionally of the system done by QA/testers. After the analysis and design phase, before writing code development, normal practice is to write the unit test code for the new functionally, then code new functionally, this way we find all the issues for the new functionally.
Junit is an open-source testing framework and the current version is 4. x. There are two types of test cases in JUnit. One is a single test case, other are multiple test cases called Test suit
Junit can be shipped as a jar file. You can download JUnit 4. x jar from the Junit site. Junit test can be executed in multiple following ways.
1 junit with maven plugin: -
You need to define the JUnit dependency for your project where you are going to write unit test code. Here we will specify artifact name, groupId, and version.
2. junit with ant task
Ant is provided a different task to run the test case by configuring the task in build.xml JUnit plugin in eclipse:- JUnit plugin should be installed to eclipse. Eclipse Galileo has the default JUnit plugin installed.
3. junit eclipse:-
Junit examples
`package org.cloudhadoop;
public class Calc {
public int add(int a, int b) {
return a + b;
}
}``
package org.cloudhadoop;
import JUnit.framework.Assert;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Before;
import org.junit.Test;
public class CalcTest {
Calc calc = null;
@Before
public void setup() {
calc = new Calc();
}
@Test
public void testAdd() {
int a = 10;
int b = 20;
int actual = 30;
Assert.assertNotNull(calc);
int expected = c.add(a, b);
Assert.assertEquals(actual, expected);
}
@Test
public void testAddErorcase() {
int a = 10;
int b = 20;
int actual = 30;
Calc c = new Calc();
Assert.assertNotNull(c);
int expected = c.add(a, b);
Assert.assertEquals(actual, expected);
}
@After
public void tearDown() {
}
}
`
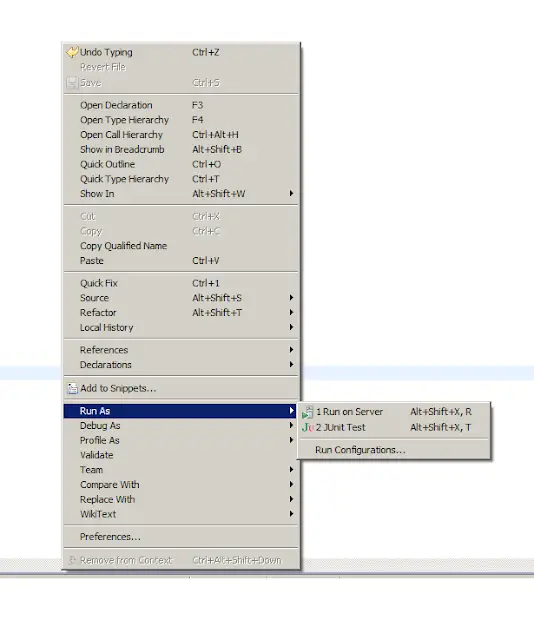
Java unit testing rules:-
All test class names end with XXXXTest, for example, the class is Calculator, the Test class should be CalculatorTest All test methods start with tesMethod and annotated the method as @Test